在 JavaScript 中上傳檔案的例子
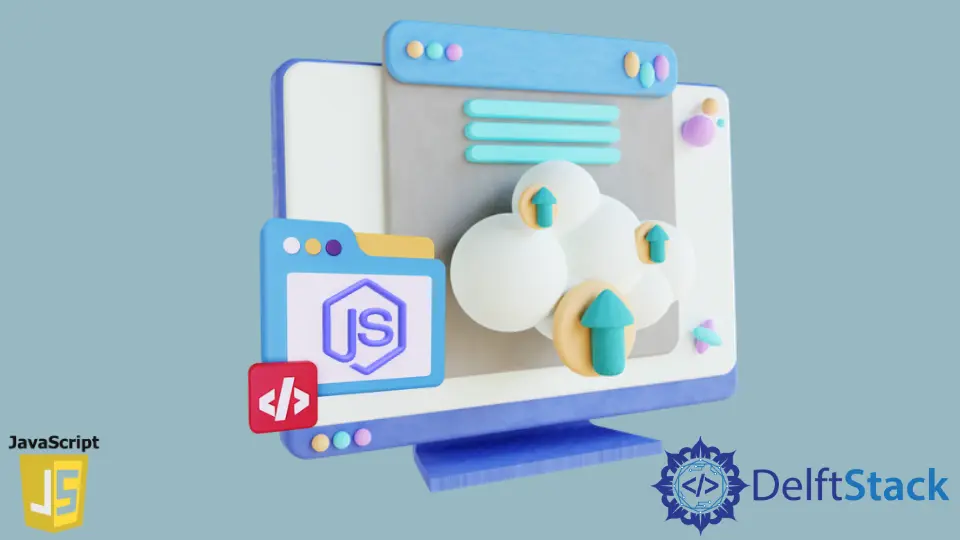
本文解釋了我們如何從檔案選擇器對話方塊中選擇一個檔案,並使用 fetch
方法將所選檔案上傳到我們的伺服器。我們需要將我們選擇的檔案資料附加到表單資料物件中,以將任何檔案上傳到我們的後端伺服器儲存中。
let formData = new FormData();
formData.append('file', fileupload.files[0]);
在 HTML 中使用 JavaScript 上傳檔案示例
我們將製作一個檔案
型別的輸入表單,以從我們的 pc/系統儲存中選擇任何副檔名的檔案。將有一個按鈕來呼叫函式 uploadFile()
,該函式會將檔案資料附加到表單資料物件中,並使用 fetch 方法簡單地將表單資料上傳到我們的伺服器。fetch
方法在 JavaScript 中用於網路請求,作為 API 呼叫,用於從前端獲取或上傳任何資料到後端。
<!DOCTYPE html>
<html>
<head>
<title>
HTML | File upload example
</title>
<script type="text/javascript">
</script>
</head>
<body>
<h2>Hi Users Choose your file and Click upload.</h2>
<!-- Input Form Elements HTML 5 -->
<input id="fileupload" type="file" name="fileupload" />
<button id="upload-button" onclick="uploadFile()"> Upload file </button>
<!-- File Upload Logic JavaScript -->
<script>
async function uploadFile() {
//creating form data object and append file into that form data
let formData = new FormData();
formData.append("file", fileupload.files[0]);
//network request using POST method of fetch
await fetch('PASTE_YOUR_URL_HERE', {
method: "POST",
body: formData
});
alert('You have successfully upload the file!');
}
</script>
</body>
<html>
在上面的 HTML
頁面原始碼中,你可以看到檔案
的簡單輸入表單型別,通過單擊選擇檔案
從使用者本地系統儲存中獲取檔案資料,並且有一個上傳檔案
按鈕來呼叫我們的宣告函式並繼續執行該功能。
在此前端網頁上,你還可以看到帶有所選副檔名的名稱。
在這裡,你可以看到 <script>
標籤,這是在 doctype html 中使用 javaScript
語句所必需的。在這些標籤中,我們宣告瞭 uploadFile()
。
該函式包含一個表單資料物件,檔案將附加到該表單資料物件中,並使用 fetch POST
方法呼叫網路請求;檔案資料將以網路請求的形式傳送。
在下一步中,如果檔案上傳成功的話,function()
向使用者顯示一個警告框 You have successfully upload the file!
。
使用 JavaScript 上傳/選擇檔案的替代方法
你也可以達到如下所示的相同結果。你可以使用 document.createElement() 方法
建立一個帶有 type="file"
的 <input>
元素。
我們可以使用 getElementById()
方法簡單地訪問帶有 type="file"
的 <input>
元素。
var data = document.getElementById('my_file');
<button onclick="myFunction()">Choose file</button>
<script>
function myTestFunction() {
var data = document.createElement("INPUT");
data.setAttribute("type", "file");
document.body.appendChild(data);
}
</script>
在上面的例子中,var data
分配了 document.createElement("INPUT");
函式,我們需要設定檔案型別的資料屬性。
在下一步中,我們需要在 document.body
上新增 appendChild
資料。