C#에서 CPU 사용량 가져오기
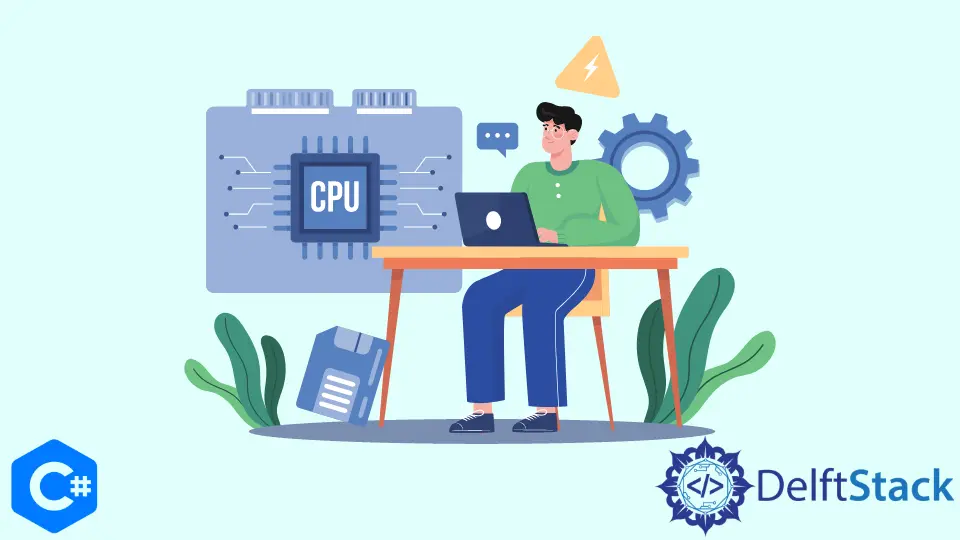
이 자습서에서는 프로그램에 영향을 미치는 성능 모니터링 매개변수에 대해 자세히 설명합니다. 또한 특히 C#에서 CPU 및 메모리 사용 방법을 시연합니다.
C#
의 성능 모니터링
응용 프로그램/프로그램의 성능은 시스템의 메모리와 프로세서를 소비하므로 매우 중요합니다. 프로그램의 성능은 이러한 매개변수 값을 효율적으로 추적하고 사용자 지정하여 최적화할 수 있습니다.
시스템의 프로세서는 대부분 시스템의 CPU를 암시합니다. C#에서는 단일 프로세스 및 전체 프로세서의 CPU 사용량을 추적할 수 있습니다.
메모리 카운터는 메모리 관리 문제를 추적하는 데 사용할 수 있습니다. System.Diagnostics
네임스페이스는 모든 성능 카운터 매트릭스를 구현하는 PerformanceCounter
클래스를 제공합니다.
이 네임스페이스를 가져오고 PerformanceCounter
클래스 메서드를 사용하여 시스템 사용을 진단할 수 있습니다. 다음은 일부 성능 카운터와 해당 설명입니다.
개체
가 성능 모니터이고 카운터는 PerformanceCounter
클래스에서 지원하는 카운터의 이름인 다음 카운터를 등록할 때 개체 이름: 카운터 - 설명
형식을 사용합니다.
프로세서 성능
-
Processor
:% Processor Time
- 이 카운터는 프로세서가 유용한 스레드 작업에 적극적으로 할애하는 시간과 요청에 응답하는 데 사용된 빈도를 나타냅니다.이 카운터는 시스템이 비활성화된 시간을
100%
로 나눈 비율을 계산합니다. 프로세서는 절대 유휴 상태가 아니므로 이 매개변수를 쉽게 계산할 수 있습니다. 항상 할 일이 있습니다. -
Processor
:% User Time
- 이 카운터의 값은 시스템에 영향을 미치는 처리 유형을 식별하는 데 도움이 됩니다. 결과는사용자
모드 작업에 사용된 생산 시간의 전체 값입니다. 일반적으로 이것은 프로그램 코드를 나타냅니다. -
프로세서
:% 인터럽트 시간
-인터럽트
를 처리하는 데 사용되는 프로세서 시간을 보여줍니다. -
Process
:% Processor Time
- 이 카운터는 이 특정 프로세스가 프로세서를 사용하는 시간 값을 제공합니다. -
System
:% Total Processor Time
- 이 카운터는 모든 프로세서의 활동을 결합하여 전체 시스템 성능을 제공합니다. 이 값은 단일 프로세서 시스템에서%Processor Time
과 동일합니다.
메모리 성능
메모리
:사용 가능한 메모리
- 이 숫자는 페이징 풀 할당, 비페이징 풀 할당, 프로세스 작업 집합 및 파일 시스템 캐시가 완료된 후에도 여전히 사용 가능한 메모리 양을 보여줍니다.Memory
:Committed Bytes
- 이 카운터는 Windows NT 프로세스 또는 서비스에서 사용하기 위한 할당된 메모리 양을 표시합니다.Memory
:Page Faults/sec
- 이 카운터는 페이지 오류 수를 지정합니다. 페이지 오류는 필요한 정보가 캐시 또는 가상 메모리에 없는 경우입니다.Memory
:Pages/sec
- 이 카운터는 시스템이 메모리 관련 데이터를 저장하거나 검색하기 위해 하드 드라이브에 액세스하는 빈도를 보여줍니다.Process
:Working Set
- 프로세스가 코드, 스레드 및 데이터에 사용하는 현재 메모리 영역 크기를 보여줍니다.
C#
의 성능 모니터링 카운터 데모
성능 모니터링 카운터를 시연하기 위해 C# 예제 코드를 생성합니다. Visual Studio 또는 Visual Studio Code를 사용하여 C# 빈 프로젝트를 만들어 보겠습니다.
첫 번째 단계는 Diagnostics
네임스페이스를 프로젝트로 가져오는 것입니다. 다음 코드를 사용하여 가져옵니다.
using System.Diagnostics;
System.Diagnostics.PerformanceCounter
클래스의 개체를 만들어야 합니다. 다음과 같이 두 개의 객체를 만듭니다. 하나는 메모리 사용 기록용이고 다른 하나는 CPU 사용용입니다.
protected static PerformanceCounter CPUCounter;
protected static PerformanceCounter memoryCounter;
다음 단계는 이러한 개체를 초기화하는 것입니다. C#에서 두 가지 방법을 사용하여 초기화할 수 있습니다.
-
개체 생성 시 매개 변수 값을 전달하여 개체를 초기화할 수 있습니다. 이 메서드에서는 기본 생성자를 호출할 필요가 없습니다.
예를 들어:
protected static PerformanceCounter CPUCounter = new PerformanceCounter(Object Name, Counter, Instance); protected static PerformanceCounter memoryCounter = new PerformanceCounter(Category Name, Counter);
-
다른 방법은
new
키워드와 기본 생성자를 사용하여 개체를 초기화하는 것입니다. 매개 변수 값은 점 식별자를 사용하여 변수에 액세스하도록 설정됩니다.예를 들어:
// Initialize the objects CPUCounter = new PerformanceCounter(); memoryCounter = new PerformanceCounter(); // Assign values CPUCounter.CategoryName = Object Name; CPUCounter.CounterName = Counter; CPUCounter.InstanceName = Instance; memoryCounter.CategoryName = Object Name; memoryCounter.CounterName = Counter;
초기화 후 다음 단계는 값을 계산하는 것입니다.
NextValue()
메서드는 카운터 값을 가져오는 데 사용됩니다.위에서 만든 각 개체를 사용하여 이러한 메서드를 호출해야 합니다.
Console.WriteLine(CPUCounter.NextValue());
총 CPU 및 메모리 사용량 검색
다음은 전체 CPU 및 메모리 사용량을 가져오는 전체 코드입니다.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Diagnostics;
namespace ConsoleApplication1 {
class Program {
// create objects
protected static PerformanceCounter CPUCounter;
protected static PerformanceCounter memoryCounter;
static void Main(string[] args) {
// initialize objects
CPUCounter = new PerformanceCounter();
memoryCounter = new PerformanceCounter();
// assign parameters
CPUCounter.CategoryName = "Processor";
CPUCounter.CounterName = "% Processor Time";
CPUCounter.InstanceName = "_Total";
memoryCounter.CategoryName = "Memory";
memoryCounter.CounterName = "Available MBytes";
// invoke the following monitoring methods
getCpuUsage();
getMemory();
Console.ReadKey();
}
// prints the value of total processor usage time
public static void getCpuUsage() {
Console.WriteLine(CPUCounter.NextValue() + "%");
}
// prints the value of available memory
public static void getMemory() {
Console.WriteLine(memoryCounter.NextValue() + "MB");
}
}
}
위 코드 스니펫의 출력은 %
의 CPU 사용량과 MB
의 사용 가능한 메모리입니다.
현재 프로세스의 CPU 및 메모리 사용량 검색
다음 코드 스니펫을 사용하여 현재 프로세스의 CPU 및 메모리 사용량을 가져올 수 있습니다.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Diagnostics;
namespace ConsoleApplication1 {
class Program {
protected static PerformanceCounter CPUCounter;
protected static PerformanceCounter memoryCounter;
static void Main(string[] args) {
CPUCounter = new PerformanceCounter("Process", "% Processor Time",
Process.GetCurrentProcess().ProcessName);
memoryCounter = new PerformanceCounter("Memory", "Available MBytes");
getCpuUsage();
getMemory();
Console.ReadKey();
}
public static void getCpuUsage() {
Console.WriteLine(CPUCounter.NextValue() + "%");
}
public static void getMemory() {
Console.WriteLine(memoryCounter.NextValue() + "MB");
}
}
}
위 코드 스니펫의 출력은 현재 프로세스에서 사용하는 프로세서와 시스템의 사용 가능한 메모리입니다.
특정 애플리케이션의 CPU 및 메모리 사용량 검색
특정 애플리케이션의 CPU 사용량을 확인하려면 PerformanceCounter
생성자에서 애플리케이션 이름을 인스턴스로 사용할 수 있습니다.
PerformanceCounter("Process", "% Processor Time", app_name);
다음은 특정 앱의 CPU 사용량을 가져오는 전체 예제 코드입니다.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Diagnostics;
namespace ConsoleApplication1 {
class Program {
static void Main(string[] args) {
CPUUsagebyApp("chrome");
}
private static void CPUUsagebyApp(string app_name) {
PerformanceCounter totalCPUUsage =
new PerformanceCounter("Processor", "% Processor Time", "_Total");
PerformanceCounter processCPUUsage =
new PerformanceCounter("Process", "% Processor Time", app_name);
// loop will be executed while you don't press the enter key from the keyboard
// you have to press space or any other key to iterate through the loop.
do {
float total = totalCPUUsage.NextValue();
float process = processCPUUsage.NextValue();
Console.WriteLine(
String.Format("App CPU Usage = {0} {1}%", total, process, process / total * 100));
System.Threading.Thread.Sleep(1000);
} while (Console.ReadKey().Key != ConsoleKey.Enter);
}
}
}
이 코드 스니펫의 출력은 "chrome"
을 매개변수로 전달한 Chrome의 총 CPU 사용량 및 CPU 사용량입니다. [Performance Monitoring in C#]?redirectedfrom=MSDN)을 읽고 깊이 있게 이해할 수 있습니다.