Como verificar se uma string contém um substrato em Python
-
Operador
in
para verificar se uma string contém uma substring -
str.find()
método para verificar se uma string contém substring -
str.index()
Método - Solução de verificação do substrato Conclusão
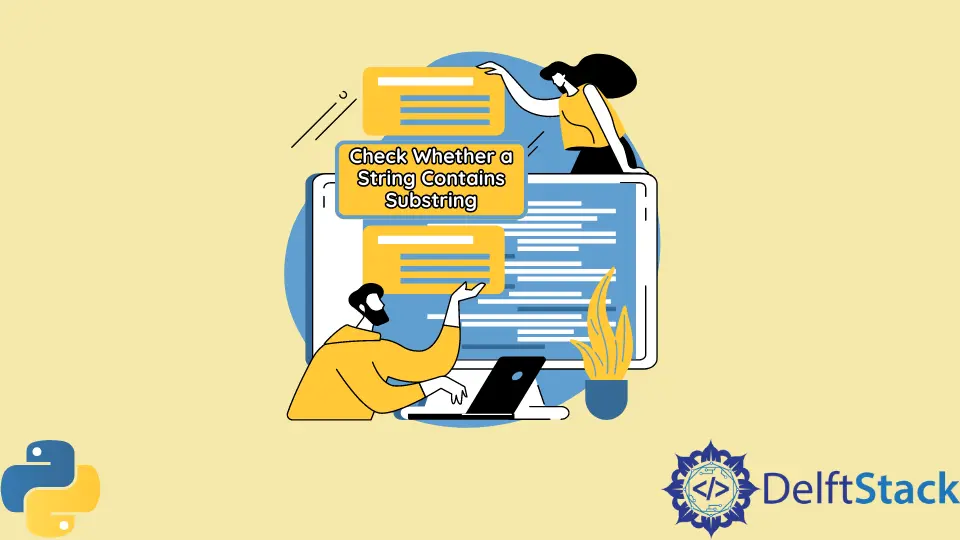
É bastante frequente precisarmos verificar se a string em questão contém um substrato específico. Vamos listar alguns métodos aqui, e depois comparar o desempenho do tempo de execução para selecionar o método mais eficiente.
Nós iremos pegar a string - It is a given string
como a string dada e given
é a substring a ser verificada.
Operador in
para verificar se uma string contém uma substring
in
operator é o operador de verificação de membros. O x in y
é avaliado como True
se x
é um membro de y
, ou em outras palavras, y
contém x
.
Ele retorna True
se a string y
contém o substring x
.
>>> "given" in "It is a given string"
True
>>> "gaven" in "It is a given string"
False
Desempenho do Operador in
import timeit
def in_method(given, sub):
return sub in given
print(min(timeit.repeat(lambda: in_method("It is a given string", "given"))))
0.2888628
str.find()
método para verificar se uma string contém substring
O find
é um método embutido de string
- str.find(sub)
.
Ele retorna o índice mais baixo em str
onde substring sub
é encontrado, caso contrário retorna -1
se sub
não for encontrado.
>>> givenStr = 'It is a given string'
>>> givenStr.find('given')
8
>>> givenStr.find('gaven')
-1
str.find()
Desempenho do Método
import timeit
def find_method(given, sub):
return given.find(sub)
print(min(timeit.repeat(lambda: find_method("It is a given string", "given"))))
0.42845349999999993
str.index()
Método
str.index(sub)
is a string
built-in method that returns the lowest index in str
where sub
is found. It will raise ValueError
when the substring sub
is not found.
>>> givenStr = 'It is a given string'
>>> givenStr.index('given')
8
>>> givenStr.index('gaven')
Traceback (most recent call last):
File "<pyshell#7>", line 1, in <module>
givenStr.index('gaven')
ValueError: substring not found
str.index()
Desempenho do Método
import timeit
def find_method(given, sub):
return given.find(sub)
print(min(timeit.repeat(lambda: find_method("It is a given string", "given"))))
0.457951
Solução de verificação do substrato Conclusão
- O operador
in
é aquele que você deve utilizar para verificar se existe um substrato na string dada, pois é o mais rápido - O
str.find()
estr.index()
também poderiam ser utilizados, mas não o ideal, devido ao mau desempenho
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook