JavaScript 字串 startsWith
-
在 JavaScript 中使用字串
startsWith()
方法 -
在 JavaScript 中使用
indexOf()
函式來檢查字串是否以另一個字串開頭 -
在 JavaScript 中使用
lastIndexOf()
函式檢查字串是否以另一個字串開頭 -
在 JavaScript 中使用
substring()
函式檢查字串是否以另一個字串開頭 -
使用 Regex 的
test()
方法檢查字串是否以 JavaScript 中的另一個字串開頭 - 在 JavaScript 中使用帶有迴圈的自定義函式來檢查字串是否以另一個字串開頭
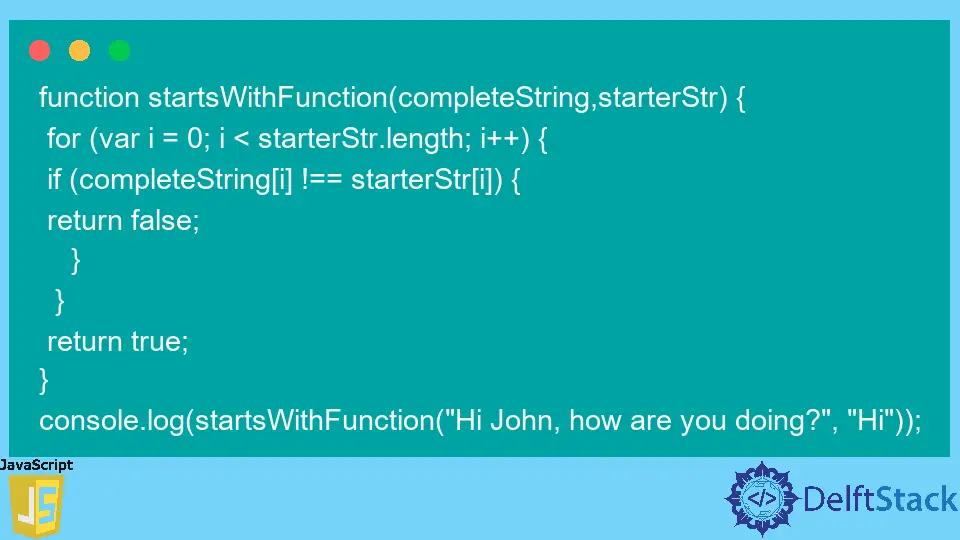
當我們想要確保字串是否以特定字串開頭時,在編碼時會有一些情況。
例如,我想檢查給定的字串是否以 Me
字串開頭。
今天,我們將學習如何使用 startsWith()
、indexOf()
、lastIndexOf()
、substring()
函式以及正規表示式的 test()
方法來檢查字串是否以特定字串開頭和一個帶迴圈的自定義函式。
在 JavaScript 中使用字串 startsWith()
方法
ECMAScript 2015(也稱為 ES6)引入了 startsWith()
方法,該方法檢查字串是否以指定字串開頭。如果找到匹配,則輸出 true
;否則,假
。
startsWith()
方法有兩個引數:searchString
,第二個是 position
,它是可選的,預設為零。如果指定了位置,則函式從該位置搜尋字串。
在下面的例子中,我們沒有指定 position
。因此,它從索引零開始區分大小寫搜尋。
var name = 'Hi John, how are you doing?'
console.log(name.startsWith('Hi John'));
輸出:
true
示例程式碼:
var name = 'Hi John, how are you doing?'
console.log(name.startsWith('Hi john'));
輸出:
false
示例程式碼:
var name = 'Hi John, how are you doing?'
console.log(name.startsWith(
'how', 9)); // it starts searching and matching from index 9
輸出:
true
在 JavaScript 中使用 indexOf()
函式來檢查字串是否以另一個字串開頭
indexOf()
與陣列一起使用來定位元素並返回找到它的第一個索引,但我們也可以通過以下方式使用字串。
如果匹配元素的索引為零,則表示字串以指定字串開頭。
示例程式碼:
var name = 'Hi John, how are you doing?'
if (name.indexOf('Hi') === 0)
console.log(true);
else console.log(false);
輸出:
true
它還區分大小寫搜尋。看看下面的示例程式碼。
var name = 'Hi John, how are you doing?'
if (name.indexOf('hi') === 0)
console.log(true);
else console.log(false);
輸出:
false
如果我們想讓 indexOf()
從指定位置定位元素怎麼辦?
var name = 'Hi John, how are you doing?'
if (name.indexOf('how') === 9)
console.log(true);
else console.log(false);
輸出:
true
在 JavaScript 中使用 lastIndexOf()
函式檢查字串是否以另一個字串開頭
此函式返回字串中特定值的最後一次出現的位置/索引。
它從末尾開始搜尋並移向字串的開頭。如果值不存在,則返回 -1
。
我們可以稍微修改一下,通過下面的方式使用 lastIndexOf()
方法來檢視字串是否以另一個字串開頭。
示例程式碼:
var name = 'Hi John, how are you doing?'
if (name.lastIndexOf('Hi', 0) === 0)
console.log(true);
else console.log(false);
輸出:
true
雖然,它從頭開始搜尋。但是我們在索引 0 處指定它的結尾。
請記住,對於此功能,hi
和 Hi
是不同的。
你可以這裡詳細瞭解它。
在 JavaScript 中使用 substring()
函式檢查字串是否以另一個字串開頭
除了指定另一個字串來檢查字串是否以它開頭,我們還可以根據字串的長度輸入索引範圍。
例如,我們要檢查字串是否以 Hi
開頭,因此索引如下。
var name = 'Hi John, how are you doing?'
// here the end index is not included means the end index
// would be endIndex-1
console.log(name.substring(0, 2));
輸出:
Hi
如果我們尋找 Hi John
,我們可以修改它,如下所示。
var name = 'Hi John, how are you doing?'
console.log(name.substring(0, 7));
輸出:
Hi John
如果你要在中間某處尋找特定的字串部分,則使用此函式不是一個好的選擇。原因是你可能在給定索引上找不到預期的字串。
使用 Regex 的 test()
方法檢查字串是否以 JavaScript 中的另一個字串開頭
var name = 'Hi John, how are you doing?'
console.log(/^Hi John/.test(name));
輸出:
true
它也區分大小寫。請參閱以下示例。
var name = 'Hi John, how are you doing?'
console.log(/^Hi john/.test(name));
輸出:
false
你還可以使用具有不同引數和屬性的 RegExp
物件的方法。你可以在此處詳細瞭解它。
你是否考慮過使用自定義函式來完成此功能?讓我們看一下以下部分。
在 JavaScript 中使用帶有迴圈的自定義函式來檢查字串是否以另一個字串開頭
示例程式碼:
function startsWithFunction(completeString, starterStr) {
for (var i = 0; i < starterStr.length; i++) {
if (completeString[i] !== starterStr[i]) {
return false;
}
}
return true;
}
console.log(startsWithFunction('Hi John, how are you doing?', 'Hi'));
輸出:
true
startsWithFunction()
採用主字串 Hi John, how are you doing?
和另一個字串 Hi
,以檢查主字串是否以它開頭。
此函式匹配每個字元並在成功時返回 true
;否則返回 false
。