C#에서 진행률 표시줄 만들기
- Windows Forms 앱(.NET Framework)에서 진행률 표시줄 만들기
-
C#
에서BackgroundWorker
클래스를 사용하여 진행률 표시줄 만들기 -
C#
에서 텍스트로 진행률 표시줄 만들기
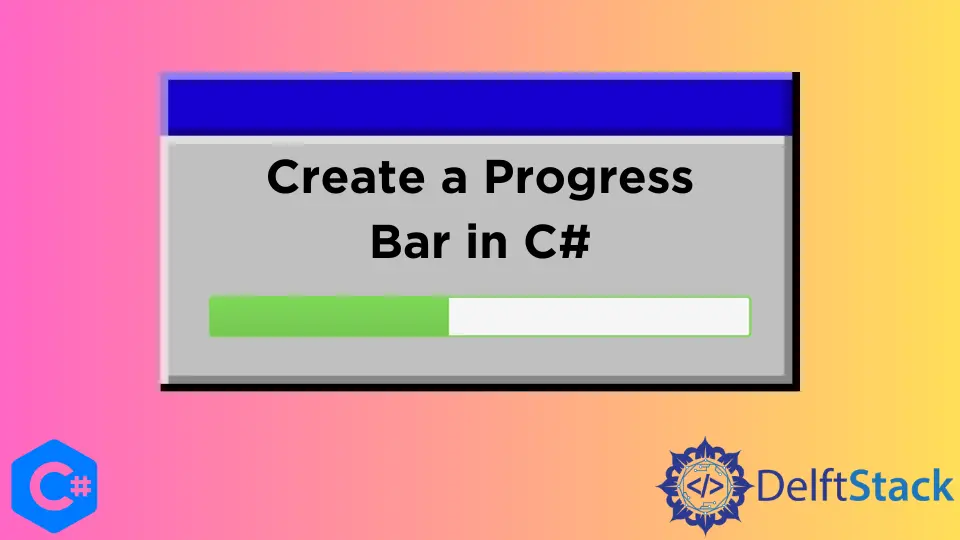
긴 파일 작업 및 계산에는 진행률 표시가 필요하며 진행률 표시줄은 장기 실행 작업 중에 사용자에게 진행률 정보를 표시하는 가장 좋은 방법입니다. 이 자습서에서는 Visual Studio 2022를 사용하여 C#에서 세 가지 진행률 표시줄을 만드는 방법을 알려줍니다.
C#의 동적 진행률 표시줄에는 범위를 지정하는 두 가지 속성(Maximum
및 Minimum
속성)이 있으며, 주로 복사할 파일 또는 실행할 프로세스 수와 동일합니다. 또한 프로세스가 완료되고 진행률 표시줄의 값을 증가시키기 위해 PerformStep
메서드와 함께 Step
속성이 사용됩니다.
C#의 미리 디자인된 진행률 표시줄은 가로로만 방향을 지정할 수 있습니다. 그러나 필요에 따라 사용자 지정 진행률 표시줄을 만들 수 있습니다. 진행률 표시줄의 주요 목적은 C# 애플리케이션에서 현재 수행 중인 작업의 진행률을 사용자에게 알리는 것입니다.
Windows Forms 앱(.NET Framework)에서 진행률 표시줄 만들기
진행률 표시줄은 작업 진행률을 나타내는 사용자 인터페이스 요소 역할을 합니다. C#의 진행률 표시줄은 진행률을 나타내는 두 가지 모드(미확정 및 연속)를 지원합니다.
진행률 표시줄의 불확정 모드는 시간이 얼마나 걸릴지 모르는 작업이나 작업을 수행할 때 유용합니다. C# 애플리케이션이 여전히 사용자에게 응답하는 동안 백그라운드 스레드에서 데이터를 비동기식으로 로드하여 수행됩니다(일반적으로 BackgroundWorker
클래스의 도움으로).
데이터가 로드되고 실제 진행이 시작될 때까지 설정될 때 확정되지 않은 진행률 표시줄을 표시하는 것이 일반적인 디자인 패러다임입니다. WinForms에서 진행률 표시줄을 생성할 때 진행률 표시줄의 Style
속성을 Marquee
로 설정하는 것이 중요합니다.
그러나 WPM의 진행률 표시줄에는 스타일
과 유사한 Isdeterminate
속성이 있으며 True
값은 Marquee
와 유사하게 작동합니다.
// namespaces for C# windows application (.NET Framework)
using System;
using System.Windows.Forms;
namespace ProgressBarExp {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
// create a `progressBar1` progress bar in your C# project design
private void progressBar1_Click(object sender, EventArgs e) {}
// create a method that performs some calculations
// the progress bar will synch with this method and progress accordingly
private void Caluculate(int i) {
// perform a task
}
// create a `RunOperation` button to perform calculations or take action in your C# project
// design
private void RunOperation_Click(object sender, EventArgs e) {
// set the visibility of progress bar
progressBar1.Visible = true;
for (int j = 0; j < 10000001; j++) {
Caluculate(j);
if (j == 10000000) {
MessageBox.Show("Operation Complete!");
progressBar1.Visible = false;
}
}
}
private void Form1_Load(object sender, EventArgs e) {
// for indeterminate progress using .NET framework
progressBar1.Style = ProgressBarStyle.Marquee;
progressBar1.Visible = false;
}
}
}
출력:
진행률 표시줄은 데이터가 로드될 때 진행률을 보고할 때만 그 목적을 올바르게 수행합니다. C# 응용 프로그램에서 진행률 표시줄은 소프트웨어 설치, 긴 작업 수행 및 시작에 널리 사용되므로 사용자는 프로세스에 걸리는 시간과 남은 진행률을 정확히 알 수 있습니다.
C#에서 연속 진행률 표시줄을 만들려면 RunOperation_Click
이벤트에서 코드를 제거하고 다음 코드로 바꿉니다.
private void RunOperation_Click(object sender, EventArgs e) {
progressBar1.Visible = true;
progressBar1.Maximum = 10000000;
progressBar1.Value = 1;
for (int j = 0; j < 10000001; j++) {
Caluculate(j);
if (progressBar1.Value == 10000000) {
MessageBox.Show("Operation Complete!");
progressBar1.Visible = false;
break;
}
// it will measure the progress of the task or operations and progress in regards to the
// completion of a task It only requires if the progress bar style is not set to Marquee
progressBar1.PerformStep();
}
}
출력:
Form1_Load
이벤트에서 progressBar1.Style = ProgressBarStyle.Marquee;
를 주석 처리합니다. C# 코드의 줄과 작업 완료율 %
에 따라 진행률 표시줄이 계속 나타납니다.
C#
에서 BackgroundWorker
클래스를 사용하여 진행률 표시줄 만들기
C# 애플리케이션에서 진행률 표시줄을 사용하는 것이 얼마나 간단한지 명확해야 합니다. 이제 정적 값뿐만 아니라 일부 실제 작업과 동기화하여 진행률 표시줄이 앞으로 이동하는 방식을 연구할 시간입니다.
대부분의 새로운 C# 개발자가 문제에 부딪히는 곳은 동일한 스레드에서 동시에 작업을 수행하고 진행률 표시줄을 업데이트할 수 없다는 사실을 깨달을 때입니다. C# 애플리케이션의 사용자 인터페이스 스레드에서 무거운 작업을 수행하고 그에 따라 진행률 표시줄을 업데이트하려는 경우 C#의 BackgroundWorker
클래스 서비스가 필요합니다.
진행률 표시줄을 거의 쓸모 없게 만드는 BackgroundWorker
클래스의 도움 없이는 말입니다. 진행률 표시줄은 작업이 성공적으로 완료되기 전에는 진행률에 대한 업데이트를 표시하지 않으며 백그라운드 작업자가 단일 스레드에서 둘 다 동시에 업데이트해야 합니다.
