jQuery를 이 키워드
-
DOM 요소로서의 jQuery
this
키워드 -
콜백 내 DOM 요소로 jQuery
this
키워드 사용 -
each()
메서드의 콜백 내에서 jQuerythis
키워드를 DOM 요소로 사용 -
AJAX
load()
메서드에서 jQuerythis
키워드를 DOM 요소로 사용 -
filter()
메서드에 대한 콜백에서 jQuerythis
키워드를 DOM 요소로 사용 -
jQuery
this
키워드를 일반 개체로 사용 -
플러그인 내부에서 jQuery
this
키워드를 jQuery 개체로 사용
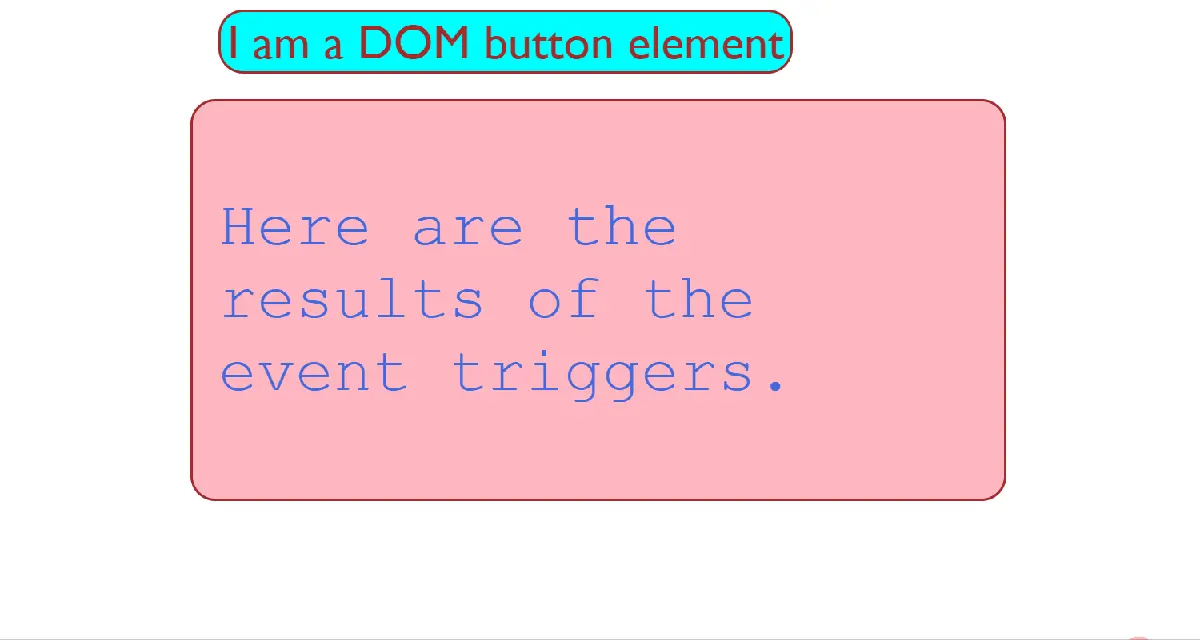
따라서 jQuery this
키워드를 사용하면 개발자가 새로운 검색이나 조회 없이 현재 개체의 메서드와 속성을 사용할 수 있습니다. 이 자습서는 다양한 컨텍스트에서 this
키워드의 여러 사용 사례를 보여줍니다.
DOM 요소로서의 jQuery this
키워드
jQuery this
키워드의 가장 일반적인 사용은 DOM 요소를 참조할 때입니다. 특별히 jQuery 플러그인을 만들지 않는 경우 이 사용 사례를 사용할 가능성이 높다는 경험 법칙을 가질 수도 있습니다.
jQuery this
키워드는 처음에는 혼란스러울 수 있습니다. jQuery(및 기타 프로그래밍 언어)는 this
키워드를 유용한 도구로 제공하며 대부분의 경우 jQuery this
키워드의 값은 쉽게 파악할 수 있습니다. 참조해야 합니다.
콜백 내 DOM 요소로 jQuery this
키워드 사용
콜백 내부의 코드가 참조해야 할 가능성이 가장 높은 엔터티는 콜백이 수신 대기하는 DOM 요소입니다. 따라서 jQuery는 이 경우 this
키워드를 호출 DOM 요소로 설정합니다. (엄지 규칙이 꽤 잘 작동하는 것을 볼 수 있습니다.)
코드 - JavaScript + jQuery:
// this as a jQuery DOM button element inside a callback
$("#DOM_button").on("click",function(){
$("p").html(`The value of 'this' keyword inside the DOM button element is ${$(this).attr('id')}`);
//use case - you can deactivate the button easily without another DOM search call
$(this).prop("disabled",true);
});
코드 - HTML:
<body>
<div id="triggers">
<!-- Button DOM element -->
<button id="DOM_button">I am a DOM button element</button>
</div>
</body>
우리의 코드는 DOM_button
요소에서 수신하는 click
이벤트 핸들러에 대한 콜백 내부의 this
키워드 값을 기록합니다. 이벤트 핸들러가 수신하는 DOM 요소의 일부 속성을 변경할 수 있습니다.
예를 들어 DOM_button
요소를 비활성화합니다. 이것은 이 컨텍스트에서 this
키워드의 일반적인 사용 사례입니다.
출력:
each()
메서드의 콜백 내에서 jQuery this
키워드를 DOM 요소로 사용
each()
메서드를 사용하여 jQuery 컬렉션을 반복할 때 콜백 내부의 각 DOM 요소에 액세스하고 싶을 것입니다. jQuery는 each
메서드 콜백 내에서 this
키워드를 현재 DOM 요소로 설정합니다.
코드 - JavaScript + jQuery:
//this as each DOM element inside a jQuery collection
$("#div_button").on("click",function(){
$("p").html(" ");
$("#div_container>div").each(function(idx){
$("p").append(`The value of 'this' keyword inside child div ${idx + 1} is ${$(this).attr('id')} <br>`);
});
});
코드 - HTML:
<body>
<div id="div_container">
<button id="div_button">
"each" Method Demo
</button>
<div id="first_div">
I am the First Div <br>
</div>
<div id="second_div">
I am the Second Div <br>
</div>
<div id="third_div">
I am the Third Div <br>
</div>
</div>
</body>
출력:
jQuery는 각 반복에서 현재 div
요소에 this
키워드를 할당합니다.
AJAX load()
메서드에서 jQuery this
키워드를 DOM 요소로 사용
jQuery에서 load()
메서드를 사용하여 AJAX 호출을 수행하면 load()
메서드를 실행하는 DOM 요소에 대한 콜백 내부의 this
키워드를 가리킵니다.
다시 말하지만, jQuery this
키워드의 값은 경험 법칙 없이 일직선상에 있습니다. 대부분의 경우 this
키워드는 자연스럽게 액세스해야 하는 개체, 클래스 또는 엔터티를 가리킵니다.
코드 - JavaScript + jQuery:
// //ajax request with the load method
$("#ajax_btn").on("click",function(){
$("#ajax_this").load("http://127.0.0.1:5500/code_files/test.html",
function(){
$("p").html(`The value of 'this' keyword inside the 'load' method to send the AJAX request is ${$(this).attr('id')}`);
//use case - you want to style it when a successful data fetch completes
$(this).css("color","green");
})
});
동일한 서버의 test.html
파일에서 데이터를 가져오기 위해 jQuery AJAX 로드
메서드를 실행합니다. AJAX 요청이 완료될 때 실행되는 load()
메서드에 대한 콜백 내부의 this
키워드 값을 출력합니다.
코드 - HTML:
<div id="ajax_this">
<button id="ajax_btn">
AJAX Call Demo
</button><br>
<br>
<div id="ajax_text">
I demo the use of this as DOM element with an AJAX call
</div>
</div>
출력:
일반적인 사용 사례는 성공적인 AJAX 호출에서 출력을 녹색으로 코딩하려는 경우입니다. 예를 들어 위의 출력 데모에서 출력을 녹색으로 표시합니다.
이 예제에서는 동일한 서버에서 test.html 리소스에 대한 AJAX 호출을 수행했습니다.
코드 - test.html
:
<title>Test HTML Page</title>
</head>
<body>
<div id="main">
<div id="top">
<h3>
We dish out a test page for our AJAX request on localhost.
</h3>
</div>
<div id="mid">
<h3>
The request completes and returns the data that displays on the page.
</h3>
</div>
</div>
</body>
</html>
filter()
메서드에 대한 콜백에서 jQuery this
키워드를 DOM 요소로 사용
jQuery 컬렉션에서 filter()
메서드를 사용하여 하위 집합을 선택할 때 각 반복에서 컬렉션의 현재 DOM 요소에 빠르게 액세스하려고 합니다. jQuery는 현재 요소에 대한 filter
메서드에 대한 콜백 내에서 this
키워드를 설정합니다.
this
키워드는 우리가 가장 직관적으로 예상하는 개체를 나타냅니다.
코드 - JavaScript + jQuery:
//this as the current DOM element inside the filter function in each iteration
$("#filter_this").on("click",function(){
let $filterSet = $("div").filter(function(){
return $(this).attr("id") == "selection_filter_this";
});
$("p").html(`The filtered set of div elements with the use of 'this' keyword are those with ID = : ${$filterSet.first().attr('id')}`);
});
코드 - HTML:
<body>
<div id="selection_filter_this">
<div id="filter_text">
I demo the use of this as DOM element with a selector filter
</div>
<button id="filter_this">
This keyword with the filter method
</button>
</div>
</body>
출력:
우리는 div
요소를 필터링하여 콜백에서 this
키워드와 selection_filter_this
ID와 일치하는 항목만 선택하는 것을 볼 수 있습니다. 그런 다음 필터링된 요소의 ID를 표시합니다.
jQuery this
키워드를 일반 개체로 사용
each()
메서드와 같은 jQuery 메서드는 배열과 같은 객체를 반복할 수 있습니다. DOM 요소에 대한 반복에만 국한되지 않습니다.
each()
와 같은 jQuery 메서드를 사용하여 배열과 같은 구조를 반복하는 경우 당연히 반복에서 현재 엔터티에 액세스해야 합니다. 따라서 jQuery는 이러한 상황에서 this
키워드를 현재 객체로 설정합니다.
코드 - JavaScript + jQuery:
// this as the current object in each iteration of the 'each' method
let objEachArr = ["First","Second","Third"];
$('#object_each_btn').on("click",function(){
$("p").html("");
$.each(objEachArr,function(curr){
$("p").append(`The value of 'this' keyword in the current iteration of the 'each' method is ${this} <br><br>`);
});
});
코드 - HTML:
<body>
<div id="object_each_div">
<div id="object_each_text">
I demo the use of 'this' as the current object in every iteration of the 'each' method.
</div>
<button id="object_each_btn">
'This' in 'each' Method
</button>
</div>
<div id="results">
<p>
We will display the results here.
</p>
</div>
</body>
배열 objEachArr
에 대한 each
메서드의 각 반복에서 this
키워드의 값을 출력합니다.
출력:
this
키워드는 each()
메서드의 각 반복에서 현재 배열 요소를 참조합니다.
플러그인 내부에서 jQuery this
키워드를 jQuery 개체로 사용
jQuery this
키워드에 대한 특별한 경우가 하나 있습니다. 플러그인 내에서 this
키워드를 사용하면 jQuery
개체를 참조합니다.
코드 - JavaScript + jQuery:
/this as the jQuery Object inside a jQuery Plug-In
$.fn.plugInDemo = function(){
this.html(`The 'this' keyword inside my plug-in is the jQuery Object and we output the jQuery Version
from this jQuery Object : ${this.jquery}`);
return this;
};
$("#plugin_btn").on("click",function(){
$("p").plugInDemo();
});
코드 - HTML:
<body>
<div id="plugin_div">
<div id="plugin_text">
I demo the use of 'this' inside a jQuery Plugin.
</div>
<button id="plugin_btn">
'This' inside a Plug-In
</button>
</div>
<div id="results">
<p>
We will display the results here.
</p>
</div>
</body>
간단한 플러그인 함수인 plugInDemo
내에서 this
키워드를 통해 jQuery
개체에 액세스하여 jQuery 버전을 출력합니다.
출력:
jQuery this
키워드는 새로운 DOM 조회 없이 현재 개체, 클래스 또는 엔터티에 빠르게 액세스할 수 있는 강력한 도구이며 앱 성능을 향상시키는 훌륭한 방법입니다.