在 C# 中查詢字串中的子字串
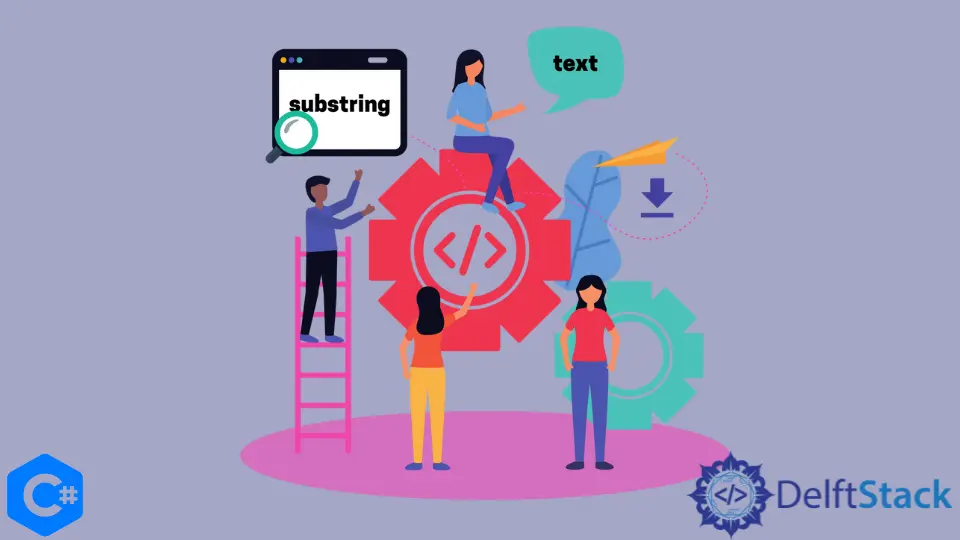
本教程將討論在 C# 中提取字串中兩個單詞之間的文字的方法。
從 C# 中的字串中提取文字
如果我們有一個字串變數,其值類似於嗨,我是字串變數
,而我們想在單詞嗨
和字串
之間找到文字,則可以使用 String.IndexOf()
方法以及實現該目標的 String.SubString()
方法。
String.IndexOf(x)
方法獲取字串內部特定字串 x
的索引。String.SubString(x, y)
方法根據開始索引 x
和結束索引 y
提取子字串。我們可以使用 String.IndexOf()
函式獲得主字串中開始和結束字串的索引。然後,我們可以通過將兩個單詞的索引傳遞給 String.SubString()
函式來提取兩個字串之間的文字。以下程式碼示例向我們展示瞭如何使用 C# 中的 String.IndexOf()
和 String.SubString()
方法從字串中提取文字。
using System;
namespace text_from_string {
class Program {
public static string stringBetween(string Source, string Start, string End) {
string result = "";
if (Source.Contains(Start) && Source.Contains(End)) {
int StartIndex = Source.IndexOf(Start, 0) + Start.Length;
int EndIndex = Source.IndexOf(End, StartIndex);
result = Source.Substring(StartIndex, EndIndex - StartIndex);
return result;
}
return result;
}
static void Main(string[] args) {
string s = "Hi, I am a string variable.";
string word1 = "Hi";
string word2 = "string";
string text = stringBetween(s, word1, word2);
Console.WriteLine(text);
}
}
}
輸出:
, I am a
在上面的程式碼中,我們定義了函式 stringBetween()
,該函式將主字串和兩個單詞都作為引數,並返回主字串中單詞之間的文字。我們使用 Source.IndexOf(Start, 0) + Start.Length
語句初始化了文字的起始索引 StartIndex
。該語句獲取 Source
字串內 Start
字串的索引,然後以 Start
字串的長度遞增該索引,以使 Start
不會出現在文字結果中。對文字的結束索引 EndIndex
執行相同的過程。然後,我們將 StartIndex
作為起始索引,將 EndIndex-StartIndex
作為新字串的長度提供給 String.SubString()
函式,以返回文字。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn