在 C# 中從字串中刪除 HTML 標記
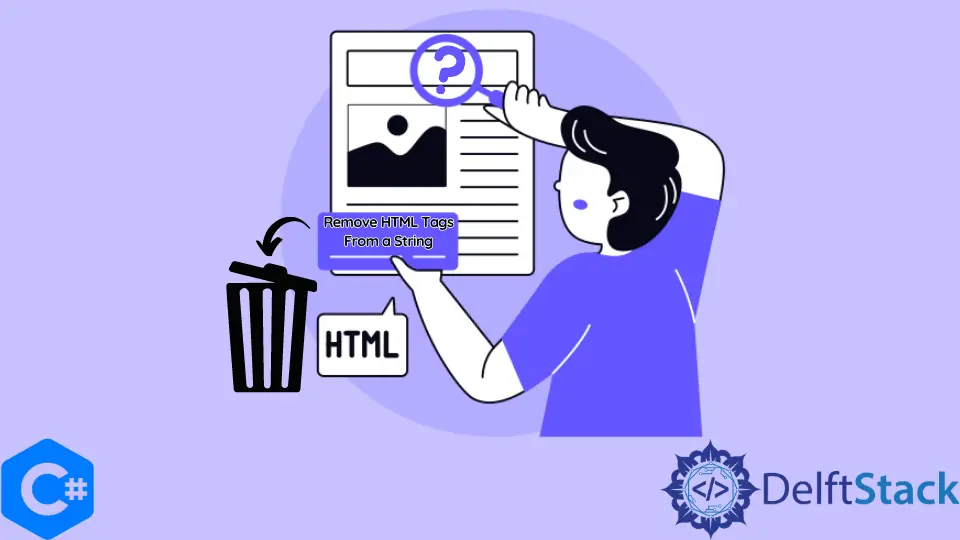
在這篇文章中,我們將演示如何在不知道其中包含哪些標籤的情況下從字串中刪除所有 HTML 標籤。
有很多方法可以完成這項任務,但沒有一種方法可以保證你刪除所有標籤。我們將看看它的一些方法。
在 C#
中使用正規表示式從字串中刪除 HTML 標籤
public static string StripHTML(string input) {
return Regex.Replace(input, "<[a-zA-Z/].*?>", String.Empty);
}
這個函式傳遞一個字串引數,我們使用 regex
的 Replace()
函式來刪除標籤,因為標籤的簽名在函式輸入中給出。
它不適用於所有情況,但大多數情況都很好。你將需要編寫演算法以從字串輸入中刪除所有標籤。
在 C#
中使用 HTML Agility Pack 從字串中刪除 HTML 標籤
另一種解決方案是使用 HTML Agility Pack。
internal static string RmvTags(string d) {
if (string.IsNullOrEmpty(d))
return string.Empty;
var doc = new HtmlDocument();
doc.LoadHtml(d);
var accTags = new String[] { "strong", "em", "u" };
var n = new Queue<HtmlNode>(doc.DocumentNode.SelectNodes("./*|./text()"));
while (n.Count > 0) {
var no = nodes.Dequeue();
var pNo = no.ParentNode;
if (!accTags.Contains(no.Name) && no.Name != "#text") {
var cNo = no.SelectNodes("./*|./text()");
if (cNo != null) {
foreach (var c in cNo) {
n.Enqueue(c);
pNo.InsertBefore(c, no);
}
}
pNo.RemoveChild(no);
}
}
return doc.DocumentNode.InnerHtml;
}
除了 strong
、em
、u
和原始文字節點之外,這將正常工作。此函式將字串作為 d
變數中的引數。
if(string.IsNullOrEmpty(d))
行檢查字串是否已經為空,然後返回空字串。
var doc = new HtmlDocument();
doc.LoadHtml(d);
這些語句建立一個新的 HTML 文件並將資料載入到文件中。它已經是一個 HTML 標記字串,並且將遵循 HTML 模式。
var accTags = new String[] { "strong", "em", "u"};
行告訴哪些標籤是允許的。你可以根據需要更改、新增或刪除標籤。
然後在 while
迴圈中,它使用佇列新增所有文件節點,使每個節點出列,並刪除 HTML 標記。
該過程繼續進行,直到所有資料都被淨化,然後它返回 HTML 文件的內部 HTML,它已經是淨化的文字。
如前所述,沒有硬性規定或方法來完成這項任務。有多種方法,沒有一種方法是完全可靠的。
此程式碼已針對低資料集進行了測試。我們永遠不能相信使用者的輸入。
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn