TypeScript에서 유형 확장
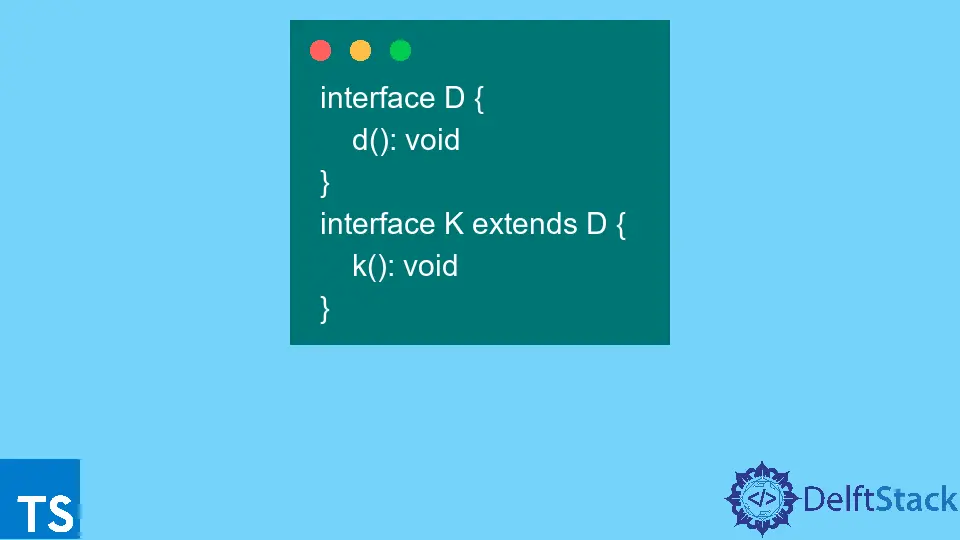
이 기사는 몇 가지 예제를 통해 TypeScript에서 유형을 확장하는 방법을 이해하는 데 도움이 됩니다.
TypeScript에서 유형 확장
안타깝게도 TypeScript에서는 유형을 확장할 수 없습니다. 인터페이스
와 클래스
만 확장할 수 있습니다.
인터페이스나 클래스를 사용하여 유형을 지정하고 확장할 수 있습니다. 예제를 살펴보고 유형에 대한 인터페이스를 확장하려고 합니다.
아래에 설명된 두 가지 방법을 포함하는 Project
라는 인터페이스가 있습니다.
하나는 submit()
이고 두 번째는 queue()
입니다.
interface Project {
submit(data: string): boolean
queue(data: string): boolean
}
project
인터페이스를 사용하는 많은 클래스가 있습니다. 요구 사항을 제출하는 project
인터페이스에 대한 새로운 방법을 사용해야 합니다.
Requirements(data: string, id: number): void
project
인터페이스를 사용하여 현재 코드를 중단하려는 경우 TypeScript는 사용할 수 있는 later()
메서드를 제공합니다. 이를 피함으로써 project
인터페이스를 확장하는 새로운 인터페이스를 만들 수 있습니다.
interface ProjectManagement extends Project {
Requirements(data: string, id: number): boolean
}
extends
키워드를 사용하여 이 구문으로 인터페이스를 확장할 수 있습니다.
interface D {
d(): void
}
interface K extends D {
k(): void
}
인터페이스 K
는 인터페이스 D
를 확장합니다. 둘 다 d()
및 k()
메서드가 있습니다.
ProjectManagement
인터페이스는 클래스처럼 project
인터페이스에서 submit()
및 queue()
두 메서드를 인수합니다.
class PM implements ProjectManagement {
Requirements(data: string, id: number): boolean {
console.log(`Send data to ${id} in ${sec} ms.`);
return true;
}
submit(data: string): boolean {
console.log(`Sent data to ${id} after ${sec} ms. `);
return true;
}
queue(data: string): boolean {
console.log(`Queue an project to ${id}.`);
return true;
}
}
TypeScript에서 여러 인터페이스를 확장하는 인터페이스
인터페이스는 많은 인터페이스를 확장하고 모든 인터페이스의 혼합을 만들 수 있습니다. 예를 들어 토론해 봅시다.
interface A {
a(): void
}
interface E {
e(): void
}
interface M extends E, A {
m(): void
}
인터페이스 M
이 인터페이스 E
및 A
를 확장하는 것을 볼 수 있습니다. M
에는 모든 E
및 A
인터페이스 메서드인 a()
및 e()
가 있습니다.
TypeScript에서 클래스를 확장하는 인터페이스
인터페이스는 클래스의 속성과 메서드를 대신합니다. TypeScript는 클래스를 확장하기 위한 인터페이스를 부여합니다.
인터페이스는 클래스의 개인 및 보호 멤버를 인수합니다. 인터페이스는 공용 멤버만 인수할 수 없습니다.
해당 클래스의 인터페이스는 인터페이스가 확장되는 클래스 또는 하위 클래스에 의해서만 구현될 수 있습니다. 인터페이스가 개인 또는 보호 멤버로 클래스를 확장하는 경우를 의미합니다.
인터페이스가 인수한 클래스의 하위 클래스가 아닌 클래스에서 인터페이스를 구현하면 오류가 발생합니다.
다음은 아래의 예입니다.
class Key {
private value: boolean;
}
interface Lock extends Key {
enable(): void
}
class FingerPrint extends Key implements Lock {
enable() { }
}
class Notification extends Key implements Lock {
enable() { }
}
class Identity extends Key { }{
enable() { }
}
인터페이스는 하나 이상의 기존 인터페이스를 확장할 수 있습니다. 클래스 또는 하위 클래스는 해당 클래스의 인터페이스만 구현할 수 있습니다.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn