Mysqli_real_escape_string으로 양식 데이터 처리
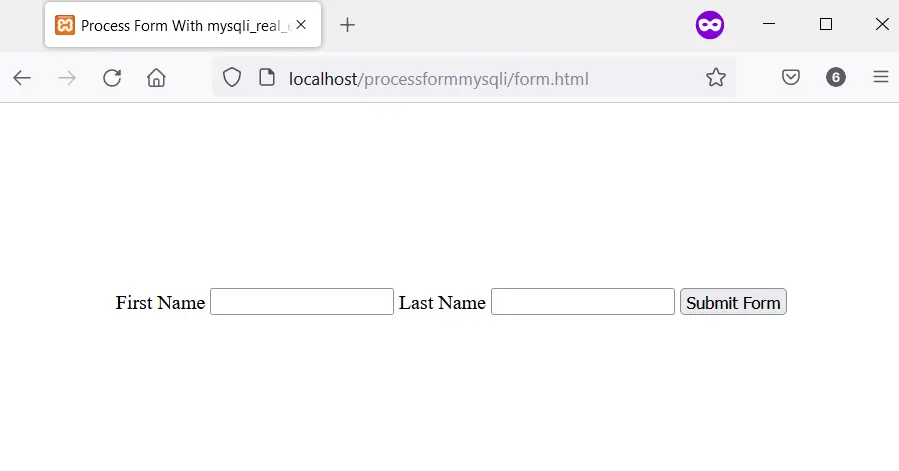
이 기사에서는 mysqli_real_escape_string
을 사용하여 양식 데이터를 처리하는 방법을 설명합니다.
먼저 샘플 데이터베이스와 테이블을 설정합니다. 그런 다음 사용자 입력을 수락하는 HTML 양식을 만듭니다.
이후 PHP에서 에러 없이 mysqli_real_escape_string
을 사용하는 방법을 설명합니다.
로컬 서버 설정
이 기사의 모든 코드는 서버에서 작동합니다. 따라서 라이브 서버에 액세스할 수 있는 경우 이 섹션을 건너뛰고 다음 섹션으로 이동할 수 있습니다.
그렇지 않은 경우 Apache Friends의 XAMPP와 같은 로컬 서버를 설치합니다. XAMPP가 설치되면 htdocs
폴더를 찾아 폴더를 만듭니다.
이 폴더에는 이 기사의 모든 코드가 저장됩니다.
데이터베이스 및 테이블 생성
XAMPP에서 phpMyAdmin
또는 명령줄을 사용하여 데이터베이스를 만들 수 있습니다. 명령줄에 있는 경우 다음 명령을 사용하여 MySQL에 로그인합니다.
#login to mysql
mysql -u root -p
MySQL에 로그인했으면 데이터베이스를 생성합니다. 이 기사에서는 데이터베이스를 my_details
라고 부를 것입니다.
CREATE database my_details
데이터베이스가 생성되면 다음 SQL 코드가 포함된 테이블을 생성합니다. 이 테이블에는 샘플 프로젝트에 대한 데이터가 저장됩니다.
CREATE TABLE bio_data (
id INT NOT NULL AUTO_INCREMENT,
first_name VARCHAR(50) NOT NULL,
last_name VARCHAR(50) NOT NULL,
PRIMARY KEY (id)) ENGINE = InnoDB;
HTML 양식 만들기
HTML 양식에는 두 가지 양식 입력이 있습니다. 첫 번째는 사용자의 이름을 수집하고 두 번째는 성을 수집합니다.
<head>
<meta charset="utf-8">
<title>Process Form With mysqli_real_escape_string</title>
<style>
body {
display: grid;
justify-content: center;
align-items: center;
height: 100vh;
}
</style>
</head>
<body>
<main>
<form action="process_form.php" method="post">
<label id="first_name">First Name</label>
<input id="first_name" type="text" name="first_name" required>
<label id="last_name">Last Name</label>
<input id="last_name" type="text" name="last_name" required>
<input type="submit" name="submit_form" value="Submit Form">
</form>
</main>
</body>
출력:
양식 데이터 처리
양식 처리 중에 mysqli_real_escape_string
을 사용하여 양식 입력을 이스케이프합니다. 게다가 데이터베이스 연결은 mysqli_real_escape_string
의 첫 번째 인수여야 합니다.
그래서 다음의 이름과 성에 mysqli_real_escape_string
을 사용했습니다. 코드를 사용하려면 process_form.php
로 저장하십시오.
<?php
if (isset($_POST['submit_form']) && isset($_POST["first_name"]) && isset($_POST["last_name"])) {
// Set up a database connection.
// Here, our password is empty
$connection_string = new mysqli("localhost", "root", "", "my_details");
// Escape the first name and last name using
// mysqli_real_escape_string function. Meanwhile,
// the first parameter to the function should
// be the database connection. If you omit, the
// database connection, you'll get an error.
$first_name = mysqli_real_escape_string($connection_string, trim(htmlentities($_POST['first_name'])));
$last_name = mysqli_real_escape_string($connection_string, trim(htmlentities($_POST['last_name'])));
// If there is a connection error, notify
// the user, and Kill the script.
if ($connection_string->connect_error) {
echo "Failed to connect to Database. Please, check your connection details.";
exit();
}
// Check string length, empty strings and
// non-alphanumeric characters.
if ( $first_name === "" || !ctype_alnum($first_name) ||
strlen($first_name) <= 3
) {
echo "Your first name is invalid.";
exit();
}
if ( $last_name === "" || !ctype_alnum($last_name) ||
strlen($last_name) < 2
) {
echo "Your last name is invalid.";
exit();
}
// Insert the record into the database
$query = "INSERT into bio_data (first_name, last_name) VALUES ('$first_name', '$last_name')";
$stmt = $connection_string->prepare($query);
$stmt->execute();
if ($stmt->affected_rows === 1) {
echo "Data inserted successfully";
}
} else {
// User manipulated the HTML form or accessed
// the script directly. Kill the script.
echo "An unexpected error occurred. Please, try again later.";
exit();
}
?>
출력(처리가 성공한 경우):
Mysqli_real_escape_string
오류의 원인
mysqli_real_escape_string
에서 데이터베이스 연결을 생략하면 오류가 발생합니다. 따라서 다음 코드에서 process_form.php
를 수정했습니다.
한편, 이 버전은 mysqli_real_escape_string
에 데이터베이스 연결이 없습니다. 결과적으로 데이터베이스에 데이터를 삽입하려고 할 때 오류가 발생합니다.
<?php
if (isset($_POST['submit_form']) && isset($_POST["first_name"]) && isset($_POST["last_name"])) {
$connection_string = new mysqli("localhost", "root", "", "my_details");
// We've omitted the connection string
$first_name = mysqli_real_escape_string(trim(htmlentities($_POST['first_name'])));
$last_name = mysqli_real_escape_string(trim(htmlentities($_POST['last_name'])));
if ($connection_string->connect_error) {
echo "Failed to connect to Database. Please, check your connection details.";
exit();
}
if ( $first_name === "" || !ctype_alnum($first_name) ||
strlen($first_name) <= 3
) {
echo "Your first name is invalid.";
exit();
}
if ( $last_name === "" || !ctype_alnum($last_name) ||
strlen($last_name) < 2
) {
echo "Your last name is invalid.";
exit();
}
$query = "INSERT into bio_data (first_name, last_name) VALUES ('$first_name', '$last_name')";
$stmt = $connection_string->prepare($query);
$stmt->execute();
if ($stmt->affected_rows === 1) {
echo "Data inserted successfully";
}
} else {
echo "An unexpected error occurred. Please, try again later.";
exit();
}
?>
샘플 오류 메시지:
Fatal error: Uncaught ArgumentCountError: mysqli_real_escape_string() expects exactly 2 arguments, 1 given in C:\xampp\htdocs\processformmysqli\process_form.php:12 Stack trace: #0 C:\xampp\htdocs\processformmysqli\process_form.php(12): mysqli_real_escape_string('Johnson') #1 {main} thrown in C:\xampp\htdocs\processformmysqli\process_form.php on line 12
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn