JavaScript 按 ID 刪除元素
Harshit Jindal
2023年1月30日
JavaScript
JavaScript DOM
-
在 JavaScript 中將
outerHTML
屬性設定為空字串以按 ID 刪除元素 -
在 JavaScript 中使用
removeChild()
按 ID 刪除元素 -
在 JavaScript 中使用
remove()
按 ID 刪除元素
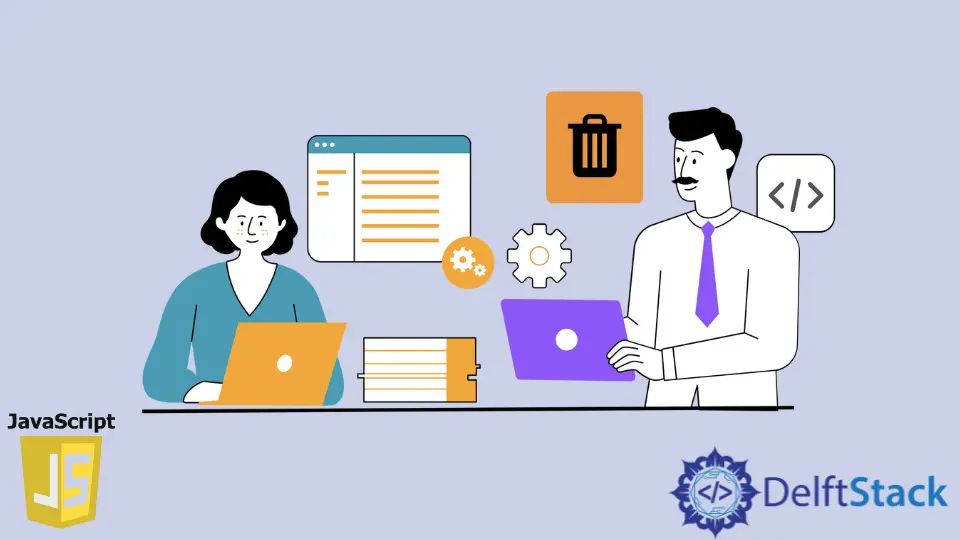
本教程介紹如何使用 JavaScript 中的 id 刪除 HTML 元素。
在 JavaScript 中將 outerHTML
屬性設定為空字串以按 ID 刪除元素
在這個方法中,我們通過分配一個空字串來選擇元素並刪除 HTML 內容。
<!DOCTYPE html>
<html lang="en">
<head>
<title>Document</title>
</head>
<body>
<h1 id="app">Hello World!!</h1>
<p id="removeme">Remove Me</p>
</body>
<script>
document.getElementById("removeme").outerHTML = "";
</script>
</html>
這是一種簡單的方法,但由於以下限制而很少使用:
- 它的速度比
removeChild()
和其他較新的 ES5 方法如remove()
慢 5-10%。 - 舊版本的 Internet Explorer 不支援。
在 JavaScript 中使用 removeChild()
按 ID 刪除元素
我們首先使用其 id 選擇元素,然後在此方法中呼叫其父級的 removeChild()
函式。這種方法提供了維護 DOM 樹結構的優點。
它迫使我們模仿從樹中刪除元素的內部行為,這樣我們就不會刪除父節點本身。
<!DOCTYPE html>
<html lang="en">
<head>
<title>Document</title>
</head>
<body>
<h1 id="app">Hello World!!</h1>
<p id="removeme">Remove Me</p>
</body>
<script>
function remove(id)
{
var element = document.getElementById(id);
return element.parentNode.removeChild(element);
}
remove("removeme");
</script>
</html>
在 JavaScript 中使用 remove()
按 ID 刪除元素
remove()
方法是作為 ES5 的一部分引入的。它允許我們直接刪除元素而不去其父元素。但與 removeChild()
方法不同,它不返回對已刪除節點的引用。
<!DOCTYPE html>
<html lang="en">
<head>
<title>Document</title>
</head>
<body>
<h1 id="app">Hello World!!</h1>
<p id="removeme">Remove Me</p>
</body>
<script>
document.getElementById("removeme").remove();
</script>
</html>
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Harshit Jindal
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn