使用 JavaScript 將此關鍵字傳遞給函式
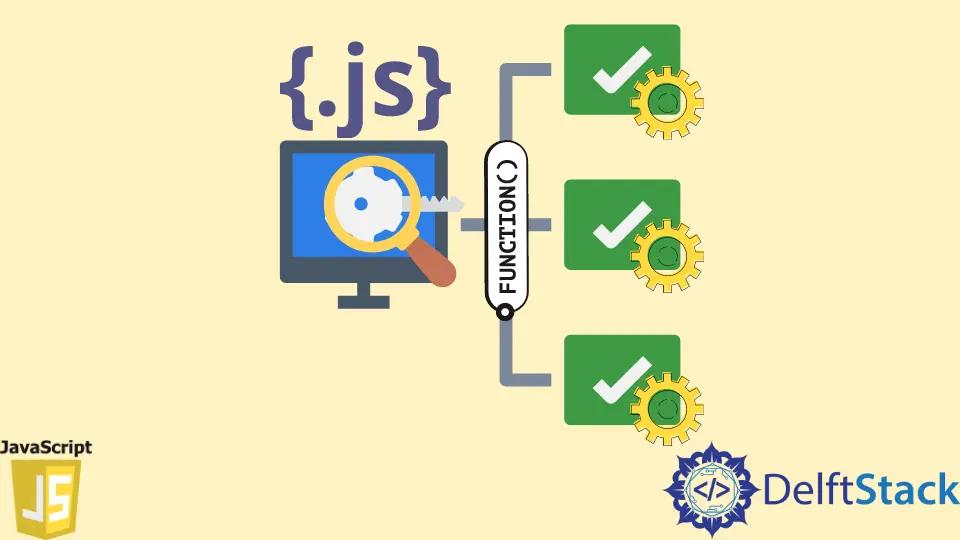
this
關鍵字是 JavaScript 中的一個關鍵概念。在 JavaScript 中,this
是物件的控制代碼。
與此相關的物件可以根據它是公共的、實體上的還是建構函式中的而直觀地改變。它也可以根據函式概念過程 bind
、call
和 apply
的數量明顯變化。
在本教程中,你將發現 this
關鍵字與依賴上下文直觀相關的內容,你將發現如何使用 bind
、call
和 apply
方法來識別 this
的值直接地。
在 JavaScript 中使用 call()
和 apply()
方法
call()
和 apply()
都做同樣的事情:它們呼叫一個給定上下文和可選引數的函式。這兩種方法的唯一區別是 call()
接受一個接一個的引數,而 apply()
接受一個引數陣列。
在這個例子中,我們將建立一個物件和一個引用它但沒有任何 this
上下文的函式。
例子:
const fruit = {
name: 'Apple',
location: 'Nepal',
} function summ() {
console.log(`${this.name} is found in ${this.location}.`)
} summ()
輸出:
"result is found in https://fiddle.jshell.net/_display/?editor_console=true."
因為 summ
和 fruit
是不相關的,所以當全域性物件搜尋這些屬性時,單獨執行 summ
將是未定義的。但是,你可以使用 call 和 apply 在方法上呼叫 this
水果上下文。
const fruit =
{
name: 'Apple',
location: 'Nepal',
}
function summ() {
console.log(`${this.name} is found in ${this.location}.`)
}
summ.call(fruit)
const fruit =
{
name: 'Apple',
location: 'Nepal',
}
function summ() {
console.log(`${this.name} is found in ${this.location}.`)
}
summ.apply(fruit)
輸出:
"Apple is found in Nepal."
現在,當使用這些策略時,fruit
和 summ
之間存在聯絡。讓我們確保我們在同一個頁面上並且知道 this
是什麼。
function printhis() {
console.log(this)
}
printhis.call(fruit)
function printhis() {
console.log(this)
}
printhis.apply(fruit)
輸出:
{
location: "Nepal",
name: "Apple"
}
this
是在這種情況下作為引數提供的物件。call()
和 apply()
在這方面是相似的,但有一個細微的區別。
除了 this
上下文之外,你還可以選擇傳遞額外的引數作為第一個引數。使用 call()
方法,你要傳遞的每個附加值都作為單獨的引數提交。
const fruit =
{
name: 'Apple',
location: 'Nepal',
}
function longerSumm(family, year) {
console.log(`${this.name} is found in ${this.location}. It is from ${
family} family found in ${year}.`)
}
longerSumm.call(fruit, 'malius', 1900)
輸出:
"Apple is found in Nepal. It is from malius family found in 1900."
如果你使用 apply()
傳送相同的引數,則會發生這種情況。
const fruit =
{
name: 'Apple',
location: 'Nepal',
}
function longerSumm(family, year) {
console.log(`${this.name} is found in ${this.location}. It is from ${
family} family found in ${year}.`)
}
longerSumm.apply(fruit, 'malius', 1932)
輸出:
Uncaught TypeError: CreateListFromArrayLike called on non-object
相反,你必須將陣列中的所有引數傳遞給 apply()
。
const fruit =
{
name: 'Apple',
location: 'Nepal',
}
function longerSumm(family, year) {
console.log(`${this.name} is found in ${this.location}. It is from ${
family} family found in ${year}.`)
}
longerSumm.apply(fruit, ['malius', 1900])
輸出:
"Apple is found in Nepal. It is from malius family found in 1900."
單獨傳遞引數和在陣列中傳遞引數之間的差異很小。使用 apply()
可能更容易,因為如果任何引數細節發生變化,它不需要修改函式呼叫。
在 JavaScript 中使用 bind()
方法
你可能需要重複調用具有另一個物件的 this
上下文的方法,在這種情況下,你可以使用 bind()
方法來構造一個帶有有意連結的 this
的新函式。
const fruit = {
name: 'Apple',
location: 'Nepal',
} function summ() {
console.log(`${this.name} is found in ${this.location}.`)
} const newSummary = summ.bind(fruit)
newSummary()
輸出:
"Apple is found in Nepal."
當你在本例中呼叫 newSummary
時,它將始終返回與其相關的原始值。如果你嘗試將新的 this
上下文繫結到它,它將失敗。
因此,你始終可以依靠連結函式來提供你期望的 this
值。
const fruit = {
name: 'Apple',
location: 'Nepal',
} function summ() {
console.log(`${this.name} is found in ${this.location}.`)
} const newSummary = summ.bind(fruit)
newSummary()
const fruit2 = {
name: 'Mango',
location: 'Terai',
}
newSummary.bind(fruit2)
newSummary()
輸出:
"Apple is found in Nepal."
即使此示例嘗試第二次繫結 newSummary
,它仍保留第一次繫結時的原始上下文。
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn