在 C# 中按字串拆分字串
Harshit Jindal
2023年10月12日
Csharp
Csharp String
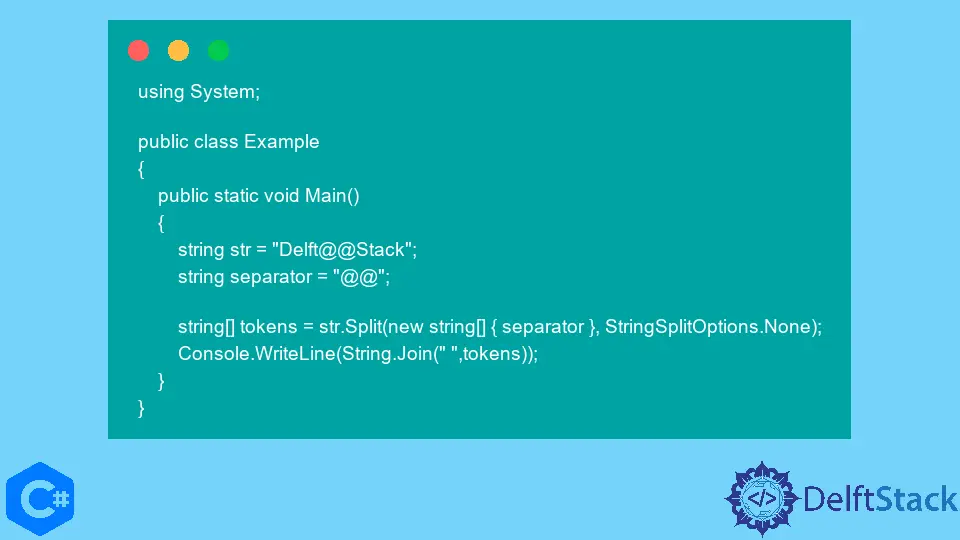
Strings
是用於儲存文字資料的物件。C# System.String
庫提供了許多用於操作、建立和比較字串的方法。
我們經常遇到的一種常見情況是拆分字串
以提取一些重要資料。本文將重點介紹使用另一個 string
拆分 string
以及在 C# 中執行此操作的不同方法。
在 C#
中使用 String.Split()
方法
String.Split()
方法具有不同的過載,為我們提供了拆分 string
的不同方法。
我們對一個特殊的過載感興趣,它接受一個 string
作為引數,並使用它作為分隔符將給定的 string
拆分為其子字串。
using System;
public class Example {
public static void Main() {
string str = "Delft@@Stack";
string separator = "@@";
string[] tokens = str.Split(new string[] { separator }, StringSplitOptions.None);
Console.WriteLine(String.Join(" ", tokens));
}
}
輸出:
Delft Stack
在上述方法中,我們使用分隔符字串@@
將給定字串拆分為子字串陣列,然後通過將它們與空格連線來列印。
在 C#
中使用 Regex.Split()
方法
Regex.Split()
方法可以實現我們想要實現的目標。它獲取輸入字串並根據正規表示式條件匹配將其拆分為子字串陣列。
using System;
using System.Text.RegularExpressions;
public class Program {
public static void Main() {
string str = "Delft@@Stack";
string separator = "@@";
string[] tokens = Regex.Split(str, separator);
Console.WriteLine(String.Join(" ", tokens));
}
}
輸出:
Delft Stack
從上面的程式碼示例中可以看出,Regex.Split()
方法的用法比 String.Split()
方法更簡單。它的執行速度更快,效率更高。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Harshit Jindal
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn