PHP 조건문
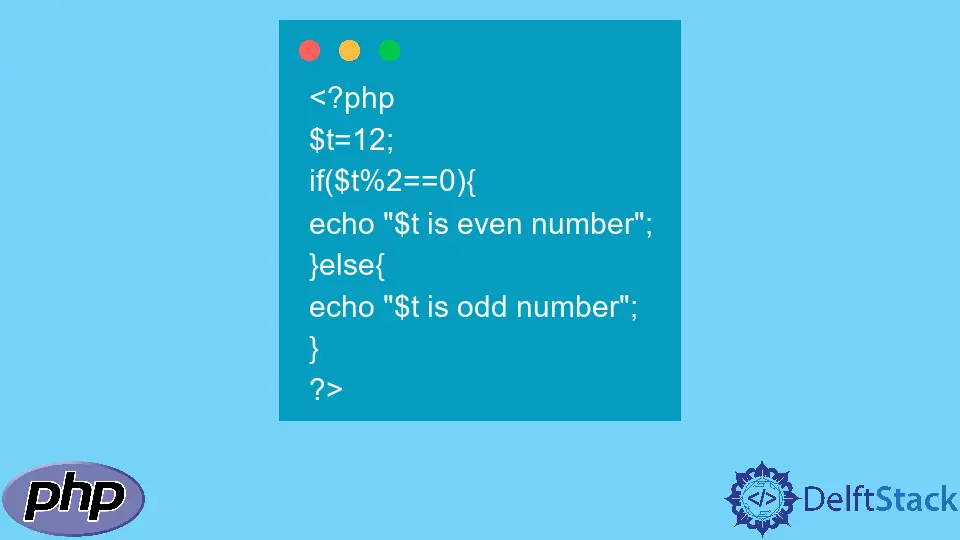
이 기사에서는 PHP에서 사용할 수 있는 다양한 조건문에 대해 설명합니다. 우리는 조건에 따라 PHP가 행동하도록 지시할 수 있습니다.
다음은 PHP에서 사용할 수 있는 조건문 목록입니다.
if
문if...else
문if...elseif..else
문스위치
문
PHP의 if
문
설정된 조건이 true
인 경우 PHP는 if
문에서 코드 블록을 실행합니다.
통사론:
if(condition){
//Code
}
예를 들어 보겠습니다.
<?php
$t=12;
if($t<50){
echo "$t is less than 50";
}
?>
출력:
12 is less than 50
PHP의 if...else
문
if...else
문을 사용하면 설정된 조건이 true
인 경우 코드 블록을 실행하고 조건이 false
인 경우 다른 코드 블록을 실행할 수 있습니다.
통사론:
if(condition){
//Code
}else{
//Code
}
예:
if...else
문을 사용하여 짝수와 홀수를 그룹화할 수 있습니다.
<?php
$t=12;
if($t%2==0){
echo "$t is even number";
}else{
echo "$t is odd number";
}
?>
출력:
12 is even number
PHP의 if...elseif..else
문
코드를 실행할 때 두 가지 이상의 조건을 확인하는 데 사용합니다.
통사론:
if(condition 1){
//Run code if condition is True;
}elseif(condition 2){
//Run Code if condition 1 is False and condition 2 is True;
}else{
//Run code if all conditions are false;
}
실제 예를 살펴보겠습니다.
if...elseif..else
문을 사용하여 등급 시스템을 만들 수 있습니다.
<?php
$m=69;
if ($m<33){
echo "fail";
}
else if ($m>=34 && $m<50) {
echo "D grade";
}
else if ($m>=50 && $m<65) {
echo "C grade";
}
else if ($m>=65 && $m<80) {
echo "B grade";
}
else if ($m>=80 && $m<90) {
echo "A grade";
}
else if ($m>=90 && $m<100) {
echo "A+ grade";
}
else {
echo "Invalid input";
}
?>
출력:
B grade
PHP의 중첩 if
문
중첩된 if
문에는 다른 if
블록 안에 if
블록이 있습니다. 내부 문이 실행되려면 외부 문이 true
여야 합니다.
통사론:
if(condition){
//code
if(condition){
//code
}
}
실제 예를 살펴보겠습니다.
중첩된 문을 사용하여 간단한 투표 자격 테스트를 만들 수 있습니다.
예:
<?php
$a= 23;
$nationality = "Dutch";
//applying conditions on nationality and age
if ($nationality == "Dutch")
{
if ($a >= 18) {
echo "Eligible to vote";
}
else {
echo "Not eligible to vote";
}
}
?>
출력:
Eligible to vote
항상 깊게 중첩하는 것이 권장되는 것은 아닙니다. 논리를 따르기가 어려워집니다.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn