java.io.IOException 수정: 스트림 종료 오류
-
java.io.IOException: Stream closed
오류가 발생하는 이유 -
새 스트림을 생성하여
java.io.IOException: Stream closed
오류 수정 -
close()
를writeToFile()
외부로 이동하여java.io.IOException: Stream closed
오류 수정
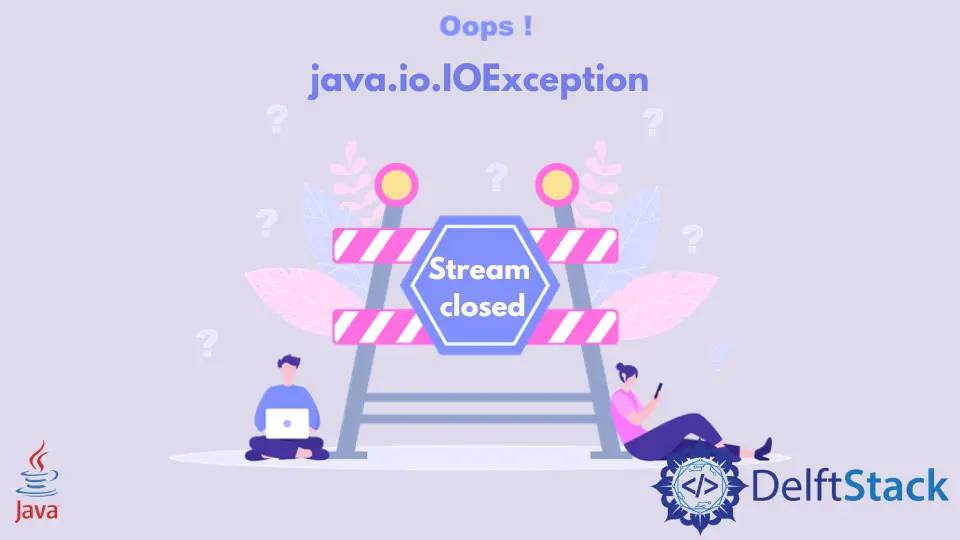
오늘은 Java 프로그래밍에서 코딩 중 java.io.IOException: Stream closed
오류가 발생하는 원인에 대해 알아보도록 하겠습니다. 또한 코드 예제를 통해 이 오류를 수정할 수 있는 두 가지 가능한 솔루션을 탐색합니다.
java.io.IOException: Stream closed
오류가 발생하는 이유
예제 코드(오류 발생):
// import libraries
import java.io.FileWriter;
import java.io.IOException;
// Test Class
public class Test {
// this method writes the given data into the specified file
// and closes the stream
static void writeToFile(
String greetings, String firstName, String lastName, FileWriter fileWriter) {
String customizedGreetings = greetings + "! " + firstName + " " + lastName;
try {
fileWriter.write(customizedGreetings + "\n");
fileWriter.flush();
fileWriter.close();
} catch (IOException exception) {
exception.printStackTrace();
}
} // end writeToFile() method
// main() method
public static void main(String[] args) throws IOException {
// creates a file in append mode and keeps it open
FileWriter fileWriter = new FileWriter("Files/file.txt", true);
// writeToFile() is called to write data into the file.txt
writeToFile("Hi", "Mehvish", "Ashiq", fileWriter);
writeToFile("Hello", "Tahir", "Raza", fileWriter);
} // end main()
} // end Test class
java.io.IOException: Stream closed
오류가 발생하는 이유를 찾기 위해 코드를 이해해 봅시다. 그런 다음 솔루션으로 이동합니다.
이 코드 스니펫은 java.io
패키지에 있는 FileWriter
클래스를 사용하며 지정된 파일에 문자
형식으로 데이터를 쓰는 데 사용됩니다. 지정된 위치에 파일이 없으면 지정된 파일을 생성하고 열어 둡니다.
파일이 이미 있으면 FileWriter
가 파일을 대체합니다.
main()
메서드 내에서 FileWriter
생성자를 호출하여 append
모드에서 지정된 파일을 생성한 다음 writeToFile()
메서드를 두 번 호출하여 주어진 데이터를 file.txt
파일에 씁니다. .
첫 번째 호출에서 writeToFile()
메서드는 데이터를 file.txt
에 쓰고 FileWriter
의 데이터를 플러시한 다음 닫습니다. close()
메서드를 호출하여 스트림을 닫았다는 점에 유의하십시오.
두 번째 호출에서 FileWriter
개체는 스트림이 닫혀 있기 때문에 쓰기로 되어 있는 파일을 찾을 수 없습니다. 따라서 writeToFile()
메서드에 대한 두 번째 호출로 인해 이 오류가 발생합니다.
이 오류를 수정하는 두 가지 해결책이 있습니다. 둘 다 코드 샘플과 함께 아래에 제공됩니다.
새 스트림을 생성하여 java.io.IOException: Stream closed
오류 수정
첫 번째 솔루션은 FileWriter
개체를 writeToFile()
함수로 이동하여 지정된 파일에 쓰려고 할 때마다 새 스트림을 만드는 것입니다.
예제 코드:
// import libraries
import java.io.FileWriter;
import java.io.IOException;
// Test class
public class Test {
// this method writes the given data into the specified file
// and closes the stream
static void writeToFile(String greetings, String firstName, String lastName) throws IOException {
FileWriter fileWriter = new FileWriter("Files/file.txt", true);
String customizedGreetings = greetings + "! " + firstName + " " + lastName;
fileWriter.write(customizedGreetings + "\n");
fileWriter.flush();
fileWriter.close();
} // end writeToFile()
// main()
public static void main(String[] args) {
// writeToFile() is called to write data into the file
try {
writeToFile("Hi", "Mehvish", "Ashiq");
writeToFile("Hello", "Tahir", "Raza");
} catch (IOException e) {
e.printStackTrace();
}
} // end main()
} // end Test class
출력(file.txt
의 데이터):
Hi! Mehvish Ashiq
Hello! Tahir Raza
close()
를 writeToFile()
외부로 이동하여 java.io.IOException: Stream closed
오류 수정
두 번째 솔루션은 close()
메서드를 writeToFile()
함수 외부로 이동하는 것인데, 이는 솔루션 1에 비해 좋은 접근 방식으로 보입니다.
예제 코드:
// import libraries
import java.io.FileWriter;
import java.io.IOException;
// Test Class
public class Test {
// this method writes the given data into the specified file
static void writeToFile(
String greetings, String firstName, String lastName, FileWriter fileWriter) {
String customizedGreetings = greetings + "! " + firstName + " " + lastName;
try {
fileWriter.write(customizedGreetings + "\n");
fileWriter.flush();
} catch (IOException exception) {
exception.printStackTrace();
}
} // end writeToFile()
// closes the stream
static void cleanUp(FileWriter fileWriter) throws IOException {
fileWriter.close();
} // end cleanUp()
// main()
public static void main(String[] args) throws IOException {
// create the file in the append mode and keep it open
FileWriter fileWriter = new FileWriter("Files/file.txt", true);
// writeToFile() is called to write data into the file.txt
writeToFile("Hi", "Mehvish", "Ashiq", fileWriter);
writeToFile("Hello", "Tahir", "Raza", fileWriter);
// close the stream
cleanUp(fileWriter);
} // end main()
} // end Test class
출력(file.txt
의 데이터):
Hi! Mehvish Ashiq
Hello! Tahir Raza