작업자 스레드에서 작업을 수행한 다음 업데이트를 사용자 인터페이스 스레드로 푸시하여 진행률 표시줄을 즉시 업데이트하고 이러한 업데이트를 표시하기 위해 C#에서 작업하는 백그라운드로 진행률 표시줄을 만드는 이상적인 방법입니다.
using System;
using System.Windows;
using System.Threading;
using System.Windows.Forms;
using System.ComponentModel;
namespace ProgressBarExp {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
// create a progress bar in your C# project's Form1.cs [Design]
private void progressBar1_Click(object sender, EventArgs e) {}
// a button to perform an operation synched with the `progressBar1`
private void RunOperation_Click(object sender, EventArgs e) {
BackgroundWorker taskSynch = new BackgroundWorker();
taskSynch.WorkerReportsProgress = true;
taskSynch.DoWork += taskSynch_DoWork;
taskSynch.ProgressChanged += taskSynch_ProgressChanged;
taskSynch.RunWorkerAsync();
}
// Form1 load event
private void Form1_Load(object sender, EventArgs e) {}
// create a method that connects `BackgroundWorker` with a task
void taskSynch_DoWork(object sender, DoWorkEventArgs e) {
for (int i = 0; i < 100; i++) {
(sender as BackgroundWorker).ReportProgress(i);
Thread.Sleep(100);
}
}
// create a method that connects a task with the `progressBar1`
void taskSynch_ProgressChanged(object sender, ProgressChangedEventArgs e) {
progressBar1.Value = e.ProgressPercentage;
}
}
}
출력:
C#
에서 텍스트로 진행률 표시줄 만들기
일반적으로 구성을 더 잘 제어할 수 있는 사용자 지정 진행률 표시줄을 만들 수 있습니다. 그러나 진행률 표시줄을 만들기 위해 처음부터 C# 코드를 작성하는 것은 시간이 오래 걸리는 작업입니다. Syncfusion UI Control
또는 ShellProgressBar
와 같은 NuGet
패키지를 사용하여 놀라운 디자인의 바로 사용할 수 있는 진행률 표시줄을 얻을 수 있습니다.
텍스트가 있는 진행률 표시줄을 만드는 가장 간단한 방법은 레이블을 사용하고 작업 진행에 따라 텍스트를 변경하는 것입니다. 진행 상황을 작업 진행 상황과 동기화할 수 있습니다.
using System;
using System.Threading;
using System.Windows.Forms;
using System.ComponentModel;
using System.Drawing;
namespace ProgressBarExp {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void RunOperation_Click(object sender, EventArgs e) {
BackgroundWorker taskSynch = new BackgroundWorker();
taskSynch.WorkerReportsProgress = true;
taskSynch.DoWork += taskSynch_DoWork;
taskSynch.ProgressChanged += taskSynch_ProgressChanged;
taskSynch.RunWorkerAsync();
}
private void Form1_Load(object sender, EventArgs e) {
// make the percentage label `backcolor` transparent
label1.BackColor = Color.Transparent;
}
void taskSynch_DoWork(object sender, DoWorkEventArgs e) {
for (int i = 0; i < 101; i++) {
(sender as BackgroundWorker).ReportProgress(i);
Thread.Sleep(100);
// to support multi-threading for label1 and label 2
if (label1.InvokeRequired) {
label1.Invoke(new MethodInvoker(delegate {
// shows the progression, it is syched with the task progression
label1.Text = "Progress: " + i + "%";
}));
}
if (label2.InvokeRequired) {
label2.Invoke(new MethodInvoker(delegate {
if (i < 25) {
label2.Text = "Starting the task...";
} else if (i > 25 && i < 50) {
label2.Text = "Half way through...";
} else if (i > 50 && i < 99) {
label2.Text = "The wait will be over soon...";
} else if (i == 100) {
label2.Text = "Task is completed successfully!";
}
}));
}
}
}
void taskSynch_ProgressChanged(object sender, ProgressChangedEventArgs e) {
// it will update the progress bar in real time depends on the progress of a task
progressBar1.Value = e.ProgressPercentage;
}
}
}
출력:
이 C# 코드를 실행하기 전에 C# 프로젝트의 Form1 [Design]
에서 progressBar1
진행률 표시줄, 작업 실행
버튼, label1
및 label2
레이블을 만들어야 합니다.
이 자습서에서는 다양한 유형의 진행률 표시줄을 만들고 C#의 BackgroundWorker
클래스를 사용하여 작업의 실제 진행률과 동기화하는 방법을 배웠습니다.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub