Golang은 인터페이스를 문자열로 변환합니다.
- 문자열에 대한 Golang 인터페이스
-
fmt.Sprintf
를 사용하여 Golang에서 인터페이스를 문자열로 변환 - Type Casting을 사용하여 Golang에서 인터페이스를 문자열로 변환
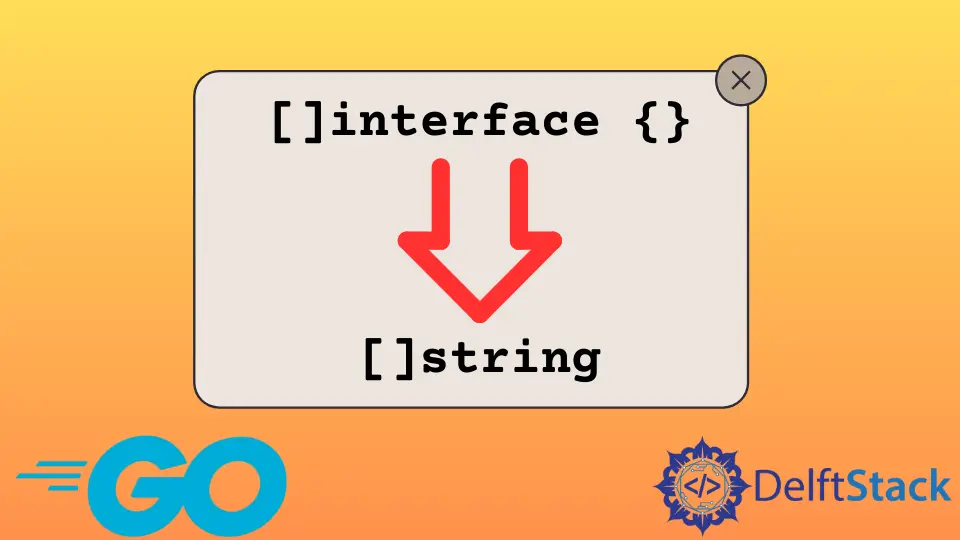
이 튜토리얼은 Golang에서 인터페이스를 문자열로 변환하는 방법을 보여줍니다.
문자열에 대한 Golang 인터페이스
인터페이스는 인터페이스의 메서드에 메서드 본문이 없는 Golang에 구현되지 않은 메서드 집합을 저장합니다. Golang에서 인터페이스는 비어 있을 수 있으며 변수 선언을 통해 인터페이스에 값을 할당할 수 있습니다.
때때로 Golang에서 인터페이스를 문자열로 변환해야 합니다. Golang에서 인터페이스를 문자열로 변환하는 두 가지 방법이 있습니다.
fmt.Sprintf
를 사용하여 Golang에서 인터페이스를 문자열로 변환
fmt.Sprintf
메서드는 Golang에서 무엇이든 문자열로 변환할 수 있습니다. 이 메서드는 인터페이스를 Golang의 문자열로 변환할 수도 있습니다.
예를 보자:
package main
import "fmt"
func main() {
//Interface with a string value
var Demo interface{} = "Delftstack"
resultString := fmt.Sprintf("%v", Demo)
fmt.Println(resultString)
//Interface with an array value
var Demo1 interface{} = []int{10, 20, 30, 40, 50}
resultString1 := fmt.Sprintf("%v", Demo1)
fmt.Println(resultString1)
}
위의 코드는 값 문자열과 배열이 있는 인터페이스를 문자열로 변환합니다. 출력을 참조하십시오.
Delftstack
[10 20 30 40 50]
Program exited.
하지만 인터페이스가 여러 값을 가진 배열이라면 어떻게 될까요? 이 경우 인터페이스 길이의 빈 슬라이스를 만들고 for
루프를 사용하여 각 값을 문자열로 변환하고 사용하거나 슬라이스에 넣을 수 있습니다.
예를 참조하십시오:
package main
import "fmt"
func main() {
Demo := []interface{}{
"Delftstack",
10, 20, 30,
[]int{6, 5, 9},
struct{ A, B int }{10, 20},
}
// Print the interface content
fmt.Println(Demo)
// Print the original type before conversion
fmt.Printf("Original Type of interface: %T\n", Demo)
// now create an empty slice based on the length of the Interface
emptyslice := make([]string, len(Demo))
// Iterate over the Interface and convert values to string
for index, value := range Demo {
emptyslice[index] = fmt.Sprint(value)
}
// Print the content of the slice
fmt.Println(emptyslice)
// Print the type after conversion
fmt.Printf("The new type is: %T\n", emptyslice)
fmt.Printf("%q\n", emptyslice)
}
위의 코드는 여러 값이 있는 인터페이스를 문자열로 변환합니다. 출력 참조:
[Delftstack 10 20 30 [6 5 9] {10 20}]
Original Type of interface: []interface {}
[Delftstack 10 20 30 [6 5 9] {10 20}]
The new type is: []string
["Delftstack" "10" "20" "30" "[6 5 9]" "{10 20}"]
Program exited.
Type Casting을 사용하여 Golang에서 인터페이스를 문자열로 변환
Golang에서도 타입 캐스팅이 가능합니다. 인터페이스를 문자열로 유형 변환할 수 있습니다. 구문 .(문자열)
을 사용하여 무엇이든 문자열로 캐스트하는 프로세스는 매우 간단합니다.
예를 들어 보겠습니다.
package main
import "fmt"
func main() {
//Interface with a string value
var Demo interface{} = "Delftstack"
resultString := Demo.(string)
fmt.Println(resultString)
}
이 경우 문자열 값이 있는 인터페이스만 문자열로 유형 변환할 수 있습니다. 배열 값이 있는 인터페이스는 유형 변환될 수 없습니다.
출력을 참조하십시오.
Delftstack
Program exited.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook