Java에서 HTML 구문 분석
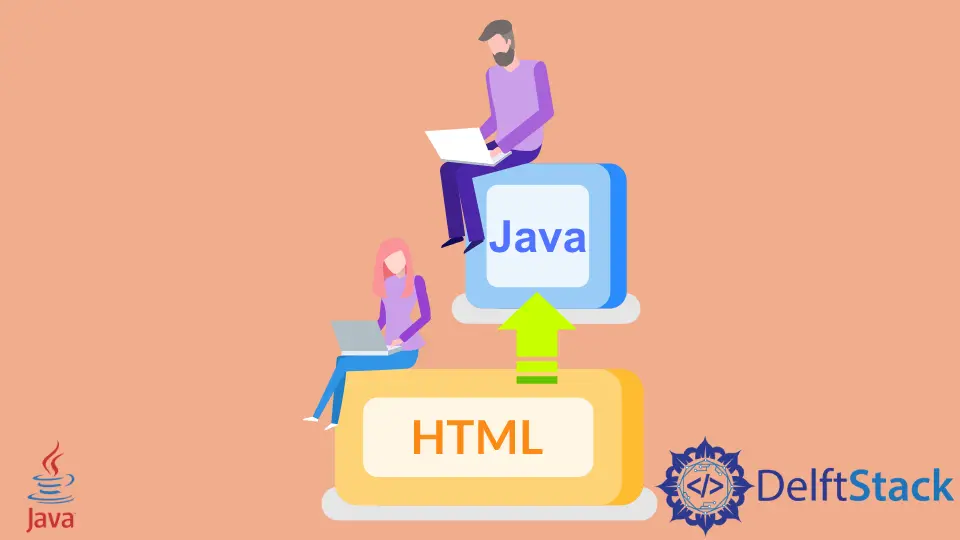
HTML 파일로 작업하는 프로그램에서 작업하는 경우 HTML 파일을 효율적으로 구문 분석하는 방법을 찾아야 할 수 있습니다. 가장 많이 사용되는 웹 스크래핑 도구 Jsoup
를 사용하여 Java 프로그래밍 언어를 통해 HTML 파일을 빠르게 구문 분석할 수 있습니다.
이 문서에서는 HTML 파일을 구문 분석하는 방법에 대해 설명합니다. 또한 주제를 쉽게 이해할 수 있도록 필요한 예와 설명을 제공하여 주제에 대해 논의합니다.
Java에서 Jsoup
작업
Jsoup
은 웹 페이지의 HTML 파일을 구문 분석한 다음 Document
개체로 변환하여 작동합니다. 이것은 DOM
의 프로그래밍 방식 표현이라고 말할 수 있습니다.
Jsoup
의 parse
라는 메소드는 Document
를 생성합니다. 아래에서 Jsoup
의 일부 기능에 대해 설명했습니다.
parse(File MyFile, @Nullable String charsetName)
- HTML 파일을 구문 분석하는 데 사용됩니다.parse(InputStream in, @Nullable String CharsetName, String BaseUri)
-InputStream
을 읽고 구문 분석합니다.parse(String html)
- HTML 문자열을 구문 분석하는 데 사용됩니다.
Jsoup
을 사용하여 Java에서 HTML 구문 분석
아래 예제는 Jsoup
를 사용하여 웹사이트를 구문 분석합니다. 예제의 Java 코드는 다음과 같습니다.
// importing necessary packages
package javaparsehtml;
import java.io.IOException;
import java.io.InputStream;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
public class JavaParseHtml {
public static void main(String[] args) {
URL MyUrl;
try {
// Providing the URL of the website
MyUrl = new URL("https://www.example.com");
HttpURLConnection MyConnection;
try {
// Create an Http connection
MyConnection = (HttpURLConnection) MyUrl.openConnection();
// Defining the request format
MyConnection.setRequestProperty("accept", "application/json");
try {
// Create a response stream
InputStream ResponseStream = MyConnection.getInputStream();
// Parsing the website
Document MyDoc = Jsoup.parse(ResponseStream, "UTF-8", "https://www.example.com");
// Showing the output as HTML
System.out.println(MyDoc.html());
} catch (IOException e) {
e.printStackTrace();
}
} catch (IOException e) {
e.printStackTrace();
}
} catch (MalformedURLException e) {
e.printStackTrace();
}
}
}
위의 예에서 HTML 파일을 구문 분석하는 방법을 설명하고 이미 각 행의 목적을 명령했습니다.
예제에서는 제공된 URL을 기반으로 HTTP 연결을 만든 다음 요청된 속성을 정의했습니다. 그런 다음 InputStream
을 만들고 웹 사이트를 구문 분석했습니다.
마지막으로 웹 사이트를 출력으로 인쇄합니다. 위의 Java 프로그램을 실행하면 아래와 같은 출력이 표시됩니다.
<!doctype html>
<html>
<head>
<title>Example Domain</title>
<meta charset="utf-8">
<meta http-equiv="Content-type" content="text/html; charset=utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<style type="text/css">
body {
background-color: #f0f0f2;
margin: 0;
padding: 0;
font-family: -apple-system, system-ui, BlinkMacSystemFont, "Segoe UI", "Open Sans", "Helvetica Neue", Helvetica, Arial, sans-serif;
}
div {
width: 600px;
margin: 5em auto;
padding: 2em;
background-color: #fdfdff;
border-radius: 0.5em;
box-shadow: 2px 3px 7px 2px rgba(0,0,0,0.02);
}
a:link, a:visited {
color: #38488f;
text-decoration: none;
}
@media (max-width: 700px) {
div {
margin: 0 auto;
width: auto;
}
}
</style>
</head>
<body>
<div>
<h1>Example Domain</h1>
<p>This domain is for use in illustrative examples in documents. You may use this domain in literature without prior coordination or asking for permission.</p>
<p><a href="https://www.iana.org/domains/example">More information...</a></p>
</div>
</body>
</html>
여기서 중요한 참고 사항은 Jsoup
의 jar
파일을 설치하거나 포함하지 않은 경우 먼저 프로젝트 디렉토리에 jar
파일을 포함하거나 패키지를 설치해야 합니다. 그렇지 않으면 오류가 발생할 수 있습니다.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn