Python의 슬롯 이해
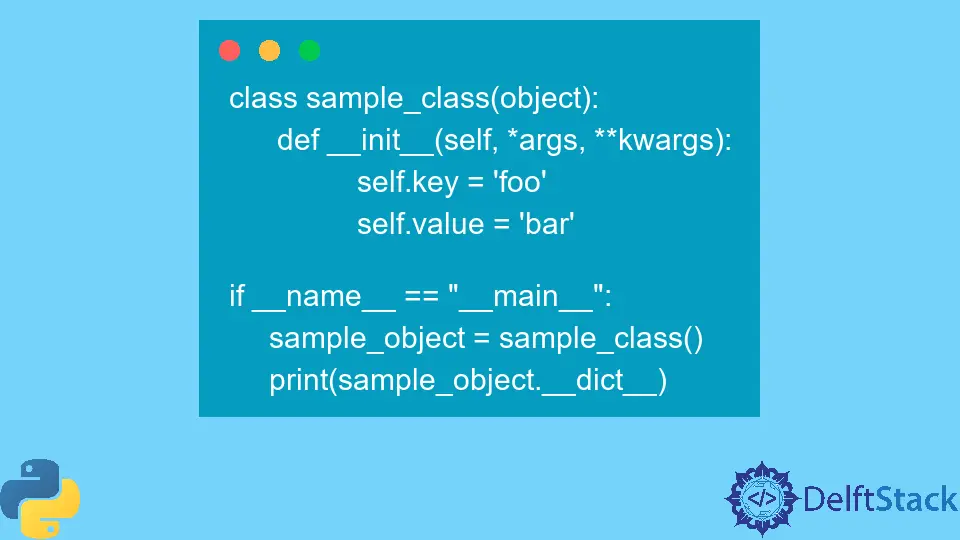
클래스에 대한 객체를 생성하려면 메모리가 필요하며 저장된 속성은 사전 형식입니다. 수천 개의 개체를 할당해야 하는 경우 많은 메모리와 인덱싱 시간이 필요합니다.
슬롯 또는 __slots__
는 개체 크기를 줄이고 인덱싱 속도를 높이는 고유한 메커니즘을 제공합니다. 이 기사에서는 슬롯 상수 변수가 Python에서 작동하는 방식과 사전을 사용하는 것보다 나은 점에 대해 설명합니다.
Python의 슬롯 이해
슬롯은 Python 코드 런타임을 향상시키는 개체에 대한 메모리 최적화 개념입니다. 예를 들어, 클래스에서 사용자 정의 객체를 생성할 때 사전에 저장된 객체의 속성은 __dict__
라고 합니다.
또한 객체를 동적으로 생성한 후 새 속성을 생성할 수 있습니다.
슬롯이 없으면 객체는 아래의 스니펫과 같습니다.
예제 코드:
class sample_class(object):
def __init__(self, *args, **kwargs):
self.key = "foo"
self.value = "bar"
if __name__ == "__main__":
sample_object = sample_class()
print(sample_object.__dict__)
출력:
{'key': 'foo', 'value': 'bar'}
Python에서 각 객체에는 속성 추가를 지원하는 동적 사전이 있습니다. 우리는 모든 인스턴스 개체에 대한 사전 인스턴스를 갖게 되어 추가 공간을 차지하고 많은 RAM을 낭비하게 됩니다.
Python에서 객체를 생성할 때 모든 특성을 저장하기 위해 설정된 양의 메모리를 할당하는 기본 기능이 없습니다.
설정된 수의 속성에 대해 공간을 할당하는 __slots__
를 활용하면 공간 낭비를 줄이고 프로그램 속도를 높일 수 있습니다.
예제 코드:
class sample_class(object):
__slots__ = ["key", "value"]
def __init__(self, *args, **kwargs):
self.key = "foo"
self.value = "bar"
if __name__ == "__main__":
sample_object = sample_class()
print(sample_object.__slots__)
출력:
['key','value']
__slots__
변수를 호출할 때 사전의 각 값을 매핑하기 위한 키에만 액세스합니다. Python의 사전은 매우 직관적이지만 수천 또는 수백만 개의 항목을 동시에 만들려고 하면 문제가 발생할 수 있습니다.
- 사전에는 메모리가 필요합니다. 수백만 개의 개체가 RAM 사용량을 소모합니다.
- 사전은 사실 해시맵입니다.
따라서 사전과 슬롯 간의 런타임 차이는 몇 가지 속성으로 눈에 띄지 않을 수 있지만 데이터가 커질수록 슬롯을 사용하게 되어 기쁩니다. 속성에 액세스할 때 실행 시간의 약 10%를 절약할 수 있습니다.
예제 코드:
import timeit
class sample_class(object):
def __init__(self, *args, **kwargs):
self.key = "foo"
self.value = "bar"
class sample_class_slots(object):
__slots__ = ["key", "value"]
def __init__(self, *args, **kwargs):
self.key = "foo"
self.value = "bar"
if __name__ == "__main__":
time = timeit.timeit("t.key", "from __main__ import sample_class; t=sample_class()")
time_slots = timeit.timeit(
"t.key", "from __main__ import sample_class_slots; t=sample_class_slots()"
)
print("Time without Slots: ", time)
print("Time with Slots: ", time_slots)
출력:
Time without Slots: 0.0202741
Time with Slots: 0.0200698
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn