C#의 오버로드 할당 연산자
Muhammad Zeeshan
2023년10월12일
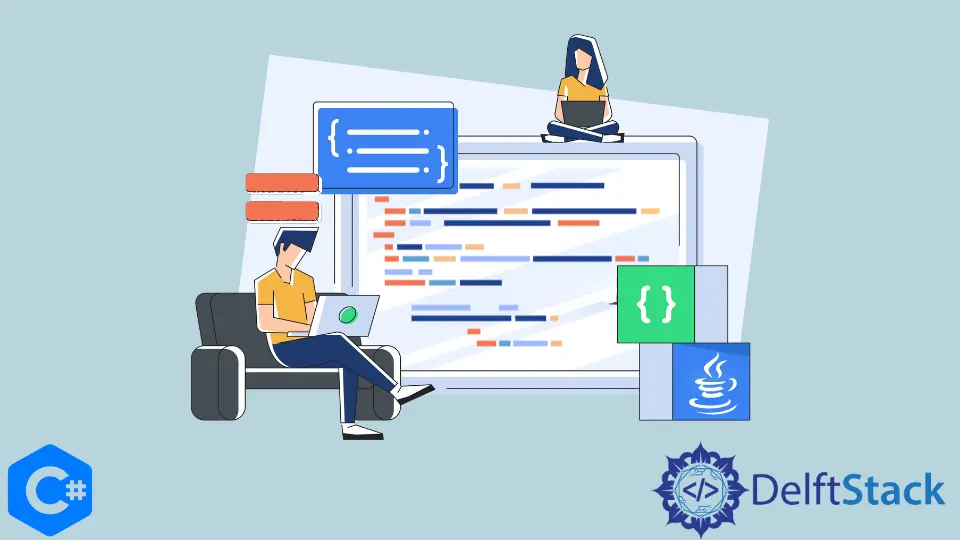
이 문서에서는 C#을 사용하여 할당 연산자를 오버로드하는 방법을 설명합니다. 먼저 연산자 오버로딩에 대해 살펴보겠습니다.
C#
의 연산자 오버로딩
내장 연산자를 재정의하는 방법을 연산자 오버로딩이라고 합니다. 피연산자 중 하나 또는 둘 다 사용자 정의 유형인 경우 다양한 작업의 사용자 정의 구현을 만들 수 있습니다.
암시적 변환 연산자를 사용하여 C#
에서 할당 연산자 오버로딩 구현
암시적 변환 작업을 개발할 수 있습니다. 불변 구조체로 만드는 것은 또 다른 현명한 조치입니다.
프리미티브는 바로 그것이며, 그것이 그들로부터 상속하는 것을 불가능하게 만드는 것입니다.
추가 및 기타 연산자와 함께 아래 예제에서 암시적 변환 연산자를 개발합니다.
-
시작하려면 다음 라이브러리를 가져옵니다.
using System; using System.Collections.Generic; using System.Linq; using System.Text.RegularExpressions;
-
Velocity
라는 구조체를 만들고value
라는 이중 변수를 만듭니다.public struct Velocity { private readonly double value; }
-
Velocity
에게 변수를 매개변수로 받는 공개Velocity
를 생성하도록 지시합니다.public Velocity(double value) { this.value = value; }
-
이제 암시적 연산자인
Velocity
를 만듭니다.public static implicit operator Velocity(double value) { return new Velocity(value); }
-
그런 다음 오버로드할
더하기
및빼기
연산자를 만듭니다.public static Velocity operator +(Velocity first, Velocity second) { return new Velocity(first.value + second.value); } public static Velocity operator -(Velocity first, Velocity second) { return new Velocity(first.value - second.value); }
-
마지막으로
Main()
메서드에서Velocity
객체를 호출하여 오버로드합니다.static void Main() { Velocity v = 0; v = 17.4; v += 9.8; }
완전한 소스 코드:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text.RegularExpressions;
public struct Velocity {
private readonly double value;
public Velocity(double value) {
this.value = value;
}
public static implicit operator Velocity(double value) {
return new Velocity(value);
}
public static Velocity operator +(Velocity first, Velocity second) {
return new Velocity(first.value + second.value);
}
public static Velocity operator -(Velocity first, Velocity second) {
return new Velocity(first.value - second.value);
}
}
class Example {
static void Main() {
Velocity v = 0;
v = 17.4;
v += 9.8;
}
}
작가: Muhammad Zeeshan
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn