파이썬에서 예외 다시 던지기
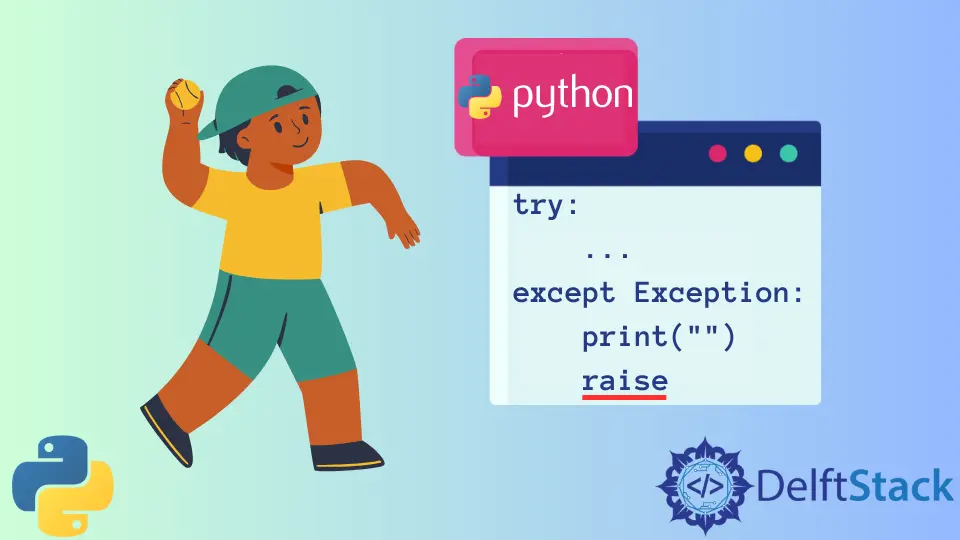
Python은 프로그램에서 예외를 처리하기 위해 try-except
블록을 제공합니다. 또한 수동으로 예외를 throw하는 raise
문을 제공합니다.
이 기사에서는 Python 프로그램에서 예외를 다시 발생시키는 방법에 대해 설명합니다.
Python에서 예외 발생
raise
문을 사용하여 프로그램에서 예외를 throw할 수 있습니다. raise
문의 구문은 다음과 같습니다.
raise exception_name
여기서 raise
문은 exception_name
이라는 예외를 입력으로 사용하고 Python 인터프리터가 처리하는 예외를 throw합니다.
예를 들어 raise
문을 사용하여 프로그램에서 ValueError
예외를 발생시킬 수 있습니다.
-
다음 프로그램은
input()
기능을 사용하여 사용자에게 숫자를 입력하도록 요청합니다.input()
함수는 입력을 변수 번호에 할당된 문자열로 반환합니다. -
그런 다음 프로그램은 입력이 숫자로만 구성되어 있는지(또는 아닌지) 확인합니다. 이를 위해
isdigit()
메서드를 사용합니다.isdigit()
메서드는 문자열에서 호출될 때 문자열의 모든 문자가 십진수인지 여부를 확인합니다. 그렇다면True
를 반환합니다. 그렇지 않으면False
를 반환합니다.
number = input("Please Enter a number:")
if number.isdigit():
number = int(number)
square = number * number
print("The square of {} is {}".format(number, square))
else:
raise ValueError
출력:
Please Enter a number:Aditya
Traceback (most recent call last):
File "/home/aditya1117/PycharmProjects/pythonProject/webscraping.py", line 7, in <module>
raise ValueError
ValueError
위의 프로그램에서 사용자의 입력이 십진수로만 구성된 경우 if
블록의 코드가 실행됩니다. 따라서 입력은 int()
함수를 사용하여 정수로 변환됩니다.
마지막으로 정수의 제곱이 계산되어 출력됩니다.
사용자가 제공한 입력이 10진수 이외의 문자로 구성된 경우 else
문 내부의 코드가 실행되고 프로그램에서 ValueError
예외가 발생합니다.
여기서 ValueError
예외는 기본 제공 예외입니다.
Python에서 사용자 정의 메시지로 예외 발생
사용자 정의 메시지로 사용자 정의 예외를 던질 수도 있습니다. 이를 위해 Exception()
생성자를 사용하여 예외 객체를 생성합니다.
Exception()
생성자는 메시지 문자열을 입력 인수로 사용하고 실행 후 예외를 반환합니다. 다음 예제와 같이 raise
문을 사용하여 사용자 지정 예외를 throw할 수 있습니다.
number = input("Please Enter a number:")
if number.isdigit():
number = int(number)
square = number * number
print("The square of {} is {}".format(number, square))
else:
raise Exception("The input contains characters other than decimal digits.")
출력:
Please Enter a number:Aditya
Traceback (most recent call last):
File "/home/aditya1117/PycharmProjects/pythonProject/webscraping.py", line 7, in <module>
raise Exception("The input contains characters other than decimal digits.")
Exception: The input contains characters other than decimal digits.
여기에서 프로그램이 입력에 십진수 이외의 문자가 포함되어 있습니다.
라는 메시지와 함께 사용자 지정 예외를 발생시키는 것을 볼 수 있습니다.
파이썬에서 예외 다시 던지기
Python의 예외는 try-except
블록을 사용하여 처리됩니다. try
블록에서 예외가 발생하면 except
블록에서 포착되어 적절한 조치가 취해집니다.
아래 예에서 이를 관찰할 수 있습니다.
number = input("Please Enter a number:")
try:
if number.isdigit():
number = int(number)
square = number * number
print("The square of {} is {}".format(number, square))
else:
raise Exception("The input contains characters other than decimal digits.")
except Exception:
print("In the except block. exception handled.")
출력:
Please Enter a number:Aditya
In the except block. exception handled.
여기에서 try
블록에서 예외가 발생합니다. 그런 다음 except
블록에서 예외를 포착하고 필요한 경우 처리하고 적절한 메시지를 인쇄합니다.
Python 프로그램에서 예외를 다시 발생시키려면 아래와 같이 except
블록에서 raise
문을 사용할 수 있습니다.
number = input("Please Enter a number:")
try:
if number.isdigit():
number = int(number)
square = number * number
print("The square of {} is {}".format(number, square))
else:
raise Exception("The input contains characters other than decimal digits.")
except Exception:
print("In the except block. exception handled. Rethrowing exception.")
raise
출력:
Please Enter a number:Aditya
In the except block. exception handled. Rethrowing exception.
Traceback (most recent call last):
File "/home/aditya1117/PycharmProjects/pythonProject/webscraping.py", line 8, in <module>
raise Exception("The input contains characters other than decimal digits.")
Exception: The input contains characters other than decimal digits.
이 예제에서는 먼저 except
블록에서 예외를 포착하고 처리했습니다. 그런 다음 raise
문을 사용하여 Python에서 예외를 다시 발생시켰습니다.
Aditya Raj is a highly skilled technical professional with a background in IT and business, holding an Integrated B.Tech (IT) and MBA (IT) from the Indian Institute of Information Technology Allahabad. With a solid foundation in data analytics, programming languages (C, Java, Python), and software environments, Aditya has excelled in various roles. He has significant experience as a Technical Content Writer for Python on multiple platforms and has interned in data analytics at Apollo Clinics. His projects demonstrate a keen interest in cutting-edge technology and problem-solving, showcasing his proficiency in areas like data mining and software development. Aditya's achievements include securing a top position in a project demonstration competition and gaining certifications in Python, SQL, and digital marketing fundamentals.
GitHub