從 C# 中的函式返回多個值
- 使用 C# 中的陣列從函式中返回多個值
- 使用 C# 中的帶有結構體/類物件的函式返回多個值
-
在 C# 中使用
Tuples<T1,T2>
類從函式返回多個值 -
在 C# 中使用
(T1,T2)
表示法從函式中返回多個值
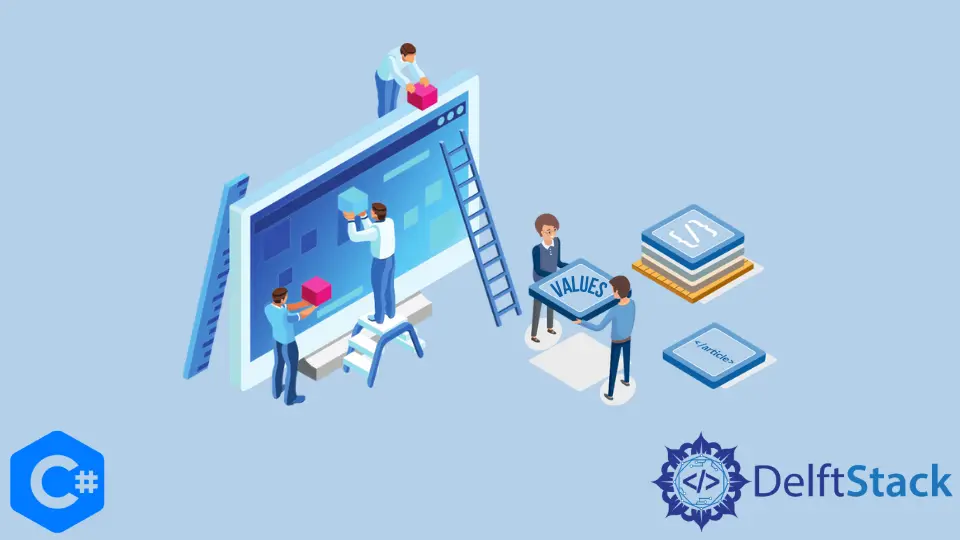
本教程將介紹從 C# 函式中返回多個值的方法。
使用 C# 中的陣列從函式中返回多個值
陣列資料結構體在 C# 中儲存相同資料型別的多個值。我們可以使用陣列從一個函式返回多個值。以下程式碼示例向我們展示瞭如何使用陣列從 C# 中的函式返回多個值。
using System;
namespace return_multipe_values {
class Program {
public static int[] compare(int a, int b) {
int[] arr = new int[2];
if (a > b) {
arr[0] = b;
arr[1] = a;
} else {
arr[0] = a;
arr[1] = b;
}
return arr;
}
static void Main(string[] args) {
int[] returnvalue = Program.compare(10, 12);
Console.WriteLine("Smaller Value = {0}", returnvalue[0]);
Console.WriteLine("Larger Value = {0}", returnvalue[1]);
}
}
}
輸出:
Smaller Value = 10
Larger Value = 12
我們用 C# 中的陣列返回型別從 compare()
函式中返回了 2 個整數值。如果必須返回相同資料型別的多個值,則此方法很有用。如果要返回不同資料型別的多個值,則不能使用陣列,因為陣列僅儲存相同資料型別的值。
使用 C# 中的帶有結構體/類物件的函式返回多個值
結構體和類用於對相關值進行分組。這些值不必具有相同的資料型別。因此,我們可以使用結構體或類物件從 C# 中的函式返回多個值。以下程式碼示例向我們展示瞭如何使用結構體物件從 C# 中的函式返回多個值。
using System;
namespace return_multipe_values {
struct Values {
public int x;
public int y;
}
class Program {
public static Values comparevalues(int a, int b) {
Values V = new Values();
if (a > b) {
V.x = b;
V.y = a;
} else {
V.x = a;
V.y = b;
}
return V;
}
static void Main(string[] args) {
Values returnedvalues = Program.comparevalues(10, 12);
Console.WriteLine("Smaller Value = {0}", returnedvalues.x);
Console.WriteLine("Larger Value = {0}", returnedvalues.y);
}
}
}
輸出:
Smaller Value = 10
Larger Value = 12
我們首先定義一個結構體 Values
,其中包含我們要從函式中返回的值。然後,我們從 comparevalues()
函式以 Values
結構體返回了多個值,作為 C# 中的返回型別。返回的值還必須儲存到相同結構體的例項中。
通過使用類作為函式的返回型別,也可以實現此目標。以下程式碼向我們展示瞭如何使用類從 C# 中的函式返回多個值。
using System;
public class Sample {
int x;
int y;
public void setxy(int v, int u) {
x = v;
y = u;
}
public void getxy() {
Console.WriteLine("The smaller value = {0}", x);
Console.WriteLine("The greater value = {0}", y);
}
}
public class Program {
public static Sample compare(int a, int b) {
Sample s = new Sample();
if (a > b) {
s.setxy(b, a);
} else {
s.setxy(a, b);
}
return s;
}
public static void Main() {
Sample returnedobj = Program.compare(10, 12);
returnedobj.getxy();
}
}
輸出:
The smaller value = 10
The greater value = 12
我們首先定義一個樣本
類,其中包含要從函式中返回的值。然後,我們從 compare()
函式返回多個值,並以 Sample
類作為 C# 中函式的返回型別。返回的值還必須儲存到同一類的例項中。儘管這兩種方法都非常適合從函式返回不同資料型別的多個值,但是編寫整個類或結構體以僅從函式返回多個值非常繁瑣且耗時。
在 C# 中使用 Tuples<T1,T2>
類從函式返回多個值
元組是在 C# 中具有特定編號和特定值順序的資料結構。元組可以具有不同資料型別的多個值。Tuple<T1,T2>
類可用於定義自定義元組具有特定順序的使用者定義資料型別。以下程式碼示例向我們展示瞭如何使用 C# 中的 Tuple<T1,T2>
類從函式返回多個值。
using System;
public class Program {
public static Tuple<string, string, int> ReturnMultipleValues() {
var result = Tuple.Create<string, string, int>("value1", "value2", 3);
return result;
}
public static void Main() {
var returnedvalues = ReturnMultipleValues();
Console.WriteLine(returnedvalues);
}
}
輸出:
(value1, value2, 3)
通過用 Tuple<string, string, int>
指定函式的返回型別,我們從 ReturnMultipleValues()
函式返回多個值。這意味著 ReturnMultipleValues()
函式將返回 3 個值,其中 string
資料型別的前兩個值和 int
資料型別的最後一個值。此返回值可以儲存到結構體相似的元組中,也可以儲存為 var
型別的變數。
在 C# 中使用 (T1,T2)
表示法從函式中返回多個值
上面討論瞭如何使用 Tuple<T1,T2>
類簡化從函式返回多個值的過程,可以通過宣告元組的 (T1, T2)
標記進一步縮短。(T1, T2)
表示法也通過縮短程式碼來實現與 Tuple<T1,T2>
類相同的功能。下面的編碼示例向我們展示瞭如何使用 (T1, T2)
表示法從 C# 中的函式返回多個值。
using System;
public class Program {
public static (string, string, int) ReturnMultipleValues() {
return ("value1", "value2", 3);
;
}
public static void Main() {
(string val1, string val2, int val3) = ReturnMultipleValues();
Console.WriteLine("{0} {1} {2}", val1, val2, val3);
}
}
輸出:
value1 value2 3
在上面的程式碼中,我們以 C# 中的 (T1,T2)
表示法從 ReturnMultipleValues()
函式返回了多個值。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn