Rust에서 두 세트 간의 차이 계산하기
Nilesh Katuwal
2023년6월21일
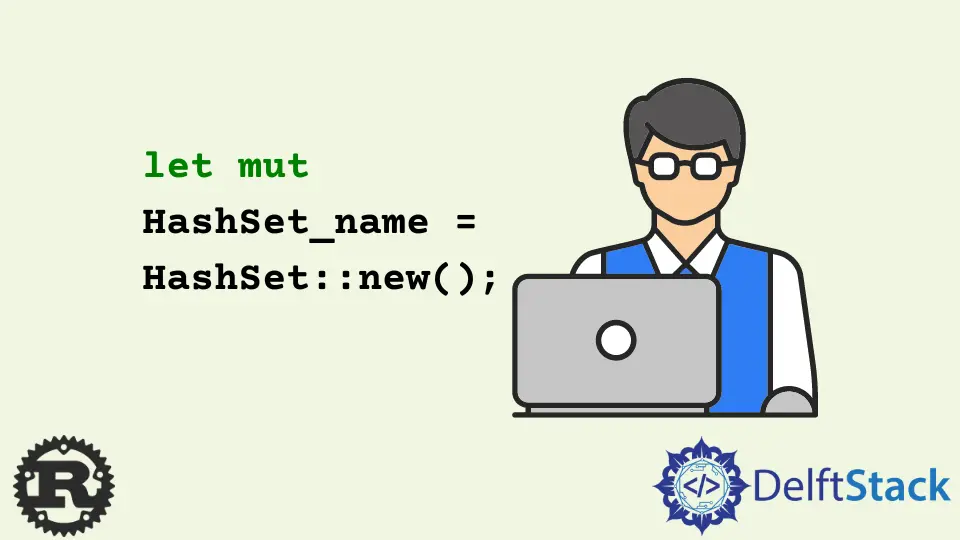
이 기사에서는 두 집합 간의 차이를 계산하는 내장 함수에 대해 설명합니다.
Rust의 해시 세트
해시 세트는 값이 ()
인 HashMap
으로 구현됩니다. 유형과 마찬가지로 HashSet
의 요소는 Eq
및 Hash
특성을 구현해야 합니다.
일반적으로 이것은 #[derive(PartialEq, Eq, hash)]
로 수행할 수 있습니다. 그러나 이를 직접 구현하는 경우 다음과 같은 특성이 유지되어야 합니다.
통사론:
let mut HashSet_name= HashSet::new();
두 개의 키가 같은지 알아야 합니다. 해시도 동일해야 합니다.
예:
fn main() {
use std::collections::HashSet;
let mut foods = HashSet::new();
foods.insert("I love to eat".to_string());
foods.insert("Ramen with cheese".to_string());
foods.insert("Ramen with eggs".to_string());
foods.insert("Ramen with seaweed".to_string());
if !foods.contains("Sesame") {
println!("We have {} foods, but sesame is not the one I wanted.",
foods.len());
}
foods.remove("Ramen with eggs.");
for food in &foods {
println!("{food}");
}
}
출력:
We have 4 foods, but sesame is not the one I wanted.
Ramen with cheese
Ramen with seaweed
Ramen with eggs
I love to eat
HashSet
에 값을 삽입하면(즉, 새 값이 기존 값과 동일하고 둘 다 동일한 해시를 가짐) 새 값이 이전 값을 대체합니다.
세트에는 네 가지 기본 작업이 있습니다(각 후속 호출은 반복자를 반환함).
결합
- 두 집합에서 모든 개별 요소를 가져옵니다.difference
- 두 번째에 없는 첫 번째 집합에서 모든 요소를 검색합니다.교차
- 두 세트 모두에 대한 모든 고유 요소를 검색합니다.대칭 차이
- 두 집합이 아닌 두 집합의 모든 요소를 검색합니다.
다음은 집합 간의 차이를 찾는 예입니다. 우리는 그 차이가 대칭적이지 않다는 것을 알아야 합니다.
fn main() {
use std::collections::HashSet;
let x = HashSet::from([1, 2, 3]);
let y = HashSet::from([4, 2, 3, 4]);
for z in x.difference(&y) {
println!("{z}");
}
let diff: HashSet<_> = x.difference(&y).collect();
assert_eq!(diff, [1].iter().collect());
let diff: HashSet<_> = y.difference(&x).collect();
assert_eq!(diff, [4].iter().collect());
}
출력:
1
Rust에서 두 세트 간의 차이 계산하기
이 프로그램은 두 개의 HashSets
를 빌드하여 정수 요소를 저장하고 두 세트 간의 차이점을 찾고 결과를 표시합니다. 이 두 개의 HashSets
는 다음 소스 코드에 정수 요소를 저장할 수 있습니다.
그런 다음 difference()
기술을 사용하여 두 세트 간의 차이를 결정했습니다. 그런 다음 결과를 인쇄했습니다.
use std::collections::HashSet;
fn main() {
let first_set: HashSet<_> = [10, 20, 30, 40].iter().cloned().collect();
let second_set: HashSet<_> = [10, 20, 30].iter().cloned().collect();
println!("The difference betwen first_set and second_set is:");
for d in first_set.difference(&second_set) {
print!("{} ", d);
}
}
출력:
The difference betwen first_set and second_set is:
40