Python을 사용하여 Excel 파일 읽기
-
Python에서
pandas
패키지를 사용하여 Excel 파일 읽기 -
Python에서
xlrd
패키지를 사용하여 Excel 파일 읽기 - Python에서 Excel 파일에 대해 수행되는 작업의 예
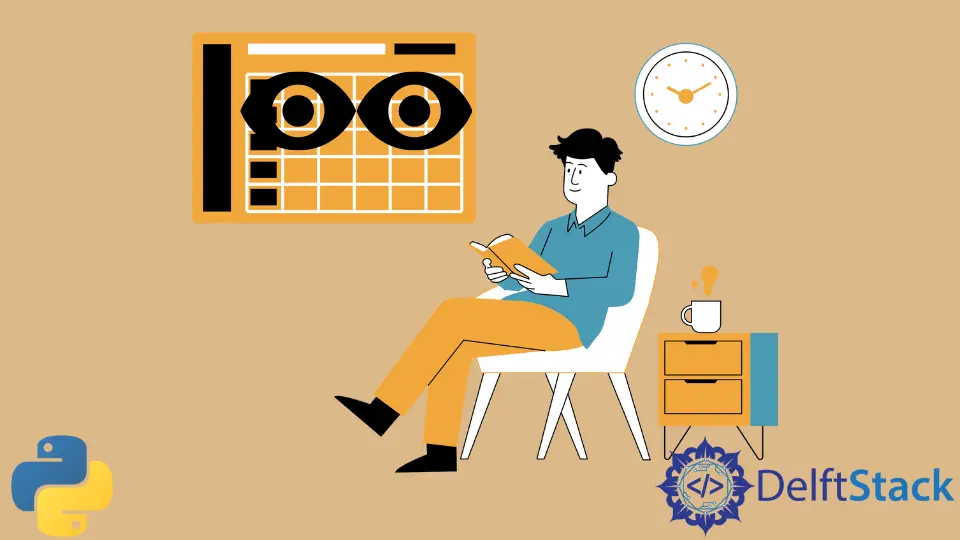
Python 프로그래밍 언어는 데이터 과학 분야에서 사용되는 것으로 잘 알려져 있습니다. 데이터 과학은 일반적으로 라인 플롯, 바이올린 플롯, 히스토그램 및 히트 맵과 같은 그래프 및 플롯과 평균, 중앙값, 모드, 확률, 분산 등과 같은 수학적 계산을 사용하여 데이터를 처리하고 분석하는 것을 포함합니다. 파이썬은 파일 읽기와 조작을 매우 매끄럽게 만든다는 사실이 더 적합합니다. 데이터는 일반적으로 xls
, xlsx
, csv
, txt
등과 같은 인기 있는 파일 형식으로 표현되기 때문에 Python으로 데이터를 처리하는 것은 케이크 조각입니다.
이 기사에서는 몇 가지 예를 통해 Python을 사용하여 Excel 파일을 읽는 방법을 소개합니다. 예를 들어, [여기]에서 다운로드할 수 있는 샘플 Excel 파일을 고려하여 우리 모두가 같은 페이지에 있습니다. 다음 코드 조각이 작동하려면 이름을 sample.xls
로 바꾸거나 다음 코드 조각 자체에서 파일 이름을 변경하십시오.
Python에서 pandas
패키지를 사용하여 Excel 파일 읽기
Python에서는 pandas
라이브러리를 사용하여 Excel 파일을 읽을 수 있습니다. pandas
모듈은 Python으로 작성된 강력하고 강력하며 빠르고 유연한 오픈 소스 데이터 분석 및 조작 라이브러리입니다. 머신이나 가상 환경에 설치되어 있지 않다면 다음 명령어를 사용하세요.
pandas
설치:pip install pandas
또는pip3 install pandas
pandas
모듈을 사용하여 Excel 파일을 읽는 방법은 다음 코드를 참조하십시오.
import xlrd
import pandas
df = pandas.read_excel("sample.xls")
print("Columns")
print(df.columns)
출력:
Columns
Index(['Segment', 'Country', 'Product', 'Discount Band', 'Units Sold',
'Manufacturing Price', 'Sale Price', 'Gross Sales', 'Discounts',
' Sales', 'COGS', 'Profit', 'Date', 'Month Number', 'Month Name',
'Year'],
dtype='object')
Python에서 xlrd
패키지를 사용하여 Excel 파일 읽기
Python에서는 xlrd
패키지를 사용하여 Excel 파일을 읽을 수 있습니다. xlrd
모듈은 Excel 파일을 읽고 서식을 지정하는 데 사용되는 Python 패키지입니다. 컴퓨터 또는 가상 환경에 설치되어 있지 않은 경우 다음 명령을 사용합니다.
xlrd
를 설치하려면 다음 명령을 사용하십시오.
pip install xlrd
또는,
pip3 install xlrd
xlrd
를 사용하여 Excel 파일을 읽는 방법은 다음 코드를 참조하십시오.
from xlrd import open_workbook
wb = open_workbook("sample.xls")
sheet = wb.sheet_by_index(0)
sheet.cell_value(0, 0)
columns = []
print("Columns")
for i in range(sheet.ncols):
columns.append(sheet.cell_value(0, i))
print(columns)
출력:
Columns
['Segment', 'Country', 'Product', 'Discount Band', 'Units Sold', 'Manufacturing Price', 'Sale Price', 'Gross Sales', 'Discounts', ' Sales', 'COGS', 'Profit', 'Date', 'Month Number', 'Month Name', 'Year']
다음은 위의 코드가 하는 일에 대한 간략한 설명입니다. 먼저 open_workbook()
함수를 사용하여 Excel 파일에 대한 파일 설명자를 생성합니다. 그런 다음 파일 포인터를 (0,0)
위치 또는 왼쪽 상단 셀로 재설정합니다. 다음으로 첫 번째 행을 반복하고 모든 열 이름을 변수에 저장합니다. 일반적으로 열 이름은 첫 번째 행에 있습니다. 이것이 코드가 해당 위치를 고려하는 이유입니다. 열 이름이 다른 행에 있는 경우 sheet.cell_value(0, i)
문의 0
값을 원하는 행 번호로 변경할 수 있습니다. 본질적으로 (0, i)
는 y
와 x
좌표를 나타내며, 여기서 y
는 0
이고 x
는 i
입니다.
Python에서 Excel 파일에 대해 수행되는 작업의 예
이 두 라이브러리를 더 잘 이해하기 위해 Excel 파일에 대해 수행할 수 있는 몇 가지 간단한 작업을 살펴보겠습니다.
Excel 파일의 처음 3개 행 인쇄
pandas
패키지 사용
import pandas
df = pandas.read_excel("sample.xls")
count = 3
for index, row in df.iterrows():
print(row, end="\n\n")
if index == count - 1:
break
출력:
Segment Government
Country Canada
Product Carretera
Discount Band None
Units Sold 1618.5
Manufacturing Price 3
Sale Price 20
Gross Sales 32370.0
Discounts 0.0
Sales 32370.0
COGS 16185.0
Profit 16185.0
Date 2014-01-01 00:00:00
Month Number 1
Month Name January
Year 2014
Name: 0, dtype: object
Segment Government
Country Germany
Product Carretera
Discount Band None
Units Sold 1321.0
Manufacturing Price 3
Sale Price 20
Gross Sales 26420.0
Discounts 0.0
Sales 26420.0
COGS 13210.0
Profit 13210.0
Date 2014-01-01 00:00:00
Month Number 1
Month Name January
Year 2014
Name: 1, dtype: object
Segment Midmarket
Country France
Product Carretera
Discount Band None
Units Sold 2178.0
Manufacturing Price 3
Sale Price 15
Gross Sales 32670.0
Discounts 0.0
Sales 32670.0
COGS 21780.0
Profit 10890.0
Date 2014-06-01 00:00:00
Month Number 6
Month Name June
Year 2014
Name: 2, dtype: object
xlrd
패키지 사용
from xlrd import open_workbook
wb = open_workbook("sample.xls")
sheet = wb.sheet_by_index(0)
sheet.cell_value(0, 0)
count = 3
for i in range(1, count + 1):
for j in range(sheet.ncols):
print(sheet.cell_value(i, j), end=", ")
print()
출력:
Government, Canada, Carretera, None, 1618.5, 3.0, 20.0, 32370.0, 0.0, 32370.0, 16185.0, 16185.0, 41640.0, 1.0, January, 2014,
Government, Germany, Carretera, None, 1321.0, 3.0, 20.0, 26420.0, 0.0, 26420.0, 13210.0, 13210.0, 41640.0, 1.0, January, 2014,
Midmarket, France, Carretera, None, 2178.0, 3.0, 15.0, 32670.0, 0.0, 32670.0, 21780.0, 10890.0, 41791.0, 6.0, June, 2014,
특정 열의 값 인쇄하기
pandas
패키지 사용
import pandas
df = pandas.read_excel("sample.xls")
column = df.columns[4]
print(column)
print("-" * len(column))
for index, row in df.iterrows():
print(row[column])
출력:
Units Sold
----------
1618.5
1321.0
2178.0
888.0
2470.0
1513.0
921.0
2518.0
1899.0
1545.0
2470.0
2665.5
958.0
2146.0
345.0
615.0
292.0
974.0
2518.0
1006.0
367.0
883.0
549.0
788.0
2472.0
1143.0
1725.0
912.0
2152.0
1817.0
1513.0
1493.0
1804.0
2161.0
1006.0
1545.0
2821.0
345.0
2001.0
2838.0
2178.0
888.0
...
xlrd
패키지 사용
from xlrd import open_workbook
wb = open_workbook("sample.xls")
sheet = wb.sheet_by_index(0)
sheet.cell_value(0, 0)
column_index = 4
column = sheet.cell_value(0, column_index)
print(column)
print("-" * len(column))
for row in range(1, sheet.nrows):
print(sheet.cell_value(row, column_index))
출력:
Units Sold
----------
1618.5
1321.0
2178.0
888.0
2470.0
1513.0
921.0
2518.0
1899.0
1545.0
2470.0
2665.5
958.0
2146.0
345.0
615.0
292.0
974.0
2518.0
1006.0
367.0
883.0
549.0
788.0
2472.0
1143.0
1725.0
912.0
2152.0
1817.0
1513.0
1493.0
1804.0
2161.0
1006.0
1545.0
2821.0
345.0
2001.0
2838.0
2178.0
888.0
...