C++ 中用於型別推斷的 auto 關鍵字
Suraj P
2023年10月12日
-
C++ 中的
auto
關鍵字 -
在 C++ 中將
auto
與函式一起使用 -
在 C++ 中對變數使用
auto
-
在 C++ 中將
auto
與迭代器一起使用 -
在 C++ 中將
auto
與函式引數一起使用 -
在 C++ 中使用
auto
關鍵字的錯誤 - まとめ
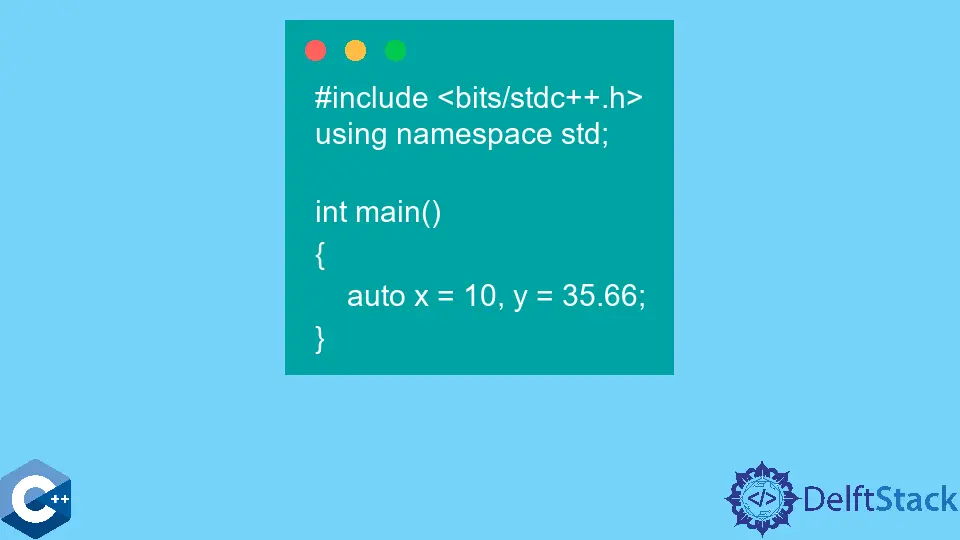
本教程將解決 C++ 11 中引入的 auto
關鍵字。我們將檢視不同的示例以瞭解其用例和使用時的常見錯誤。
C++ 中的 auto
關鍵字
C++ 11 版本中的關鍵字 auto
是 Type inference
功能的一部分。型別推斷是指在編譯時推斷函式或變數的資料型別。
所以我們不必指定資料型別編譯器可以在編譯時自動推斷它。return 語句幫助我們推斷函式的返回資料型別。
而在變數的情況下,初始化有助於確定資料型別。
在 C++ 中將 auto
與函式一起使用
#include <iostream>
using namespace std;
auto division() {
double a = 55.0;
double b = 6.0;
double ans = a / b;
return ans;
}
int main() { cout << division(); }
輸出:
9.16667
這裡的 return 語句幫助編譯器推斷我們的 division()
函式的返回型別。由於函式返回雙精度型別 ans
,編譯器推斷 auto
必須由雙精度型別替換。
在 C++ 中對變數使用 auto
#include <iostream>
#include <typeinfo>
using namespace std;
int main() {
auto x = 10;
auto y = 10.4;
auto z = "iron man";
cout << x << endl;
cout << y << endl;
cout << z << endl;
}
輸出:
10
10.4
iron man
基於語句的右側,也稱為 initializers
,編譯器推斷變數的型別。
在 C++ 中將 auto
與迭代器一起使用
在處理向量、集合或對映等 STL 容器時,auto
關鍵字被高度用於迭代它們。我們可以通過跳過冗長的迭代器宣告來最小化時間損失。
#include <bits/stdc++.h>
using namespace std;
int main() {
vector<int> v{22, 14, 15, 16};
vector<int>::iterator it = v.begin(); // without auto
auto it2 = v.begin();
while (it2 != v.end()) {
cout << *it2 << " ";
it2++;
}
cout << endl;
map<int, int> mp;
mp.insert(pair<int, int>(1, 40));
mp.insert(pair<int, int>(2, 30));
mp.insert(pair<int, int>(3, 60));
mp.insert(pair<int, int>(4, 20));
map<int, int>::iterator vt = mp.begin(); // without auto
auto vt2 = mp.begin();
while (vt2 != mp.end()) {
cout << vt2->first << " -> " << vt2->second << endl;
vt2++;
}
cout << endl;
}
輸出:
22 14 15 16
1 -> 40
2 -> 30
3 -> 60
4 -> 20
我們觀察使用 auto
和不使用之間的區別。如果沒有 auto
,我們必須明確指定迭代器的型別,這很乏味,因為語句很長。
在 C++ 中將 auto
與函式引數一起使用
在 C++ 中,我們必須每次都指定函式引數,但這可以通過使用 auto
來簡化。
#include <bits/stdc++.h>
using namespace std;
void myfunc(auto x, auto str) { cout << x << " " << str << endl; }
int main() {
int x = 10;
string str = "Iron Man";
myfunc(x, str);
}
輸出:
10 Iron Man
資料型別的推斷髮生在函式呼叫期間。因此,會發生變數匹配,並且編譯器會計算出所有引數的確切資料型別。
在 C++ 中使用 auto
關鍵字的錯誤
整數與布林混淆
在 C++ 中,我們知道布林值被初始化為 0
或 1
。現在,這可能會在除錯時造成一些混亂。
#include <bits/stdc++.h>
using namespace std;
int main() { auto temp = 0; }
使用 auto
關鍵字的多個宣告
我們一次性宣告變數以節省時間,但如果兩者的型別不同,這可能會產生問題。
#include <bits/stdc++.h>
using namespace std;
int main() { auto x = 10, y = 35.66; }
輸出:
[Error] inconsistent deduction for 'auto': 'int' and then 'double'
當我們在編譯器中編譯上面的程式碼時,我們會得到一個錯誤,這是因為編譯器被混淆了。
まとめ
我們瞭解到,auto
關鍵位元組省了大量編寫基本程式碼的時間,專注於程式中更密集和更復雜的部分。雖然非常有用,但我們必須小心使用它。