JavaScript의 문자열에서 부분 문자열 제거
Kirill Ibrahim
2023년1월30일
JavaScript
JavaScript String
-
문자열에서 특정 하위 문자열을 제거하는 JavaScript
replace()
메서드 -
문자열에서 특정 하위 문자열의 모든 발생을 제거하기 위해 Regex를 사용하는 JavaScript
replace()
메서드 -
문자열에서 특정 하위 문자열을 추출하는 JavaScript
substr()
메서드
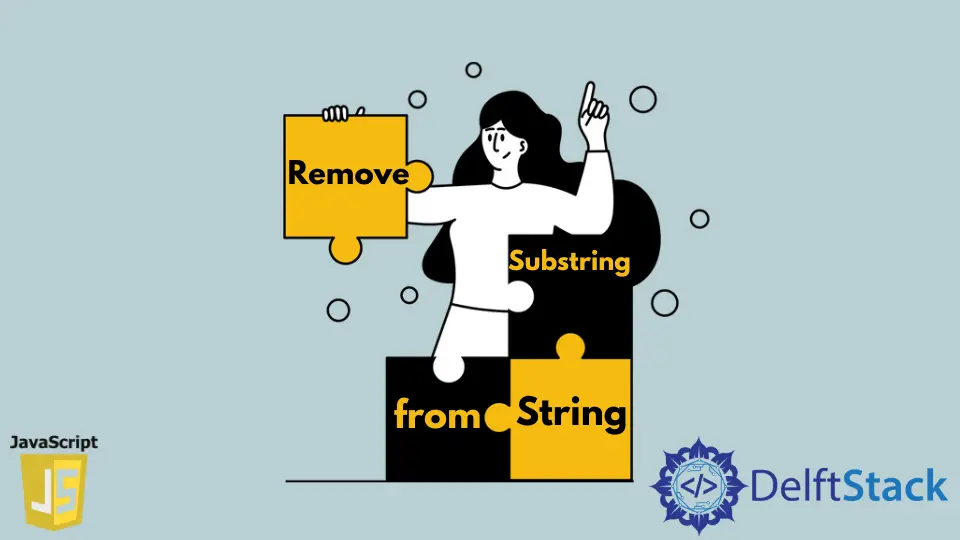
JavaScript에는 문자열에서 하위 문자열을 제거하는 두 가지 인기있는 방법이 있습니다. 아래의 모든 메서드에는 컴퓨터에서 실행할 수있는 코드 예제가 있습니다.
문자열에서 특정 하위 문자열을 제거하는 JavaScript replace()
메서드
replace()
함수는 Java 스크립트의 내장 함수입니다. 주어진 문자열의 일부를 다른 문자열이나 정규식으로 바꿉니다. 주어진 문자열에서 새 문자열을 반환하고 원래 문자열을 변경하지 않습니다.
replace()
구문:
Ourstring.replace(Specificvalue, Newvalue)
Specificvalue
는 새 값인Newvalue
로 대체됩니다.
JavaScript replace()
메서드 예 :
<!DOCTYPE html>
<html>
<head>
<title>
How to remove a substring from string in JavaScript?
</title>
</head>
<body>
<h1>
DelftStack
</h1>
<p>Our string is DelftStac for Software</p>
<p>
Our New String is: <span class="output"></span>
</p>
<button onclick="removeText()">
Generate Text
</button>
<script type="text/javascript">
function removeText() {
ourWord = 'DelftStac for Software';
ourNewWord = ourWord.replace('DelftStack', '');
document.querySelector('.output').textContent
= ourNewWord;
}
</script>
</body>
</html>
문자열에서 특정 하위 문자열의 모든 발생을 제거하기 위해 Regex를 사용하는 JavaScript replace()
메서드
전역 속성과 함께 Specificvalue
대신 정규 표현식이 사용됩니다.
예:
<!DOCTYPE html>
<html>
<head>
<title>
How to remove to remove all occurrences of the specific substring from string in JavaScript?
</title>
</head>
<body>
<h1>
DelftStack
</h1>
<p>Our string is DelftStackforDelftStack</p>
<p>
Our New String is: <span class="output"></span>
</p>
<button onclick="removeText()">
Generate Text
</button>
<script type="text/javascript">
function removeText() {
ourWord = 'DelftStackforDelftStack';
ourNewWord = ourWord.replace(/DelftStack/g, '');
document.querySelector('.output').textContent
= ourNewWord;
}
</script>
</body>
</html>
문자열에서 특정 하위 문자열을 추출하는 JavaScript substr()
메서드
substr()
함수는 주어진 문자열에서 하위 문자열을 추출하거나 문자열의 일부를 반환하는 JavaScript의 내장 함수입니다. 지정된 인덱스에서 시작하여 주어진 문자 수만큼 확장됩니다.
substr()
구문:
string.substr(startIndex, length)
startIndex
가 필요합니다. length
는 선택 사항이며 해당Startindex
에서 선택할 문자열의 길이이며 지정되지 않은 경우 나머지 문자열로 추출됩니다.
예:
<!DOCTYPE html>
<html>
<head>
<title>
How to remove a substring from string in JavaScript?
</title>
</head>
<body>
<h1>
DelftStack
</h1>
<p>Our string is DelftStackforDelftStack</p>
<p>
Our New String is: <span class="output"></span>
</p>
<button onclick="removeText()">
Generate Text
</button>
<script type="text/javascript">
function removeText() {
ourWord = 'DelftStackforDelftStack';
ourNewWord = ourWord.substr(10,13);
document.querySelector('.output').textContent
= ourNewWord;
}
</script>
</body>
</html>
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다