TypeScript의 다중 상속
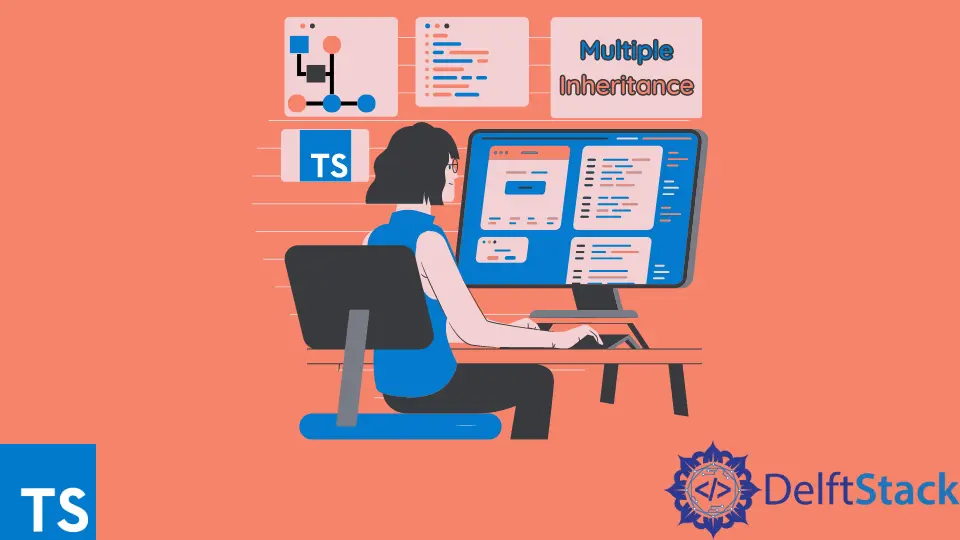
몇 년 동안 몇 가지 프로그래밍 패러다임이 발전했습니다. 객체 지향 프로그래밍(OOP)은 실제 개체와 이들의 상호 작용을 기반으로 하는 주요 패러다임 중 하나입니다.
상속은 하위 엔터티가 부모 엔터티의 속성과 동작을 획득할 수 있도록 하는 잘 알려진 OOP 개념 중 하나입니다.
TypeScript의 단일 상속
TypeScript는 어느 정도까지 OOP 기술을 지원합니다. 이것은 프로그래머가 코드를 효과적으로 재사용할 수 있도록 하는 하나의 상위 엔티티로부터의 상속을 지원합니다.
다음과 같이 extents
키워드를 사용하여 상위 엔터티에서 상속할 수 있습니다.
class Animal {
constructor() {
console.log("Animal class");
}
}
class Cat extends Animal {
constructor() {
super();
}
}
const cat = new Cat();
출력:
"Animal class"
이것은 주어진 클래스가 하나의 부모 클래스로만 확장할 수 있는 단일 상속이라고 합니다.
TypeScript 클래스를 사용한 다중 상속
Java와 유사한 언어로 둘 이상의 상위 엔티티로부터 속성과 동작을 획득하는 것이 가능합니다. 이것을 다중 상속이라고 합니다.
TypeScript는 다중 상속을 지원하지 않습니다.
extens
를 사용하여 TypeScript에서 여러 부모 클래스를 확장해 보겠습니다.
class Engineer {
constructor() {
console.log("Employee class");
}
}
class Person {
constructor() {
console.log("Person class");
}
}
class Employee extends Engineer, Person {
}
그런 다음 다음과 같이 TypeScript 코드를 변환해 보겠습니다.
tsc example1.ts
출력:
예상대로 TypeScript가 단일 클래스 확장만 허용한다는 오류가 발생합니다.
TypeScript 인터페이스를 통한 다중 상속
TypeScript 인터페이스는 기본적으로 다중 상속을 지원합니다. 둘 이상의 클래스를 확장할 수 있습니다.
Person
과 Engineer
라는 두 개의 클래스를 만들고 몇 가지 더미 메서드를 추가해 보겠습니다.
class Person {
name: string;
constructor() {
console.log("Person class");
}
sayImAPerson(){
console.log("Hey, I am a person");
}
}
class Engineer {
salary: number;
constructor() {
console.log("Engineer class");
}
sayImAnEngineer(){
console.log("I am an engineer too");
}
}
다음으로 우리는 위의 두 클래스를 확장하기 위해 새로운 유형이나 클래스를 생성할 것입니다.
class Employee {
empId: string;
}
Employee
클래스는 둘 이상의 상위 클래스를 확장할 수 없으므로 TypeScript 선언 병합을 사용하려면 Employee
라는 이름의 인터페이스를 사용해야 합니다. 이러한 방식으로 인터페이스와 클래스를 병합할 수 있습니다.
다음과 같이 Person
및 Engineer
클래스를 확장하는 Employee
인터페이스를 생성해 보겠습니다.
interface Employee extends Person, Engineer {
}
TypeScript 코드를 변환하고 오류가 발생하는지 확인합시다.
출력:
예상대로 인터페이스 선언 내에서 둘 이상의 상위 클래스와 함께 extens
를 사용할 때 오류가 없습니다. 따라서 인터페이스는 다중 상속을 지원합니다.
그러나 상속된 클래스 내에서 메서드를 호출하면 여전히 문제가 있습니다. 메소드 구현에 대한 액세스 권한이 없습니다.
sayImAnEngineer()
메서드를 호출해 보겠습니다.
let emp: Employee = new Employee;
emp.sayImAnEngineer();
먼저 코드를 트랜스파일해야 합니다. 오류가 발생하지 않습니다. 그런 다음 생성된 JavaScript를 실행해 보십시오. 다음 출력과 같이 오류가 발생합니다.
출력:
TypeScript가 sayImAnEngineer
메서드를 찾을 수 없습니다. TypeScript mixins
를 사용하여 이를 극복할 수 있습니다.
TypeScript 믹스인을 사용한 다중 상속
TypeScript 믹스인 메커니즘은 상위 클래스에서 사용 가능한 모든 메서드를 파생 클래스 또는 하위 클래스로 복사할 수 있습니다. 믹스인 생성 메소드는 상위 클래스의 모든 속성을 거쳐 콘텐츠를 검색해야 합니다.
그런 다음 파생 클래스의 모든 내용을 있는 그대로 설정해야 합니다. 공식 TypeScript 문서에서 제공하는 방법이 있습니다: mixin 생성 방법.
다음과 같이 TypeScript 파일 내에서 사용하는 것이 좋습니다.
function applyMixins(derivedCtor: any, constructors: any[]) {
constructors.forEach((baseCtor) => {
Object.getOwnPropertyNames(baseCtor.prototype).forEach((name) => {
Object.defineProperty(
derivedCtor.prototype,
name,
Object.getOwnPropertyDescriptor(baseCtor.prototype, name) ||
Object.create(null)
);
});
});
}
TypeScript 예제에 applyMixins
메소드를 포함시켜 보겠습니다.
class Person {
name: string;
constructor() {
console.log("Person class");
}
sayImAPerson(){
console.log("Hey, I am a person");
}
}
class Engineer {
salary: number;
constructor() {
console.log("Engineer class");
}
sayImAnEngineer(){
console.log("I am an engineer too");
}
}
class Employee {
empId: string;
}
interface Employee extends Person, Engineer {
}
function applyMixins(derivedCtor: any, constructors: any[]) {
constructors.forEach((baseCtor) => {
Object.getOwnPropertyNames(baseCtor.prototype).forEach((name) => {
Object.defineProperty(
derivedCtor.prototype,
name,
Object.getOwnPropertyDescriptor(baseCtor.prototype, name) ||
Object.create(null)
);
});
});
}
이제 다음과 같이 applyMixins
메서드를 호출해 보겠습니다.
applyMixins(Employee, [Person, Engineer]);
첫 번째 매개변수는 파생 클래스여야 합니다. 이 경우 Employee
클래스입니다. 다음 매개변수는 파생 클래스가 확장할 모든 상위 클래스를 포함하는 배열입니다.
마지막으로 Employee
객체를 만들고 Engineer
상위 클래스에서 sayImAnEngineer
메서드를 호출해 보겠습니다.
let emp: Employee = new Employee;
emp.sayImAnEngineer();
출력:
이번에는 오류가 없습니다. 이것은 mixins 기술이 작동했음을 의미합니다. 이런 식으로 TypeScript에서 다중 상속을 구현할 수 있습니다.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.