Golang에서 두 개의 지도 병합
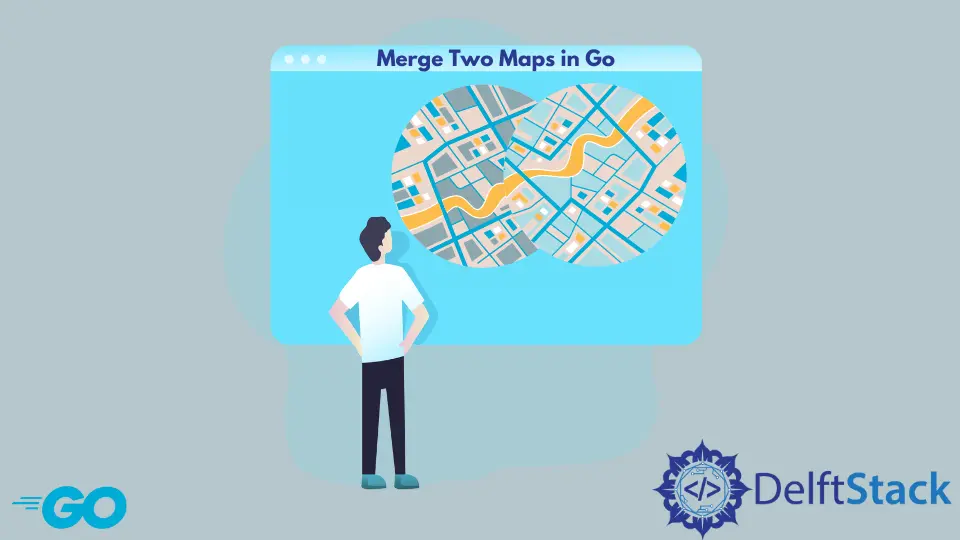
이 튜토리얼은 Golang에서 두 개의 맵을 병합하는 방법을 보여줍니다.
Golang은 지도를 병합하는 내장 기능을 제공하지 않지만 copy
방법을 사용하거나 해당 프로세스를 따라 수행할 수 있습니다. 두 가지 방법을 모두 시도해 봅시다.
copy
방법을 사용하여 Golang에서 두 개의 지도 병합
copy
방법을 사용하여 지도의 병합 기능을 수행할 수 있는 한 지도의 내용을 다른 지도로 복사할 수 있습니다. copy
방법은 Golang 1.18 버전에서 도입되었습니다.
copy
방법은 맵인 두 개의 매개변수를 사용합니다. 두 번째 맵의 콘텐츠가 첫 번째 맵에 복사됩니다.
예를 들어 보겠습니다.
package main
import (
"fmt"
"golang.org/x/exp/maps"
)
func main() {
map1 := map[string]int{
"DelftstackOne": 10,
"DelftstackTwo": 20,
"DelftstackThree": 30,
}
map2 := map[string]int{
"DelftstackTwo": 20,
"DelftstackThree": 30,
"DelftstackFour": 40,
"DelftstackFive": 50,
}
// Print the map before merging
fmt.Println("The First Map: ", map1)
fmt.Println("The Second Map: ", map2)
maps.Copy(map1, map2)
fmt.Println("The merged Map is: ", map1)
}
위의 코드는 copy
방법을 사용하여 합집합을 기반으로 두 개의 맵을 병합합니다. copy
방법의 유일한 단점은 첫 번째 맵을 수정한다는 것입니다.
출력을 참조하십시오.
The First Map: map[DelftstackOne:10 DelftstackThree:30 DelftstackTwo:20]
The Second Map: map[DelftstackFive:50 DelftstackFour:40 DelftstackThree:30 DelftstackTwo:20]
The merged Map is: map[DelftstackFive:50 DelftstackFour:40 DelftstackOne:10 DelftstackThree:30 DelftstackTwo:20]
Program exited.
Golang에서 두 개의 맵을 병합하는 사용자 정의 방법
동일한 값을 가진 항목을 제외하는 통합을 기반으로 두 맵을 병합하는 방법을 설계할 수 있습니다. 다음은 예입니다.
package main
import "fmt"
type Delftstack struct {
Id int
Name string
Salary int
}
type EmployeeMaps map[int]Delftstack
func MapUnion(map1, map2 EmployeeMaps) EmployeeMaps {
for x, y := range map1 {
if m, n := map2[x]; n {
y.Salary += m.Salary
}
map2[x] = y
}
return map2
}
func main() {
map1 := EmployeeMaps{2: {30, "Jack", 2000}, 5: {50, "Samuel", 1500}, 6: {60, "John", 1800}}
map2 := EmployeeMaps{1: {10, "Pamela", 3000}, 2: {20, "Connor", 2000}, 3: {30, "Jack", 2000}, 4: {40, "Joe", 4000}}
result := MapUnion(map2, map1)
fmt.Println(result)
}
위의 코드는 기본적으로 맵의 합집합인 동일한 값을 가진 항목을 제외하여 두 개의 맵을 병합합니다. 코드는 먼저 구조체를 만들고 int 키와 구조체 값으로 매핑합니다. 그런 다음 사용자 정의 방법 MapUnion
을 사용하여 값의 합집합을 기반으로 병합합니다.
출력을 참조하십시오.
map[1:{10 Pamela 3000} 2:{20 Connor 4000} 3:{30 Jack 2000} 4:{40 Joe 4000} 5:{50 Samuel 1500} 6:{60 John 1800}]
Program exited.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook