C++ 中向量的最大值和最小值
-
在 C++ 中使用
for
迴圈從向量中查詢最大值和最小值 -
在 C++ 中使用
std::max_element
和std::min_element
函式從向量中獲取最大值和最小值 -
C++ 中使用
std::minmax_element
函式從向量中獲取最大值和最小值
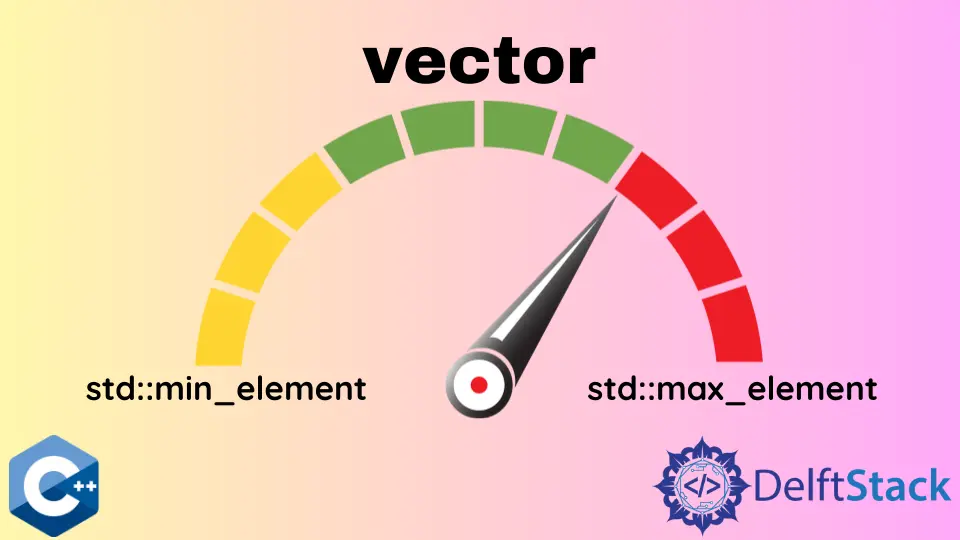
C++ 中的向量只不過是一個可以自動調整自身大小的動態陣列。由於向量是元素的容器,我們可能想找出向量包含的最大值或最小值。
在處理向量時,我們可以像在陣列中搜尋最大或最小元素一樣使用迴圈。本文還將介紹一些幫助我們做同樣事情的庫函式。
在 C++ 中使用 for
迴圈從向量中查詢最大值和最小值
在下面的示例中,我們有兩個函式模板; maxElement
用於查詢最大元素,minElement
用於查詢最小元素。在 main()
塊中,我們定義了一個向量 marks
並將其傳遞給模板函式。
minElement
函式和 maxElement
函式的返回值分別儲存在變數 min,
和 max
中,並將這些變數列印到螢幕上。maxElement
函式有一個變數 max
,其中包含巨集 INT_MIN
值,然後我們使用 for
迴圈遍歷所有向量元素並將它們與儲存在 max
中的值進行比較。
如果我們發現一個元素大於儲存在其中的值,我們會更新 max
的值,並且對向量的所有元素都執行此操作。函式 maxElement
返回變數 max
的最後更新值,這就是我們從向量 marks
中獲取最大值的方式。
minElement
函式也以相同的方式工作並返回向量的最小值。讓我們舉個例子,使用迴圈從 C++ 中的向量中找到最大值和最小值。
程式碼:
#include <climits>
#include <iostream>
#include <vector>
using namespace std;
template <typename D>
int maxElement(vector<D> const &v) {
int max = INT_MIN;
for (const D &i : v) {
if (max < i) {
max = i;
}
}
return max;
}
template <typename D>
int minElement(vector<D> const &v) {
int min = INT_MAX;
for (const D &i : v) {
if (min > i) {
min = i;
}
}
return min;
}
int main() {
vector<int> marks = {23, 45, 65, 23, 43, 67, 87, 12};
int min = minElement(marks);
int max = maxElement(marks);
cout << "The minimum marks are: " << min << endl;
cout << "The maximum marks are: " << max << endl;
return 0;
}
輸出:
The minimum marks are: 12
The maximum marks are: 87
這是一種在 C++ 中從向量中查詢最大和最小元素的簡單方法。
在 C++ 中使用 std::max_element
和 std::min_element
函式從向量中獲取最大值和最小值
要在 C++ 中從向量中找到最大值和最小值,我們可以分別使用 std::max_element
和 std::min_element
函式。
max_element
函式返回一個指向最大值的迭代器,min_element
函式返回一個指向最小值的迭代器,兩者都在 (start, end)
範圍內。
語法:
*min_element(start_index, end_index);
*max_element(start_index, end_index);
在下面的示例中,我們首先將指向向量開始和結束的指標 marks
作為引數傳遞給函式 max_element
和 min_element
。max_element
和 min_element
函式返回的值分別儲存在變數 max
和 min
中。
程式碼:
#include <algorithm>
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> marks = {23, 34, 56, 75, 23, 44, 58};
int max = *max_element(marks.begin(), marks.end());
int min = *min_element(marks.begin(), marks.end());
cout << "The minimum marks are: " << min << endl;
cout << "The maximum marks are: " << max << endl;
return 0;
}
輸出:
The minimum marks are: 23
The maximum marks are: 75
請注意,這兩個函式都可以在預定義函式的幫助下進行比較。在此處閱讀有關這些功能的更多資訊。
C++ 中使用 std::minmax_element
函式從向量中獲取最大值和最小值
std::minmax_element
更像是上述兩個函式的濃縮版本。我們可以使用 std::minmax_element
函式來獲取一對迭代器作為返回值,而不是單獨使用它們。
此函式將返回一對迭代器,其中第一個值指向最小元素,第二個值指向最大元素。例如,我們將向量的第一個和最後一個索引 marks
傳遞給函式 minmax_element
,該函式返回的值儲存在變數 res
中,該變數使用 auto
定義關鍵詞。
然後,我們使用 dot(.)
運算子從這對迭代器中分離出最小值和最大值,並將它們儲存在變數 min
和 max
中。我們使用 minmax_element
函式從 C++ 中的向量中獲取最大值和最小值。
程式碼:
#include <algorithm>
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> marks = {34, 23, 56, 75, 23, 67, 88, 12};
auto res = minmax_element(marks.begin(), marks.end());
int min = *res.first;
int max = *res.second;
cout << "The minimum marks are: " << min << endl;
cout << "The maximum marks are: " << max << endl;
return 0;
}
輸出:
The minimum marks are: 12
The maximum marks are: 88
下面是上述程式碼的修改版本,它還返回向量的最大值和最小值的索引。這是使用 std::distance
函式完成的,該函式計算第一個和最後一個元素之間的元素數。
程式碼:
#include <algorithm>
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> marks = {34, 23, 56, 75, 23, 67, 88, 12};
auto res = minmax_element(marks.begin(), marks.end());
int min = *res.first;
int max = *res.second;
int indx_max = distance(marks.begin(), res.first);
int indx_min = distance(marks.begin(), res.second);
cout << "The minimum marks are: " << min << endl;
cout << "Found at index: " << indx_min << endl;
cout << "The maximum marks are: " << max << endl;
cout << "Found at index: " << indx_max << endl;
return 0;
}
輸出:
The minimum marks are: 12
Found at index: 6
The maximum marks are: 88
Found at index: 7
要了解有關此功能的更多資訊,請檢視此文件。這就是我們如何從 C++ 中的向量中獲取最大值和最小值的全部內容。