Fila de prioridade em C#
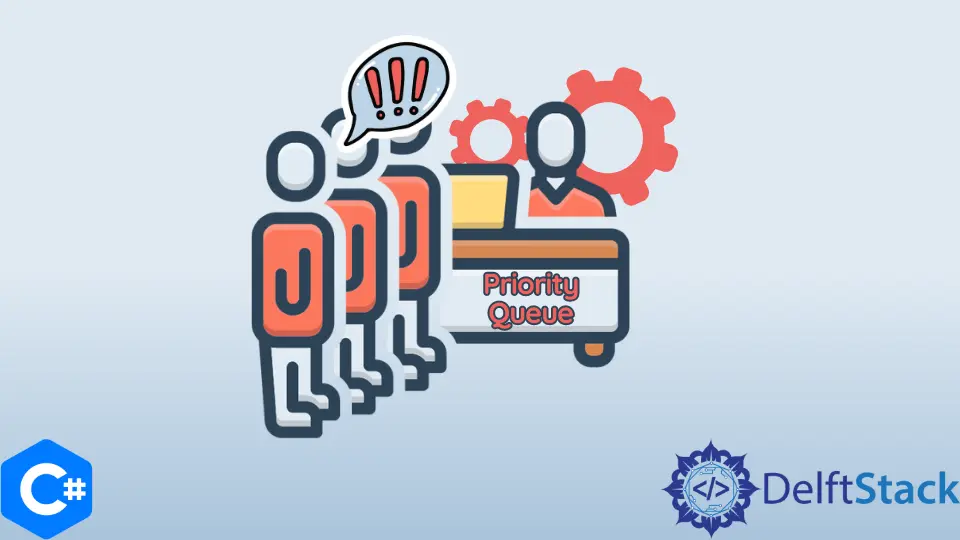
Este artigo apresentará uma fila de prioridade equivalente em C#.
Use a Lista
como fila de prioridade em C#
Em C#, não há classe especial para a fila de prioridade. Mas podemos implementá-lo usando arrays e listas. Usaremos a estrutura de dados da lista para implementar uma fila de prioridade. Uma fila de prioridade é um tipo de dado abstrato que possui uma prioridade associada a seus elementos. A lista será criada de forma que a prioridade mais alta esteja sempre no topo. O algoritmo para implementar uma fila de prioridade usando a lista é o seguinte.
PUSH(HEAD, VALUE, PRIORITY)
Step 1 : Create a new node with VALUE and PRIORITY Step 2
: Check if HEAD has lower priority.If true follow Steps 3 -
4 and end.Else goto Step 5. Step 3 : NEW->NEXT = HEAD Step 4 : HEAD = NEW Step 5
: Set TEMP to head of the list Step 6
: While TEMP->NEXT != NULL and TEMP->NEXT->PRIORITY > PRIORITY Step 7
: TEMP = TEMP->NEXT[END OF LOOP] Step 8 : NEW->NEXT = TEMP->NEXT Step 9
: TEMP->NEXT = NEW Step 10 : End POP(HEAD) Step 2
: Set the head of the list to the next node in the list.HEAD = HEAD->NEXT.Step 3
: Free the node at the head of the list Step 4 : End TOP(HEAD)
: Step 1 : Return HEAD->VALUE Step 2 : End
A operação push adicionará elementos à fila de prioridade de forma que o elemento com a prioridade mais alta esteja sempre no topo. A operação pop removerá o elemento com a prioridade mais alta da fila. A operação superior irá recuperar o elemento de maior prioridade sem removê-lo. O programa abaixo mostra como podemos fazer push, pop e elementos principais de uma fila prioritária.
using System;
class PriorityQueue {
public class Node {
public int data;
public int priority;
public Node next;
}
public static Node node = new Node();
public static Node newNode(int d, int p) {
Node temp = new Node();
temp.data = d;
temp.priority = p;
temp.next = null;
return temp;
}
public static int top(Node head) {
return (head).data;
}
public static Node pop(Node head) {
Node temp = head;
(head) = (head).next;
return head;
}
public static Node push(Node head, int d, int p) {
Node start = (head);
Node temp = newNode(d, p);
if ((head).priority > p) {
temp.next = head;
(head) = temp;
} else {
while (start.next != null && start.next.priority < p) {
start = start.next;
}
temp.next = start.next;
start.next = temp;
}
return head;
}
public static int isEmpty(Node head) {
return ((head) == null) ? 1 : 0;
}
public static void Main(string[] args) {
Node queue = newNode(1, 1);
queue = push(queue, 9, 2);
queue = push(queue, 7, 3);
queue = push(queue, 3, 0);
while (isEmpty(queue) == 0) {
Console.Write("{0:D} ", top(queue));
queue = pop(queue);
}
}
}
Resultado:
3 1 9 7
O elemento que tem menos valor de prioridade
tem prioridade mais alta.