TypeScript에서 런타임 시 개체 유형 확인
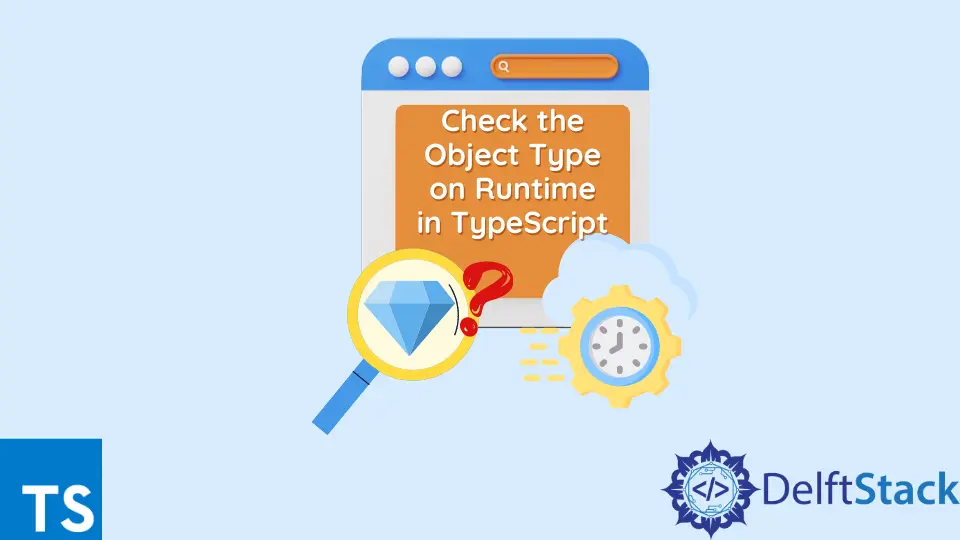
이 문서에서는 TypeScript에서 런타임에 개체 유형을 확인하는 방법에 대해 설명합니다.
TypeScript의 주요 유형
TypeScript는 강력한 형식의 언어입니다. 따라서 컴파일 시간에 유형을 확인하여 런타임 오류를 줄입니다.
TypeScript에는 다음과 같이 몇 가지 기본 유형이 있습니다.
- 문자열, 숫자 및 부울과 같은 기본 유형
- 사용자 정의 클래스 유형
- 인터페이스 유형
- 조합 유형
- 유형 별칭
TypeScript에서 런타임 시 클래스 유형 확인
TypeScript는 EcmaScript 6의 class
키워드를 지원합니다. OOP 방식으로 코드를 작성하는 데 사용할 수 있습니다.
Engineer
클래스를 만들어 보겠습니다.
class Engineer {
}
이 클래스에는 속성이나 동작이 포함되어 있지 않으므로 메서드를 추가해 보겠습니다.
class Engineer {
visitSite(): void {
console.log("This is the Engineer class");
}
}
다음과 같이 Doctor
라는 또 다른 클래스가 있다고 가정해 보겠습니다.
class Doctor {
visitWard(): void {
console.log("This is the Doctor class");
}
}
Engineer
및 Doctor
는 두 가지 사용자 정의 유형입니다. 일부 시나리오에서는 기존 개체 유형이 Engineer
또는 Doctor
클래스에 속하는지 확인해야 합니다.
이것은 TypeScript instanceof
연산자를 사용하면 매우 간단합니다.
instanceof
연산자를 사용하여 클래스 유형 확인
주어진 객체가 TypeScript 클래스의 인스턴스인지 생성자인지 확인합니다. 다단계 상속을 고려하여 해당 클래스가 다른 수준에 나타나는지 확인합니다.
일치하는 클래스가 발견되면 true
를 반환합니다. 그렇지 않으면 false
가 출력됩니다.
통사론:
my_object instanceof my_custom_class_type
이 연산자를 사용하여 TypeScript 로직 내에서 Engineer
와 Doctor
클래스를 구별해 보겠습니다.
먼저 새로운 검사기 함수 checkObjectType()
을 만듭니다.
function checkObjectType(myObject) {
if (myObject instanceof Engineer) {
myObject.visitSite()
}
if (myObject instanceof Doctor) {
myObject.visitWard()
}
}
위의 검사기 기능에서 myObject
의 클래스를 확인합니다. 결과를 기반으로 우리는 속한 클래스 메소드를 visitSite()
또는 visitWard()
라고 부릅니다.
두 클래스에서 개체를 시작하고 개체를 checkObjectType
메서드에 전달해 보겠습니다.
let engineerObject: Engineer = new Engineer;
let doctorObject: Doctor = new Doctor;
checkObjectType(engineerObject);
checkObjectType(doctorObject);
출력:
예상대로 TypeScript는 제공된 각 개체에 대해 올바른 클래스를 식별합니다.
TypeScript에서 런타임 시 인터페이스 유형 확인
instanceof
는 주어진 인스턴스가 클래스에 속하는지 여부를 확인하는 데 사용할 수 있습니다. 그러나 이것은 TypeScript 인터페이스 유형이나 유형 별칭에서는 작동하지 않습니다.
런타임에는 이러한 모든 인터페이스 유형이 사라집니다. 따라서 일반적인 JavaScript typeof
연산자는 출력을 object
로 제공합니다.
다음과 같이 Airplane
과 Car
라는 두 개의 인터페이스를 정의해 보겠습니다.
interface Airplane {
hasWings() {
console.log("2 wings");
}
}
interface Car {
hasWheels() {
console.log("4 wheels");
}
}
다음으로, 주어진 객체가 Airplane
또는 Car
유형에 속하는지 확인하고 객체를 호환 가능한 유형으로 좁히기 위해 TypeScript 사용자 정의 유형 가드를 생성합니다. 여기서 반환 유형으로 유형 술어를 사용해야 합니다.
유형 술어의 구문:
my_parameter_name is custom_type
my_parameter_name
은 함수에 전달된 현재 인수여야 합니다. 이 경우 custom_type
은 Airplane
또는 Car
가 됩니다.
두 가지 유형 가드를 정의해 보겠습니다.
function isAirplane(anyObject: any): anyObject is Airplane {
return (anyObject as Airplane).hasWings() !=== undefined;
}
전달된 객체 anyObject
에 hasWings()
메서드를 사용할 수 있는지 확인하여 객체의 모양이 인터페이스 모양과 동일한지 확인합니다. 그런 다음 객체를 비행기
유형으로 좁히는 유형 술어를 반환합니다.
isCar()
함수를 유사하게 구현할 수 있습니다.
function isCar(anyObject: any): anyObject is Car {
return (anyObject as Car).hasWheels() !=== undefined;
}
마지막으로 testType()
함수를 구현하여 코드를 테스트할 수 있습니다.
let car: Car = {wheels: 4};
let flight: Airplane = {wings: 2};
function testType(obj: any) {
if (isAirplane(obj)) {
// 'obj' is narrowed to type 'Airplane'
console.log("Airplane wings: "+ obj.wings);
}
else if (isCar(obj)) {
// 'obj' is narrowed to type 'Car'
console.log("Car wheels: "+ obj.wheels);
}
}
testType(car);
testType(flight);
출력:
사용자 정의 유형 가드의 도움으로 런타임에 인터페이스의 정확한 유형을 확인할 수 있습니다.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.