C#에서 직렬화 가능 특성 만들기
-
SoapFormatter
또는SerializableAttribute
클래스를 사용하여C#
에서 직렬화 가능 속성 생성 -
BinaryFormatter
를 사용하여C#
에서 직렬화 가능 속성 생성 -
XMLSerializer
를 사용하여C#
에서 직렬화 가능 속성 생성
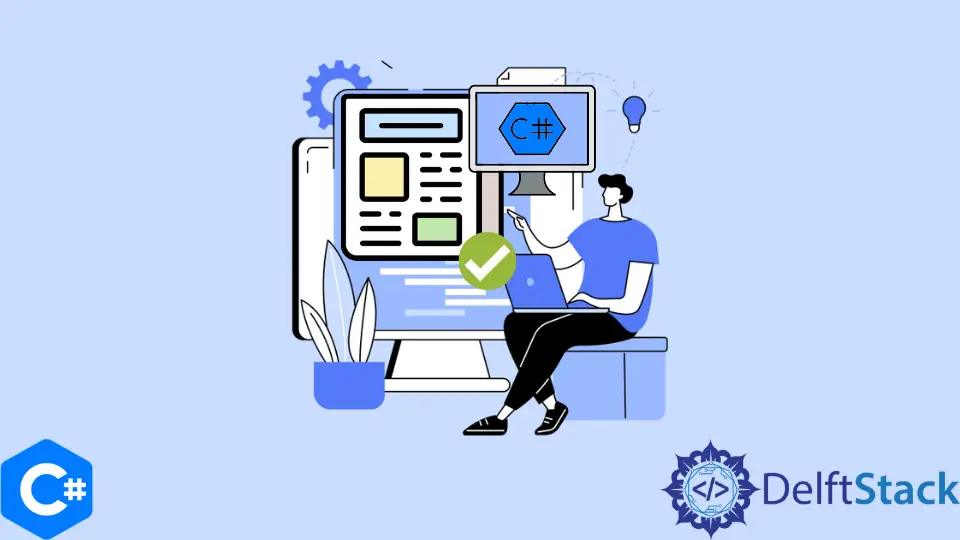
이 자습서에서는 C#에서 특성을 정확하게 사용하기 위해 특성의 직렬화 가능성을 활성화하는 세 가지 기본 방법을 배웁니다. 속성 직렬화는 속성 또는 객체를 메모리에서 일련의 비트로 유지하기 위한 여러 단계를 기반으로 하는 프로세스입니다.
C#에서 클래스를 직렬화하면 자동으로 모든 요소에 영향을 미치고 특성의 표시된 필드만 직렬화되지 않습니다. 즉, 루트 개체를 직렬화하는 것만으로 복잡한 데이터 구조를 쉽게 저장할 수 있습니다.
‘Hibernate’는 객체 또는 속성(직렬화 가능한 클래스의 경우)을 바이트 크기로 저장하고 역직렬화가 필요할 때마다 객체를 다른 시스템으로 전송하는 직렬화 가능한 속성의 뛰어난 현대식 아키텍처 예입니다.
SoapFormatter
또는 SerializableAttribute
클래스를 사용하여 C#
에서 직렬화 가능 속성 생성
SerializableAttribute
로 표시된 공용 또는 개인의 모든 속성은 Iserializable
인터페이스가 속성 직렬화 프로세스를 재정의하지 않는 한 자동으로 직렬화됩니다. 직렬화 프로세스에서 일부 속성을 제외하려면 NonSerializedAttribute
가 매우 유용합니다.
SerializableAttribute
특성 표시는 SoapFormatter
또는 BinaryFormatter
및 XmlSerializer
를 사용할 때만 적용됩니다. DataContractSerializer
및 JaveScriptSerializer
는 이를 지원하거나 인식하지 않습니다. BinaryFormatter
클래스는 System.Runtime.Serialization.Formatters.Binary
네임스페이스에 속하며 직렬화 가능 속성은 Clipboard.SetData()
를 사용하여 클립보드에 저장됩니다.
Attribute의 이진 직렬화는 최적화된 메모리 및 시간 소비로 인해 더 효율적입니다. 그러나 사람이 읽을 수는 없습니다. 반면에 SOAP 직렬화는 효율적이거나 최적화되지 않았지만 C# 코드를 읽을 수 있게 합니다.
XML 직렬화는 NonSerialized
대신 XmlIgnore
를 사용하고 System.Xml.Serialization
네임스페이스에 있기 때문에 Serializable
을 무시하므로 약간 다릅니다. 개인 클래스 멤버를 직렬화할 수 없습니다.
// using `System.Runtime.Serialization` namespace is optional
using System;
using System.IO;
using System.Windows.Forms;
// required | essential namespace
using System.Runtime > Serialization.Formatters.Soap;
namespace serializable_attribute {
public partial class Form1 : Form {
[Serializable()]
public class test_class {
public int attribute_one;
public string attribute_two;
public string attribute_three;
public double attribute_four;
// the `attribute_five` as the fifth member is not serialized
[NonSerialized()]
public string attribute_five;
public test_class() {
attribute_one = 11;
attribute_two = "the lord of the rings";
attribute_three = "valerian steel";
attribute_four = 3.14159265;
attribute_five = "i am unserialized text!";
}
public void Print() {
// MessageBox.Show(attribute_five.ToString()); remember, object reference is NULL
string delimiter = " , ";
string messageBoxContent = String.Join(delimiter, attribute_one, attribute_two,
attribute_three, attribute_four, attribute_five);
MessageBox.Show(messageBoxContent);
}
}
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
test_class obj = new test_class();
Stream serialized_stream = File.Open(
"data.xml", FileMode.Open); // replace your `.xml` file location or let it be default
// define a new `SoapFormatter` object
SoapFormatter formatter_soap = new SoapFormatter();
// serialize the object with the stream
formatter_soap.Serialize(serialized_stream, obj);
serialized_stream.Close(); // close the stream
// obj = null; | optional
serialized_stream = File.Open("data.xml", FileMode.Open);
formatter_soap = new SoapFormatter();
// deserialize the stream w.r.t the serializable object
obj = (test_class)formatter_soap.Deserialize(serialized_stream);
// close the stream
serialized_stream.Close();
// print the serialized object
obj.Print();
}
}
}
출력:
11, the lord of the rings, valerian steel, 3.14159265 ,
입니다. 역직렬화는 직렬화 가능 속성의 버전 번호가 올바른 경우에만 발생합니다.
역직렬화 중에 오류가 발생할 수 있는 컴파일러 구현에 따라 매우 민감한 계산 때문에 좋은 프로그래머는 항상 버전 번호를 명시적으로 선언해야 합니다.
직렬화 가능 속성은 파일에 저장하거나 쓸 수 있습니다. 다형성을 이해한다면 이것은 매우 의미가 있습니다. 속성을 직렬화하거나 메모리, 데이터베이스 또는 파일에 저장하기 위해 바이트 스트림으로 변환하여 상태를 저장할 수 있습니다.
속성 직렬화는 웹 서버에서 원격 애플리케이션으로 전송하거나 한 도메인에서 다른 도메인으로 전달하거나 방화벽을 통해 JSON 또는 XML 문자열로 전달하거나 애플리케이션 간에 보안 또는 사용자별 정보를 유지 관리하는 데 필수적입니다.
BinaryFormatter
를 사용하여 C#
에서 직렬화 가능 속성 생성
직렬화 프로세스는 C#에서 Serializable
특성을 사용하여 각 클래스, 인터페이스 또는 구조를 장식하는 데 중요합니다. 유효하고 읽을 수 있는 이름으로 FileStream
객체를 생성하는 것은 속성을 직렬화하는 데 중요합니다.
Serialize()
메서드는 BinaryFormatter
개체의 도움으로 만들 수 있습니다. BinaryFormatter.Deserialize()
메소드는 적절한 유형으로 캐스트되어야 하는 객체를 반환합니다.
객체 또는 속성의 직렬화 프로세스를 완전히 배우려면 역직렬화 프로세스를 이해해야 합니다.
직렬화 가능 속성의 역직렬화 프로세스는 참조를 null
로 설정하고 처음부터 완전히 새로운 배열을 생성하여 역직렬화된 테스트 개체 배열을 수용하는 것으로 시작합니다. 다음 실행 가능한 C# 프로그램에서 이를 배우게 되며 SerializeNow()
및 DeSerializeNow()
메소드도 각각 배우게 됩니다.
일반적으로 속성 직렬화는 직렬화된 개체 위치의 인스턴스 만들기, 파일 개체에서 스트림 만들기, BinaryFormatter
인스턴스 만들기, 마지막으로 Serialize()
메서드 호출로 구성됩니다.
using System;
using System.IO;
using System.Runtime.Serialization.Formatters.Binary;
using System.Windows.Forms;
namespace serializable_attribute {
public partial class Form1 : Form {
[Serializable()]
public class test_class {
public int attribute_one;
public string attribute_two;
public string attribute_three;
public double attribute_four;
// the `attribute_five` as the fifth member is not serialized
[NonSerialized()]
public string attribute_five;
public test_class() {
attribute_one = 11;
attribute_two = "the lord of the rings";
attribute_three = "valerian steel";
attribute_four = 3.14159265;
attribute_five = "i am unserialized text!";
}
public void Print() {
// MessageBox.Show(attribute_five.ToString()); remember, object reference is NULL
string delimiter = " , ";
string messageBoxContent = String.Join(delimiter, attribute_one, attribute_two,
attribute_three, attribute_four, attribute_five);
MessageBox.Show(messageBoxContent);
}
}
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
test_class obj = new test_class();
Stream serialized_stream = File.Open("data.xml", FileMode.Create);
BinaryFormatter formatter_binary = new BinaryFormatter();
formatter_binary.Serialize(serialized_stream, obj);
serialized_stream.Close();
// obj = null;
serialized_stream = File.Open("data.xml", FileMode.Open);
formatter_binary = new BinaryFormatter();
obj = (test_class)formatter_binary.Deserialize(serialized_stream);
serialized_stream.Close();
obj.Print();
}
}
}
출력:
11, the lord of the rings, valerian steel, 3.14159265 ,
바이너리 또는 XML 직렬화 및 역직렬화를 위한 클래스는 System.Runtime.Serialization
네임스페이스에 속하므로 직렬화 프로세스의 필수 부분/구성 요소가 됩니다. XML 및 이진 직렬화 모두 별도의 이점을 제공할 수 있습니다.
속성의 이진 직렬화는 소켓 기반 네트워크 스트림을 사용하며 모든 구성원은 읽기 전용이므로 성능을 향상시킬 수 있습니다. 반면에 XML 직렬화는 특정 XSD(XML 스키마 정의 언어) 문서를 준수하는 XML 스트림을 사용하므로 공용 속성이 있는 강력한 형식의 특성이 생성됩니다.
또한 유형에 대한 직렬화 가능 속성에 SerializableAttribute
를 사용하여 직렬화 또는 기타 기능을 나타낼 수도 있습니다. 핸들, 포인터 또는 특정 조건이나 환경과 관련되거나 연결된 기타 데이터 구조를 포함하는 특성을 직렬화하지 않는 것이 좋습니다.
XMLSerializer
를 사용하여 C#
에서 직렬화 가능 속성 생성
XML 형식의 디스크에 대한 개체의 상태 지속성을 활성화합니다. C#에서 XMLSerializer
를 사용하려면 System.Xml
및 System.Xml.Serialization
네임스페이스를 가져와야 합니다.
StreamWriter
개체는 개체의 직렬화를 활성화할 수 있습니다. 가장 중요한 것은 Serialize()
및 Deserialize()
메서드 생성은 위와 동일하지만 BinaryFormatter
를 사용하는 대신 XMLSerializer
를 사용하게 됩니다.
C# 프로그램의 bin
또는 debug
폴더에서 직렬화된 속성의 .xml
파일을 찾을 수 있습니다. XML 인코딩에서 테스트 클래스의 지속 상태를 관찰할 수 있습니다. Xsd.exe
는 훌륭한 실행 프로그램 또는 기존 XSD(XML 스키마 정의) 문서를 기반으로 클래스를 만드는 XML 스키마 정의 도구로 작동하는 도구입니다.
using System;
using System.IO;
using System.Xml.Serialization;
using System.Windows.Forms;
namespace serializable_attribute {
[Serializable]
public class XMLSerialize {
public XMLSerialize() {}
public XMLSerialize(string rank, string sol_name) {
this._rank = rank;
this._name = sol_name;
}
public string _rank { get; set; }
public string _name { get; set; }
public override string ToString() {
return (_rank + " " + _name);
}
}
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
XMLSerialize[] ser_obj = new XMLSerialize[3];
ser_obj[0] = new XMLSerialize("Captain", "Floyyd");
ser_obj[1] = new XMLSerialize("Major", "Gerizmann");
ser_obj[2] = new XMLSerialize("Sec. Lef.", "Angel");
TextWriter obj_txtWriter = null;
obj_txtWriter = new StreamWriter("test.xml");
XmlSerializer obj_xmlSerl = new XmlSerializer(typeof(XMLSerialize[]));
obj_xmlSerl.Serialize(obj_txtWriter, ser_obj);
string delimiter = " , ";
string messageBoxContent = String.Join(delimiter, ser_obj[0], ser_obj[1], ser_obj[2]);
MessageBox.Show(messageBoxContent);
obj_txtWriter.Close();
obj_txtWriter = null;
}
}
}
출력:
Captain Floyyd, Major Gerizmann, Sec. Lef. Angel
C#에서 특성의 직렬화는 기본 및 사용자 지정 직렬화의 두 가지 유형일 수 있습니다. 속성의 기본 직렬화는 .NET을 사용하여 속성을 자동으로 직렬화하기 때문에 쉽습니다. 유일한 요구 사항은 클래스에 SerializableAttribute
속성이 적용된다는 것입니다.
사용자 지정 직렬화를 사용하면 직렬화할 개체를 표시할 수 있습니다. 클래스는 SerializableAttribute
로 표시되고 ISerializable
인터페이스를 사용해야 하며 사용자 지정 생성자를 사용하여 사용자 지정 방식으로 역직렬화할 수도 있습니다.
특성의 디자이너 직렬화는 개발 도구와 관련된 특수 특성 지속성을 포함하는 가장 드문 형태의 특성 직렬화입니다. 그래프를 복구하기 위해 소스 파일로 변환하는 과정으로, 그래프에는 마크업이나 C# 코드, 심지어 SQL 테이블 정보까지 포함될 수 있다.
대부분의 직렬화 가능한 속성은 기술적 .custom
인스턴스입니다. 그러나 SerializableAttribute
는 명령줄 인터페이스 .class
플래그(직렬화 가능)에 매핑됩니다. System.SerializableAttribute
는 특성의 직렬화 기능을 지정합니다.
주어진 유형이 System.Runtime.Serialization.ISerializable
인터페이스를 구현하는 경우 NonSerializedAttribute
를 사용할 필요가 없다는 점을 기억하는 것이 중요합니다. 이는 클래스가 고유한 직렬화 및 역직렬화 메서드를 제공함을 나타냅니다. 다음과 같을 수 있습니다.
public sealed class SerializableAttribute : Attribute {public method SerializableAttribute();}
이 자습서에서는 특성을 직렬화하고 C#에서 특성의 직렬화 및 역직렬화를 수정하는 가능한 모든 방법을 배웠습니다.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub