Java의 스레드로부터 안전한 컬렉션
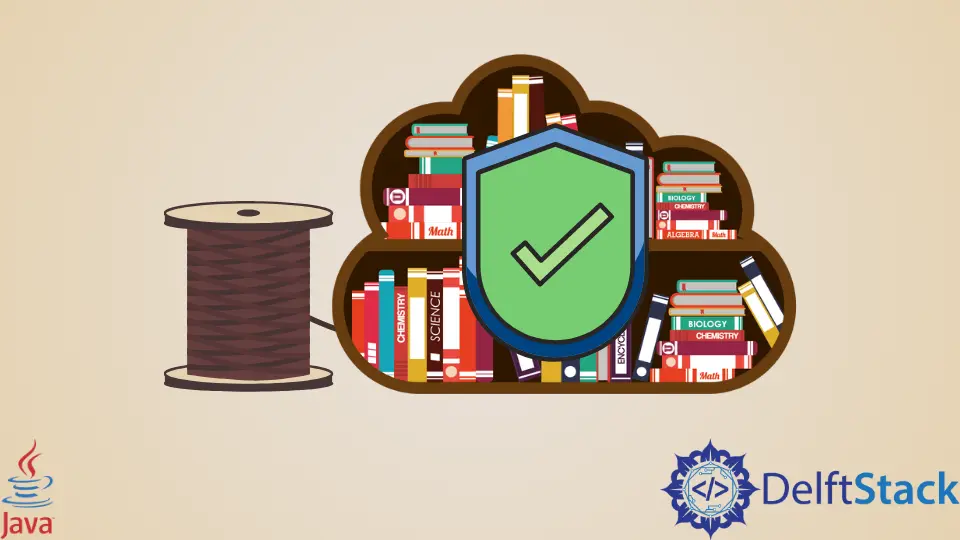
Java에서 스레드 안전
은 클래스의 내부 상태를 보장하고 여러 스레드가 동시에 호출되는 동안 메소드의 값이 정확함을 보장하는 클래스입니다.
이 자습서에서는 Java에서 스레드로부터 안전한
코드 예제를 통해 다양한 컬렉션 클래스에 대해 설명합니다.
Java의 스레드 안전 컬렉션
Java에서 가장 인기 있는 thread-safe
컬렉션 클래스는 Vector
, Stack
, Hashtable
, Properties
등입니다. 아래의 Vector
부터 시작하여 각각 알아봅시다.
스레드 안전 컬렉션 클래스 - 벡터
벡터
는 필요에 따라 커질 수 있는 객체의 배열입니다. 이 클래스는 add()
, remove()
, get()
, elementAt()
및 size()
와 같은 메서드를 지원합니다.
예제 코드:
// Importing necessary packages
import java.util.*;
public class JavaVector {
public static void main(String[] arg) {
// Declaring a vector
Vector MyVector = new Vector();
// Adding data to vector
MyVector.add(12);
MyVector.add(3);
MyVector.add("ABC");
MyVector.add(10);
MyVector.add("DEF");
System.out.println("The vector is: " + MyVector);
// Removing data from the vector.
MyVector.remove(1);
System.out.println("The vector after removing an element is: " + MyVector);
}
}
출력:
The vector is: [12, 3, ABC, 10, DEF]
The vector after removing an element is: [12, ABC, 10, DEF]
스레드 안전 컬렉션 클래스 - Stack
Stack
클래스는 스택 데이터 구조를 구현합니다. 그것은 LIFO
또는 Last In First Out
을 기반으로 합니다. Stack
클래스의 가장 일반적인 작업은 push()
, pop()
, empty()
및 search()
입니다.
예제 코드:
// Importing necessary packages
import java.util.*;
public class JavaStack {
public static void main(String[] args) {
// Declaring a stack
Stack<Integer> MyStk = new Stack<Integer>();
// Adding data to stack
MyStk.push(5);
MyStk.push(7);
MyStk.push(9);
// POP from the stack
Integer num1 = (Integer) MyStk.pop();
System.out.println("The Popped element is: " + num1);
// PUSH the stack
Integer num2 = (Integer) MyStk.peek();
System.out.println("The top element is: " + num2);
}
}
출력:
The popped element is: 9
The top element is: 7
스레드 안전 컬렉션 클래스 - Hashtable
Java Hashtable
클래스는 keys
를 values
에 매핑하는 해시 테이블을 구현합니다. 또한 Map
인터페이스를 구현하고 디렉토리를 상속합니다.
예제 코드:
// Importing necessary packages
import java.util.*;
public class JavaHashtable {
public static void main(String args[]) {
// Declaring a HashTable
Hashtable<Integer, String> HTable = new Hashtable<Integer, String>();
// Adding data to HashTable
HTable.put(100, "This");
HTable.put(102, "is");
HTable.put(101, "a");
HTable.put(103, "data");
for (Map.Entry MyMap : HTable.entrySet()) {
// Extracting data and keys from the HashTable
System.out.println(MyMap.getKey() + " " + MyMap.getValue());
}
}
}
출력:
103 data
102 is
101 a
100 This
스레드 안전 컬렉션 클래스 - HashMap 동기화
Java에서 스레드 안전
컬렉션으로 사용할 수 있는 HashMap
의 동기화 버전이 있습니다. 이 HashMap
은 Synchronize HashMap
과 가장 유사하게 작동합니다.
예제 코드:
// Importing necessary packages
import java.util.*;
public class JavaHashMap {
public static void main(String[] args) throws Exception {
try {
// Declaring a HashMap
Map<String, String> HMap = new HashMap<String, String>();
// Adding values to the HashMap
HMap.put("1", "This");
HMap.put("2", "is");
HMap.put("3", "a");
HMap.put("4", "sample");
HMap.put("5", "data");
// Printing the value of HashMap
System.out.println("The Map is : " + HMap);
// Synchronizing the HashMap
Map<String, String> SMap = Collections.synchronizedMap(HMap);
// Printing the Collection after synchronizing the HashMap
System.out.println("The synchronized map is : " + SMap);
} catch (IllegalArgumentException e) {
// Printing exception if necessary
System.out.println("Exception thrown : " + e);
}
}
}
출력:
The Map is : {1=This, 2=is, 3=a, 4=sample, 5=data}
The synchronized map is : {1=This, 2=is, 3=a, 4=sample, 5=data}
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn