Java의 이중 콜론 연산자(::)
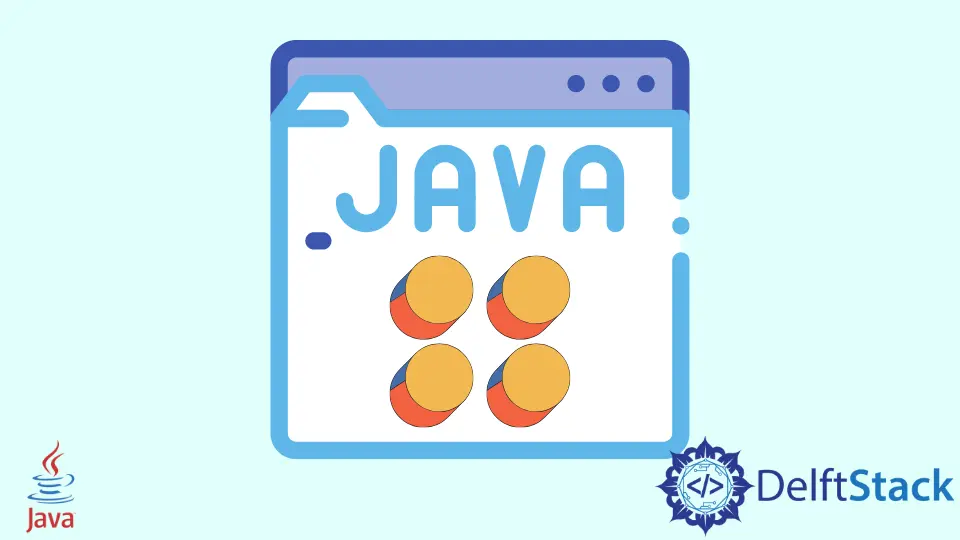
Java에서는 이중 콜론 연산자(::
)를 사용하여 해당 클래스 이름을 통해 메서드를 참조하여 메서드를 호출할 수 있습니다.
람다 식을 사용하여 이 작업을 수행할 수도 있습니다. 여기에서 찾을 수 있는 유일한 차이점은 ::
연산자를 사용하는 동안 이름으로 메서드를 직접 참조할 수 있다는 것입니다.
반면 람다 식을 사용할 때는 호출해야 하는 메서드에 대한 대리자가 필요합니다. 이 기사에서는 Java에서 ::
연산자를 사용하는 방법에 대해 설명합니다.
Java에서 ::
연산자를 사용할 수 있는 곳은 다양합니다. 메소드를 참조하기 위해 ::
연산자를 사용할 수 있습니다.
따라서 ::
를 사용하여 클래스나 객체에서 정적 메서드를 추출할 수 있습니다. 생성자에 ::
연산자를 사용할 수도 있습니다.
또한 입력 스트림이 제공되면 ::
연산자를 사용하여 입력 스트림의 요소를 인쇄할 수 있습니다.
Java에서 이중 콜론(::
) 연산자를 사용하는 경우 및 방법
::
연산자를 사용하여 정적 메서드, 인스턴스 메서드, 수퍼 메서드, 특정 유형의 임의 개체의 인스턴스 메서드, 심지어 클래스 생성자를 처리할 수 있습니다.
각각의 접근 방식에 대해 하나씩 논의해 보겠습니다.
정적 메서드를 다루는 동안
정적 메소드를 다룰 때 Java에서 ::
연산자를 사용할 수 있습니다. 클래스의 정적 메서드에 대한 참조를 얻으려면 먼저 클래스 이름을 작성한 다음 ::
연산자를 작성한 다음 메서드 이름을 작성합니다.
이 접근 방식의 구문을 살펴보겠습니다.
통사론:
(ClassName::methodName)
이제 작동 방식을 이해하기 위해 아래 코드를 살펴보겠습니다.
import java.util.*;
public class Main {
static void staticFunction(String s) // static function which is called using :: operator
{
System.out.println(s);
}
public static void main(String args[]) {
List<String> list = new ArrayList<String>();
list.add("This");
list.add("is");
list.add("an");
list.add("example");
list.forEach(Main::staticFunction);
}
}
출력:
This
is
an
example
인스턴스 메소드를 다루는 동안
인스턴스 메소드를 다룰 때 Java에서 ::
연산자를 사용할 수도 있습니다.
클래스의 인스턴스 메소드에 대한 참조를 얻으려면 먼저 클래스의 객체를 작성합니다. 그런 다음 ::
연산자를 넣고 마지막으로 메서드 이름을 작성합니다.
이 접근 방식의 구문을 살펴보겠습니다.
통사론:
(ObjectOfTheClass::methodName)
이제 작동 방식을 이해하기 위해 아래 코드를 살펴보겠습니다.
import java.util.*;
public class Main {
void instanceFunction(String s) // function which is called using :: operator
{
System.out.println(s);
}
public static void main(String args[]) {
List<String> list = new ArrayList<String>();
list.add("This");
list.add("is");
list.add("an");
list.add("example");
Main object = new Main();
list.forEach(object::instanceFunction);
}
}
출력:
This
is
an
example
슈퍼 메소드를 다루는 동안
수퍼 메소드를 다룰 때 Java에서 ::
연산자를 사용할 수도 있습니다.
클래스의 super 메소드를 참조하기 위해 먼저 super
키워드를 작성합니다. 그런 다음 ::
연산자를 넣고 마지막으로 메서드 이름을 작성합니다.
이 접근 방식의 구문을 살펴보겠습니다.
통사론:
(super::methodName)
이제 작동 방식을 이해하기 위해 아래 코드를 살펴보겠습니다.
// Java code to show use of double colon operator for super methods
import java.util.*;
import java.util.function.*;
class SuperClass {
// super function to be called
String printFunction(String s) {
return ("This is Print function output from the SuperClass: " + s);
}
}
public class Main extends SuperClass {
// instance method to override super method
@Override
String printFunction(String s) {
// call the super method
// using double colon operator
Function<String, String> func = super::printFunction;
String newValue = func.apply(s);
System.out.println(newValue);
return newValue;
}
public static void main(String[] args) {
List<String> list = new ArrayList<String>();
list.add("This");
list.add("is");
list.add("an");
list.add("example");
Main object = new Main();
list.forEach(object::printFunction);
}
}
출력:
This is Print function output from the SuperClass: This
This is Print function output from the SuperClass: is
This is Print function output from the SuperClass: an
This is Print function output from the SuperClass: example
특정 유형의 임의 개체의 인스턴스 메서드를 처리하는 동안
특정 유형의 임의 객체의 인스턴스 메소드를 처리할 때 Java에서 ::
연산자를 사용할 수도 있습니다.
특정 유형의 임의 개체의 인스턴스 메서드를 참조하려면 먼저 클래스 이름을 작성합니다. 그런 다음 ::
연산자를 넣고 마지막으로 메서드 이름을 작성합니다.
이 접근 방식의 구문을 살펴보겠습니다.
통사론:
(ClassName::methodName)
이제 작동 방식을 이해하기 위해 아래 코드를 살펴보겠습니다.
// Java code to show the use of double colon operator for instance method of arbitrary type
import java.util.*;
class Test {
String s = null;
Test(String s) {
this.s = s;
}
// instance function to be called
void instanceFunction() {
System.out.println(this.s);
}
}
public class Main {
public static void main(String[] args) {
List<Test> list = new ArrayList<Test>();
list.add(new Test("This"));
list.add(new Test("is"));
list.add(new Test("an"));
list.add(new Test("example"));
list.forEach(Test::instanceFunction); // call the instance method using double colon operator
}
}
출력:
This
is
an
example
클래스 생성자를 다루는 동안
클래스 생성자를 다룰 때 Java에서 ::
연산자를 사용할 수도 있습니다.
클래스 생성자에 대한 참조를 얻으려면 먼저 클래스 이름을 작성합니다. 그런 다음 ::
연산자를 넣고 마지막으로 특정 클래스의 생성자를 호출하는 new
를 작성합니다.
이 접근 방식의 구문을 살펴보겠습니다.
통사론:
(ClassName::new)
이제 작동 방식을 이해하기 위해 아래 코드를 살펴보겠습니다.
// Java code to show the use of double colon operator for class constructor
import java.util.*;
public class Main {
public Main(String s) {
System.out.println(s);
}
public static void main(String[] args) {
List<String> list = new ArrayList<String>();
list.add("This");
list.add("is");
list.add("an");
list.add("example");
list.forEach(Main::new); // call the instance method using double colon operator
}
}
출력:
This
is
an
example
결론
이 글에서는 자바에서 이중 콜론 연산자(::
)를 사용하여 다섯 곳을 살펴보았다.
모든 기술에는 의미가 있으며 위의 상황이 발생할 때마다 ::
연산자를 적용할 수 있습니다. 부적절하게 사용하면 잘못된 상황이 발생할 수 있으므로 구문에 주의하십시오.