在 C# 中不區分大小寫的字串包含函式
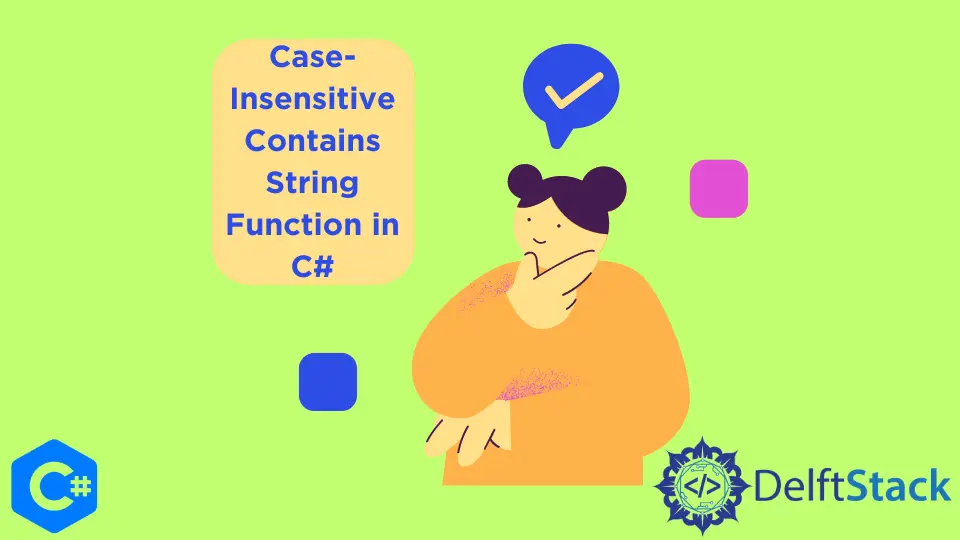
本教程將介紹如何在 C# 中以不區分大小寫的方式建立一個檢查字串是否包含另一個字串的函式。
在 C# 中使用 string.IndexOf()
函式建立不區分大小寫的包含函式
當我們談論不區分大小寫的字串時,第一個直覺是考慮 string.ToUpper()
和 string.ToLower()
函式。但是,這不是一個好方法,因為在執行這些函式後,許多字元會會變成完全不同的字母。如果我們確實想在 C# 中建立不區分大小寫的包含檢查函式的程式碼,則必須使用一些不同的方法。
string.IndexOf(string1, StringComparison)
函式用於根據 StringComparison
引數指定的比較型別,獲取 string1
引數在 string
裡面的第一次出現。它返回一個整數值,該整數值表示在 string
內部出現 string1
引數的第一個索引。StringComparison.OrdinalIgnoreCase
引數指定編譯器在查詢索引時忽略大小寫。以下程式碼示例向我們展示瞭如何在為 C# 中使用 string.IndexOf()
函式和 StringComparison.OrdinalIgnoreCase
作引數,建立不區分大小寫的 contains(string)
函式。
using System;
using System.Globalization;
namespace case_insensitive_string_contains {
class Program {
static void Main(string[] args) {
string mainString = "This is the Main String";
string wordToCheck = "string";
bool result = mainString.IndexOf(wordToCheck, StringComparison.OrdinalIgnoreCase) >= 0;
if (result == true) {
Console.WriteLine("{0} is present in {1}", wordToCheck, mainString);
} else {
Console.WriteLine("{0} is not present in {1}", wordToCheck, mainString);
}
}
}
}
輸出:
string is present in This is the Main String
我們建立了一個不區分大小寫的包含函式,使用 string.IndexOf()
函式和 StringComparison.OrdinalIgnoreCase
作為 C# 中的引數來確定 mainString
字串中是否存在 wordToCheck
字串。我們將比較結果儲存在布林變數 result
中並顯示答案。
在 C# 中使用 CultureInfo
類建立不區分大小寫的包含函式
CultureInfo
類包含有關不同文化的資訊,例如名稱,寫作風格,使用的日曆型別等等。CultureInfo.CompareInfo.IndexOf()
函式用於確定一個字串在相同區域性的另一個字串中首次出現的索引。我們可以使用 CompareOptions.IgnoreCase
作為引數來查詢時忽略大小寫索引。以下程式碼示例向我們展示瞭如何使用 CultureInfo.CompareInfo.IndexOf()
函式和 CompareOptions.IgnoreCase
作為 C# 中的引數,建立不區分大小寫的 contains(string)
函式。
using System;
using System.Globalization;
namespace case_insensitive_string_contains {
class Program {
static void Main(string[] args) {
string mainString = "This is the Main String";
string wordToCheck = "string";
CultureInfo culture = new CultureInfo("");
bool result =
culture.CompareInfo.IndexOf(mainString, wordToCheck, CompareOptions.IgnoreCase) >= 0;
if (result == true) {
Console.WriteLine("{0} is present in {1}", wordToCheck, mainString);
} else {
Console.WriteLine("{0} is not present in {1}", wordToCheck, mainString);
}
}
}
}
輸出:
string is present in This is the Main String
我們建立了一個不區分大小寫的包含函式來確定 wordToCheck
字串是否存在於 mainString
字串中,並以 CultureInfo.CompareInfo.IndexOf()
函式和 CompareOptions.IgnoreCase
作為 C# 中的引數。我們將比較結果儲存在布林變數 result
中並顯示答案。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn