Go의 로그 수준
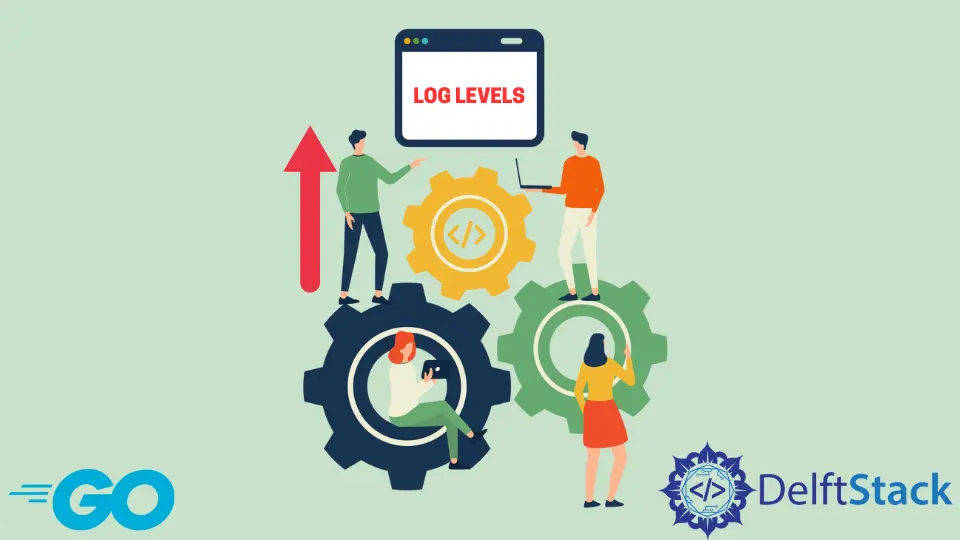
이 튜토리얼은 Golang에서 로그 수준을 만들고 사용하는 방법을 보여줍니다.
Go의 로그 수준
Golang은 간단한 로깅 패키지인 log
라는 로깅 패키지를 제공합니다. 이 패키지는 수준별 로깅을 제공하지 않습니다. 수준별 로깅을 원하는 경우 debug
, info
및 warn
과 같은 접두사를 수동으로 추가해야 합니다.
Golang log
패키지에도 수준별 로그 기능이 없지만 로그 수준을 수동으로 구현하는 기본 도구는 여전히 제공합니다. Golang log
패키지에 대한 기본 예제를 시도해 보겠습니다.
package main
import "log"
func main() {
log.Println("Hello, This is delftstack.com!")
}
위의 코드는 주어진 내용을 기록하고 인쇄합니다. 출력을 참조하십시오.
2009/11/10 23:00:00 Hello, This is delftstack.com!
그러나 로그 수준은 어떻습니까? 위에서 언급했듯이 사용자 지정 로그 수준과 사용자 지정 로거를 만들 수 있습니다.
새 로거를 생성하기 위해 다음 세 가지 매개변수를 사용하는 log.New()
메서드를 사용할 수 있습니다.
out
-out
은 로그 데이터를 쓰기 위해io.writer
인터페이스를 구현하는 데 사용됩니다.접두사
-접두사
는 각 줄의 시작 부분에 추가되는 문자열입니다.플래그
-플래그
는 로거에서 사용할 속성을 정의하는 데 사용됩니다.
이제 일반적으로 7개의 로그 수준이 사용됩니다.
로그 수준 | 설명 |
---|---|
Trace |
가장 낮은 단계 |
Debug |
두 번째 수준 |
Info |
세 번째 수준 |
Warn |
4층 |
Error |
다섯 번째 수준 |
Fatal |
여섯 번째 수준 |
Panic |
최고 수준 |
정보
, 경고
, 디버그
및 오류
수준을 기반으로 사용자 지정 로깅 수준을 만드는 예제를 시도해 보겠습니다.
package main
import (
"fmt"
"io/ioutil"
"log"
"os"
)
var (
Warning_Level *log.Logger
Info_Level *log.Logger
Debug_Level *log.Logger
Error_Level *log.Logger
)
func init() {
file, err := os.OpenFile("Demo_logs.txt", os.O_APPEND|os.O_CREATE|os.O_WRONLY, 0666)
if err != nil {
log.Fatal(err)
}
Info_Level = log.New(file, "INFO: ", log.Ldate|log.Ltime|log.Lshortfile)
Warning_Level = log.New(file, "WARNING: ", log.Ldate|log.Ltime|log.Lshortfile)
Debug_Level = log.New(file, "Debug: ", log.Ldate|log.Ltime|log.Lshortfile)
Error_Level = log.New(file, "ERROR: ", log.Ldate|log.Ltime|log.Lshortfile)
}
func main() {
Info_Level.Println("Loading the application.")
Info_Level.Println("Loading taking time.")
Warning_Level.Println("There is warning.")
Debug_Level.Println("Here is debugging information.")
Error_Level.Println("An Error Occured.")
// read the log file
data, err := ioutil.ReadFile("Demo_logs.txt")
if err != nil {
log.Panicf("failed reading data from file: %s", err)
}
fmt.Printf("\nThe file data is : %s", data)
}
위의 코드는 Golang의 log
패키지를 사용하여 사용자 정의 로그 수준을 생성하고 로그 정보를 파일에 기록합니다. 출력을 참조하십시오.
The file data is : INFO: 2009/11/10 23:00:00 prog.go:32: Loading the application.
INFO: 2009/11/10 23:00:00 prog.go:33: Loading taking time.
WARNING: 2009/11/10 23:00:00 prog.go:34: There is warning.
Debug: 2009/11/10 23:00:00 prog.go:35: Here is debugging information.
ERROR: 2009/11/10 23:00:00 prog.go:36: An Error Occured.
Program exited.
‘Fatal’ 및 ‘Panic’ 수준은 또한 프로그램 또는 애플리케이션의 종료를 유발할 수 있으며, 이것이 내장된 ’log’ 패키지로 이러한 로그 수준을 생성하는 것이 불가능한 이유입니다. 그러나 log
패키지 외에 일부 타사 로그 패키지는 7가지 기본 제공 로그 수준을 제공합니다. 그들을 탐구하자.
zerolog
와 함께 이동의 로그 수준
zerolog
는 구조화된 JSON 로깅에 사용되는 Golang용 타사 라이브러리입니다. zerolog
는 간단한 로깅을 위해 log
하위 패키지와 함께 사용할 수 있는 글로벌 로거를 제공합니다.
단순 로깅과 함께 zerolog
는 내장 로그 수준도 제공합니다. zerolog
에는 Info
, Warning
, Debug
, Error
및 Trace
를 포함하는 7개의 로그 수준이 있습니다.
zerolog
패키지는 GitHub에서 제공되며 다음 명령을 사용하여 Golang에서 다운로드할 수 있습니다.
go get -u github.com/rs/zerolog/log
zerolog
를 사용하면 로그 수준을 사용하는 것이 매우 간단합니다. 아래 예를 참조하십시오.
package main
import (
"github.com/rs/zerolog"
"github.com/rs/zerolog/log"
)
func main() {
zerolog.SetGlobalLevel(zerolog.InfoLevel)
log.Trace().Msg("Tracing..")
log.Info().Msg("The file is loading.")
log.Debug().Msg("Here is some useful debugging information.")
log.Warn().Msg("There is a warning!")
log.Error().Msg("An Error Occured.")
log.Fatal().Msg("An Fatal Error Occured.")
log.Panic().Msg("This is a panic situation.")
}
위의 코드는 로그 수준에 따라 정보를 기록합니다. 출력을 참조하십시오.
{"level":"info","time":"2009-11-10T23:00:00Z","message":"The file is loading."}
{"level":"warn","time":"2009-11-10T23:00:00Z","message":"There is a warning!"}
{"level":"error","time":"2009-11-10T23:00:00Z","message":"An Error Occured."}
{"level":"fatal","time":"2009-11-10T23:00:00Z","message":"An Fatal Error Occured."}
Program exited.
Logrus
와 함께 이동의 로그 수준
‘Logrus’는 JSON 로그인을 제공하는 Golang의 또 다른 타사 패키지입니다. Logrus
패키지는 GitHub에서 제공되며 cmd에서 다음 명령을 사용하여 다운로드할 수 있습니다.
go get "github.com/Sirupsen/logrus"
Logrus
는 심각도에 따라 정렬된 Trace
, Debug
, Info
, Warn
, Error
, Fatal
및 Panic
을 포함하는 7가지 로그 수준을 제공합니다. 이러한 로그 수준을 사용하는 것은 ’log’ 하위 패키지를 사용해야 하는 ‘zerolog’와 유사합니다.
Logrus
의 로그 수준으로 예를 들어 보겠습니다.
package main
import (
log "github.com/sirupsen/logrus"
)
func main() {
log.SetFormatter(&log.JSONFormatter{})
log.SetLevel(log.DebugLevel)
log.SetLevel(log.TraceLevel)
//log.SetLevel(log.PanicLevel)
log.Trace("Tracing the log info, Lowest level")
log.Debug("Debugging information. Level two")
log.Info("Log Info. Level three")
log.Warn("This is warning. Level Four")
log.Error("An error occured. Level Five")
// Calls os.Exit(1) after logging
log.Fatal("Application terminated. Level Six")
// Calls panic() after logging
log.Panic("Panic Situation. Highest Level.")
}
위의 코드는 Logrus
패키지를 사용하여 레벨에 따라 정보를 기록합니다. 일부 로그 수준을 표시하려면 수준을 설정해야 합니다.
출력을 참조하십시오.
{"level":"trace","msg":"Tracing the log info, Lowest level","time":"2009-11-10T23:00:00Z"}
{"level":"debug","msg":"Debugging information. Level two","time":"2009-11-10T23:00:00Z"}
{"level":"info","msg":"Log Info. Level three","time":"2009-11-10T23:00:00Z"}
{"level":"warning","msg":"This is warning. Level Four","time":"2009-11-10T23:00:00Z"}
{"level":"error","msg":"An error occured. Level Five","time":"2009-11-10T23:00:00Z"}
{"level":"fatal","msg":"Application terminated. Level Six","time":"2009-11-10T23:00:00Z"}
Program exited.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook