Java에서 목록을 배열로 변환하는 방법
Hassan Saeed
2023년10월12일
Java
Java Array
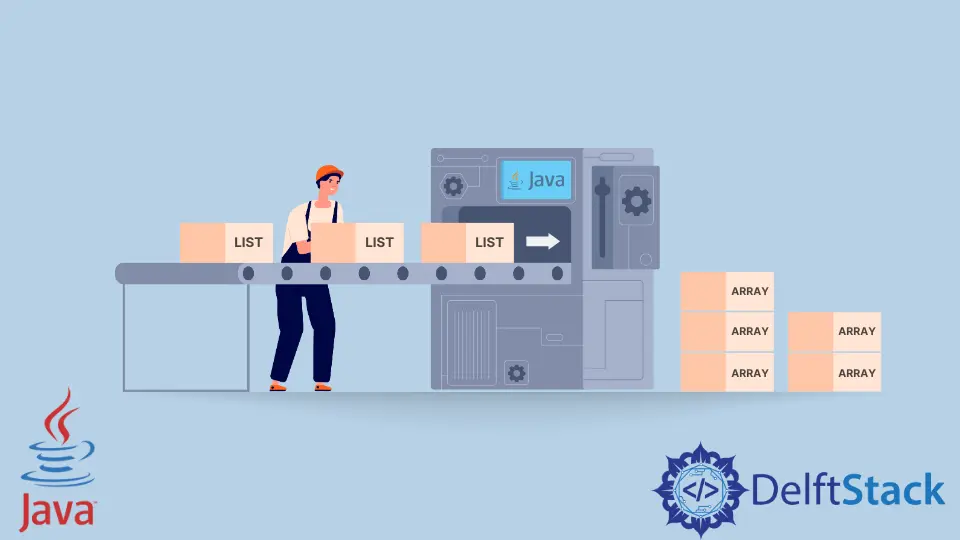
이 자습서에서는 Java에서 목록을 배열로 변환하는 방법에 대해 설명합니다.
목록을 Java의 배열로 변환
이 메서드는 목록과 동일한 크기의 새 배열을 만들고 목록을 반복하여 배열을 요소로 채 웁니다. 아래 예는이를 설명합니다.
import java.util.*;
public class MyClass {
public static void main(String args[]) {
List<Integer> list = new ArrayList();
list.add(1);
list.add(2);
int[] array = new int[list.size()];
for (int i = 0; i < list.size(); i++) array[i] = list.get(i);
}
}
결과 배열에는 목록의 모든 요소가 포함됩니다. 결과 배열이 기본 형식이 아닌 경우이 메서드를 사용해서는 안됩니다.
toArray()
를 사용하여 Java에서 목록을 참조 유형 배열로 변환
이 메서드는 목록에 클래스 개체와 같은 참조 유형의 요소가 포함 된 경우에 사용됩니다. 내장 메소드toArray()
를 사용하여 이러한 유형의 목록을 배열로 변환 할 수 있습니다. 아래 예는이를 설명합니다.
import java.util.*;
public class MyClass {
public static void main(String args[]) {
// Define the Foo class
class Foo {
private int value;
public Foo(int value) {
this.value = value;
}
public int getValue() {
return value;
}
}
// Create instances of Foo
Foo obj1 = new Foo(42);
Foo obj2 = new Foo(99);
// Create a List of Foo objects
List<Foo> list = new ArrayList<>();
// Add the obj1 and obj2 to the list
list.add(obj1);
list.add(obj2);
// Convert the list to an array
Foo[] array = list.toArray(new Foo[0]);
// Print the values from the array
for (Foo foo : array) {
System.out.println("Value: " + foo.getValue());
}
}
}
stream()
을 사용하여 Java에서 목록을 배열로 변환
Java 8 이상에서는 Stream API의stream()
메서드를 사용하여 Java에서 목록을 배열로 변환 할 수도 있습니다. 아래 예는이를 설명합니다.
import java.util.*;
public class MyClass {
public static void main(String args[]) {
List<Integer> list = new ArrayList<>();
list.add(1);
list.add(2);
Integer[] integers = list.stream().toArray(Integer[] ::new);
// Print the converted array
System.out.println(Arrays.toString(integers));
}
}
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다