Python 다중 처리 공유 객체
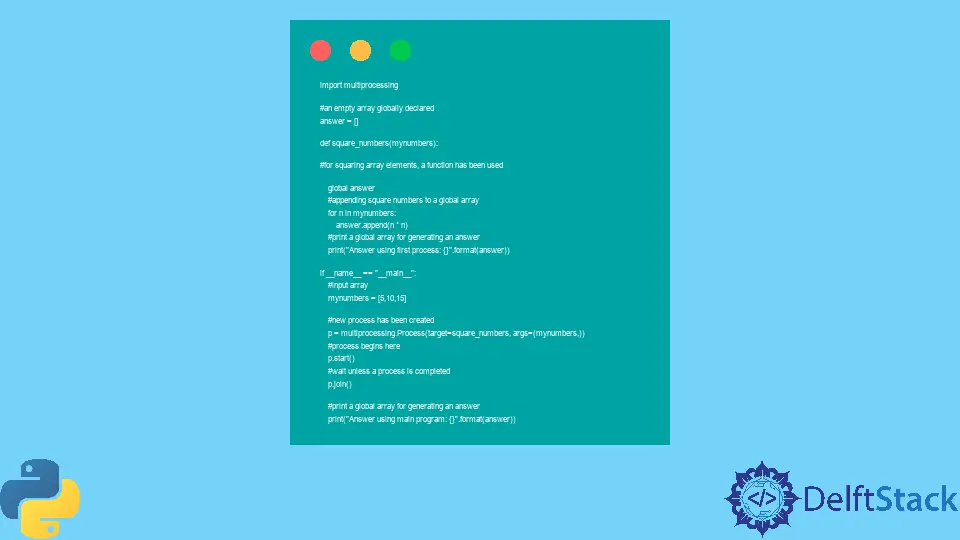
Python에서 공유 메모리 다중 처리는 여러 프로세서 연결로 구성되지만 이러한 프로세서는 시스템의 주 메모리에 직접 액세스할 수 있어야 합니다. 이렇게 하면 연결된 모든 프로세서가 사용하거나 생성한 다른 프로세서 데이터에 액세스할 수 있습니다.
다중 처리에서 Python 공유 메모리 개체 사용
Python에서 다중 처리를 사용하면 새 프로세스가 독립적으로 실행되고 자체 메모리 공간을 가질 수 있습니다. 아래 예제를 보면서 파이썬을 이용한 공유객체 다중처리에 대해 자세히 알아보자.
예제 코드:
import multiprocessing
# an empty array globally declared
answer = []
def square_numbers(mynumbers):
# for squaring array elements, a function has been used
global answer
# appending square numbers to a global array
for n in mynumbers:
answer.append(n * n)
# print a global array for generating an answer
print("Answer using first process: {}".format(answer))
if __name__ == "__main__":
# input array
mynumbers = [5, 10, 15]
# new process has been created
p = multiprocessing.Process(target=square_numbers, args=(mynumbers,))
# process begins here
p.start()
# wait unless a process is completed
p.join()
# print a global array for generating an answer
print("Answer using main program: {}".format(answer))
출력:
Answer using first process: [25, 100, 225]
Answer using main program: []
위의 예를 사용하여 두 위치에 전역 배열 응답을 인쇄했습니다.
프로세스 p
는 메모리 공간에서 프로세스 p
에 대해 배열 요소가 변경되도록 square_numbers
함수라고 합니다.
주 프로그램은 p
프로세스가 완료된 후 실행되며 메모리 공간에서 응답으로 빈 배열을 얻습니다.
Python의 다중 처리는 여러 프로세스 간에 데이터를 공유하기 위한 값 객체와 배열을 제공합니다.
예제 코드:
import multiprocessing
def square_data(mydata, answer, square_sum):
# a function has been made for squaring of given data
# appending squares of mydata to the given array
for ix, n in enumerate(mydata):
answer[ix] = n * n
# sum the square values
square_sum.value = sum(answer)
# print array of squared values for process p
print("Answer in process p: {}".format(answer[:]))
# print the sum of squared values for process p
print("Sum of squares values in process p: {}".format(square_sum.value))
if __name__ == "__main__":
# here, we input the data
mydata = [1, 2, 3]
# an array has been created for the int data type for three integers
answer = multiprocessing.Array("i", 3)
# value has been created for int data type
square_sum = multiprocessing.Value("i")
# new process has been created
p = multiprocessing.Process(target=square_data, args=(mydata, answer, square_sum))
# process begins from here
p.start()
# wait unless the process is completed
p.join()
# print an array of squared values for the main program
print("Answer in main program: {}".format(answer[:]))
# print the sum of squared values for the main program
print("Sum of square values in main program: {}".format(square_sum.value))
출력:
Answer in process p: [1, 4, 9]
Sum of squares in process p: 14
Answer in main program: [1, 4, 9]
Sum of squares in main program: 14
위의 예에서는 배열을 만들고 세 개의 정수를 전달했습니다. 제곱 값의 배열을 인쇄한 다음 p
프로세스에 대한 제곱 값의 합을 인쇄했습니다.
그런 다음 메인 프로그램에 대한 제곱 값의 배열과 제곱 값의 합을 다시 인쇄했습니다.
결론
Python을 사용하여 공유 메모리 다중 처리를 설명하는 방법에는 여러 가지가 있을 수 있습니다. 따라서 이 기사에서는 개체가 공유 메모리 공간에 배치되고 독립적으로 실행되는 방법에 대한 다중 처리 공유 메모리 개념을 설명했습니다.
이 외에도 Python을 사용하면 프로세스가 다양한 프로세스 간에 데이터를 공유할 수 있다는 것도 배웠습니다.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn