在 Go 中使用分隔符拆分字串
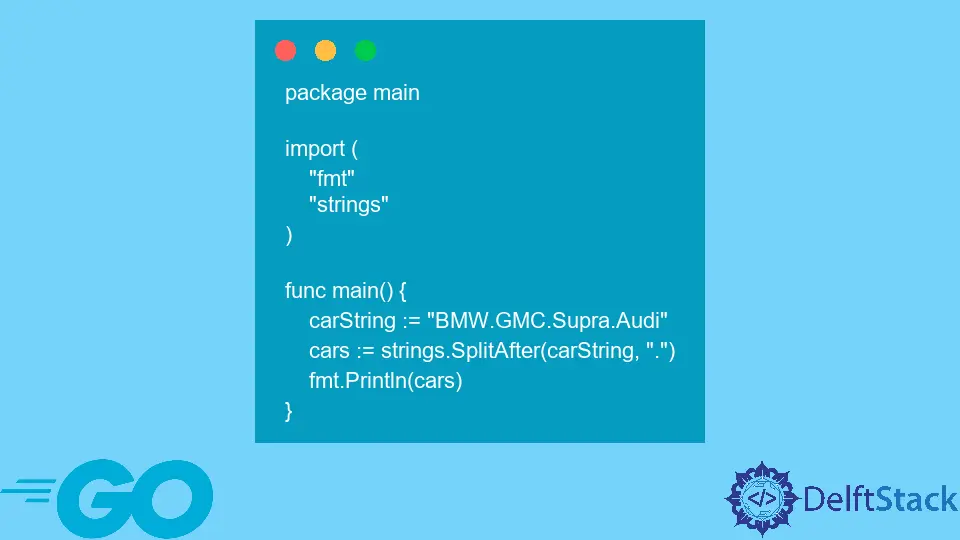
本文將提供在 Go 中分割字串的方法。
使用 Go 中的 Split()
方法使用分隔符拆分字串
在 Go 中,Split()
函式(包含在 strings 包中)使用分隔符將字串劃分為子字串列表。子字串以切片的形式返回。
在以下示例中,我們將使用以逗號分隔的字串作為分隔符。
示例 1:
package main
import (
"fmt"
"strings"
)
func main() {
var str = "a-b-c"
var delimiter = "-"
var parts = strings.Split(str, delimiter)
fmt.Println(parts)
}
輸出:
[a b c]
示例 2:
package main
import (
"fmt"
"strings"
)
func main() {
str := "hi, there!, Good morning"
split := strings.Split(str, ",")
fmt.Println(split)
fmt.Println("Length of the slice:", len(split))
}
輸出:
[hi there! Good morning]
Length of the slice: 3
示例 3:
package main
import (
"fmt"
"strings"
)
func main() {
carString := "BMW,GMC,Supra,Audi"
cars := strings.Split(carString, ",")
fmt.Println(cars)
}
輸出:
[BMW GMC Supra Audi]
在 Go 中使用 SplitAfter()
方法拆分帶有分隔符的字串
SplitAfter()
分隔原始文字,但將分隔符留在每個子字串的末尾,類似於 Split()
。
package main
import (
"fmt"
"strings"
)
func main() {
carString := "BMW.GMC.Supra.Audi"
cars := strings.SplitAfter(carString, ".")
fmt.Println(cars)
}
輸出:
[BMW. GMC. Supra. Audi]
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe