C#에서 SOAP 서비스 만들기
- SOAP에 대한 간략한 설명
- SOAP 웹 서비스
- C#에서 .NET Core를 사용하여 SOAP 서비스 호출
-
C#에서
SoapClientMessage
클래스 사용 - C#에서 ASP.NET Core를 사용하여 SOAP 서비스 만들기
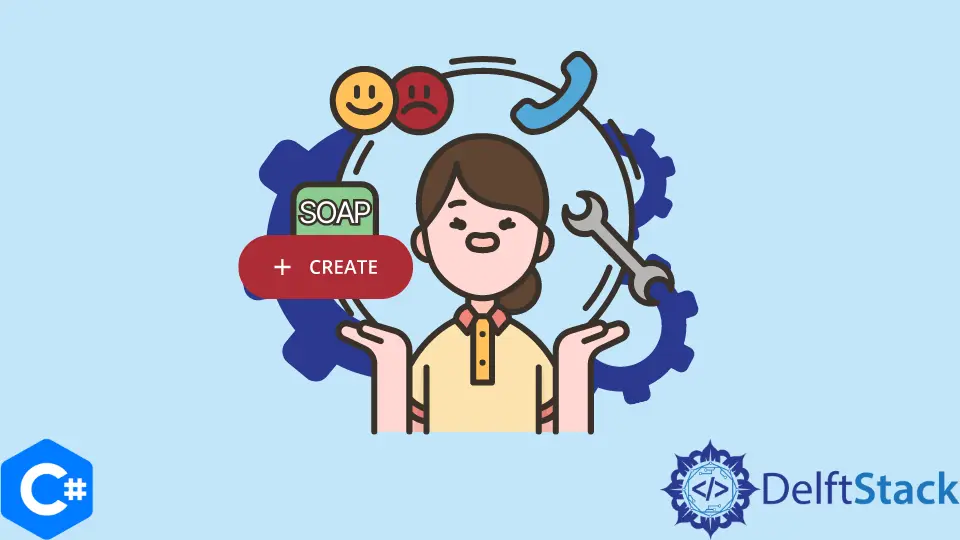
ASP.NET Core에서 SOAP 또는 WCF를 활용하려는 경우 혼자가 아닙니다. .NET Core에서 가장 원하고 많이 찾는 기능 중 하나입니다.
이 게시물에서는 .NET Core 애플리케이션에서 WCF SOAP 서비스를 사용하는 방법을 살펴보겠습니다. 또한 ASP.NET Core를 사용하여 SOAP 서비스를 설정하고 호스팅하는 방법도 보여줍니다.
SOAP에 대한 간략한 설명
SOAP(Simple Object Access Protocol)는 분산되어 있고 다양한 시스템 간에 구조화된 데이터를 전송하기 위한 프로토콜입니다. 통신 형식은 XML이며 HTTP와 같은 응용 프로그램 계층 프로토콜에 의존합니다.
대부분의 사람들은 온라인 서비스의 표준 프로토콜로 익숙합니다.
SOAP는 최근까지 웹 서비스 구축을 위한 사실상의 표준이었으며 SOA(Service Oriented Architecture)도 이에 크게 의존했습니다. SOAP는 따라야 하는 메시지 형식을 설정하지만 그 안에 원하는 것을 포함할 수 있습니다. 이진 첨부 파일도 사용할 수 있습니다.
SOAP 표준은 헤더(header >
)와 본문(body >
)을 포함할 수 있는 포함된 봉투(envelope >
)를 제공하며, 후자는 오류 섹션(fault >
)을 포함할 수 있습니다. 뿐만 아니라 다른 하위 섹션. body >
만 필요하고 fault >
는 오류가 발생한 요청이 아니라 응답을 위해 예약되어 있습니다.
메시지 중에 이진 자료의 일부를 통합하는 기능을 MTOM(Message Transmission Optimization Mechanism)이라고 하며, Base-64를 사용하여 이진 스트림을 인코딩하여 30% 더 크게 만드는 대안입니다.
SOAP 웹 서비스
온라인 서비스를 호출할 때 본문에는 수행할 작업과 제공될 수 있는 모든 인수가 포함되어야 합니다. POST는 항상 SOAP 웹 서비스에서 사용되며 봉투는 항상 하나의 잘 알려진 URL에 페이로드로 전송됩니다.
웹 서비스 프레임워크는 요청을 특정 시스템 클래스 및 기능으로 라우팅합니다.
웹 서비스는 각 작업 메서드의 이름, 인수 및 반환 값, 예상 오류와 같은 서비스에 대한 정보가 포함된 WSDL(웹 서비스 정의 언어) 파일을 사용할 수 있도록 합니다. Visual Studio(및 SVCUtil.exe 명령줄 프로그램과 같은 기타 도구)는 이 WSDL을 사용하여 웹 서비스와 인터페이스하는 코드 프록시를 생성할 수 있습니다.
C#에서 .NET Core를 사용하여 SOAP 서비스 호출
결과적으로 .NET Core를 사용하여 클라이언트 측에서 WCF를 활용하려는 경우 가능합니다. 먼저 System.ServiceModel
을 포함했는지 다시 확인하십시오.
시스템의 기본 ServiceModel.Http
또는 System(BasicHttpBinding
/BasicHttpsBinding
또는 NetHttpBinding
/NetHttpsBinding
바인딩, SOAP 및 REST를 포함) 중 하나입니다. ServiceModel.NetTcp
는 보내고 받을 수 있는 프로토콜입니다(NetTcpBinding
의 경우 Windows 전용 바이너리 프로토콜).
기본적인 예를 살펴보겠습니다.
첫째, 계약:
public interface IPServ {
string P(string msg);
}
그런 다음 클라이언트 코드:
var bind = new BasicHttpBinding();
var endp = new EndpointAddress(new Uri("[url]"));
var cF = new ChannelFactory(bind, endp);
var servC = cF.CreateChannel();
var res = servC.Ping("Ping");
cF.Close();
보시다시피 완전한 .NET 프레임워크에서 작업하는 경우와 모든 것이 동일합니다. App.config
또는 Web.config
파일이 없으므로 올바른 바인딩 및 끝점 주소 생성과 같은 모든 것을 수동으로 구성해야 합니다.
svcutil.exe
프로그램을 사용하여 .NET Core 호환 프록시 클래스를 수동으로 생성할 수도 있습니다. 이렇게 하면 .NET Core를 사용할 수 있습니다.
SOAP로 ASP.NET Core를 구성하는 것이 얼마나 쉬운지를 고려할 때 SOAP로 구성하는 것이 얼마나 어려운지 간과하기 쉽습니다.
C#에서 SoapClientMessage
클래스 사용
XML 웹 서비스 클라이언트에서 전달 및 수신한 SOAP 메시지를 기록하는 SOAP 확장에는 다음 코드 세그먼트가 포함됩니다. SoapExtension으로 전송된 SoapClientMessage
는 이 조각에 의해 처리됩니다.
SOAP 확장의 ProcessMessage
기능은 SoapClientMessage
속성을 로그 파일에 씁니다.
// Process the SOAP message received and write it to a log file.
public override void PM(SoapMessage m) {
switch (m.Stage) {
case SoapMessageStage.BeforeSerialize:
break;
case SoapMessageStage.AfterSerialize:
WriteOutput((SoapClientMessage)m);
break;
case SoapMessageStage.BeforeDeserialize:
WriteInput((SoapClientMessage)m);
break;
case SoapMessageStage.AfterDeserialize:
break;
default:
throw new Exception("this is and invalid state");
}
}
출력하기 위해 파일로 나가는 SOAP 메시지의 로그를 로그 파일이라고 합니다.
public void WO(SoapClientMessage m) {
newStream.Position = 0;
FileStream mf = new FileStream(filename, FileMode.Append, FileAccess.Write);
StreamWriter ms = new StreamWriter(mf);
ms.WriteLine("--> Requested at " + DateTime.Now);
// Print to the log file the field of request header for header SoapAction .
ms.WriteLine("The Http SoapAction header request field is: ");
ms.WriteLine("\t" + m.Action);
// Write the client type that called the XML Web service method to the log file.
ms.WriteLine("The client type is: ");
if ((m.Client.GetType()).Equals(typeof(MathSvc)))
ms.WriteLine("\tMathSvc");
// The client's technique will be printed to the log file.
ms.WriteLine("The approach that the client has requested is:");
ms.WriteLine("\t" + m.MethodInfo.Name);
// The client's technique will be printed on the log file.
if (m.OneWay)
ms.WriteLine("The client does not wait for the server to complete the task.");
else
ms.WriteLine("The client waits for the server to complete the task.");
// The URL of the site that implements the method is printed to the log file.
ms.WriteLine("The XML Web service method that has been requested has the following URL: ");
ms.WriteLine("\t" + m.Url);
ms.WriteLine("The SOAP envelope's contents are as follows: ");
ms.Flush();
// Copy one stream's content to another.
Copy(newStream, mf);
mf.Close();
newStream.Position = 0;
// Copy one stream's content to another.
Copy(newStream, oldStream);
}
C#에서 ASP.NET Core를 사용하여 SOAP 서비스 만들기
예를 들어 클라이언트 측의 경우 이전에 보여준 것과 동일한 클래스를 사용할 수 있습니다. 서버 측에서 계약을 구현하는 서비스 클래스를 개발해야 합니다.
public class SS : IPServ {
public string P(string m) {
return string.Join(string.Empty, m.Reverse());
}
}
이제 다음 주소에서 서비스 구현을 등록하기만 하면 됩니다.
public void ConfigServ(IServColl s) {
s.AddSingleton(new PingService());
s.AddMvc();
// rest code goes here
}
public void Conf(IApplicationBuilder app, IHostingEnvironment env, ILoggerFactory loggerFactory) {
app.UseSoapEndpoint(path: "/PingService.svc", binding: new BasicHttpBinding());
app.UseMvc();
// rest code goes here
}
다음과 같은 경우에 발생해야 하는 예외에서 의미 있는 메시지를 추출하고 클라이언트에 오류 문자열로 제공하기 위해 사용자 지정 예외-문자열 변환기를 구현할 수 있습니다.
s.AddSoapExceptionTransformer((ex) = > ex.Message);
이 코드는 HTTP POST를 수신 대기하고 유효한 헤더를 확인하는 ASP.NET Core 파이프라인에 사용자 지정 미들웨어를 주입합니다. 지정된 구현 클래스로 전달하기 전에 페이로드에서 작업 메서드와 매개변수를 추출합니다.
고려해야 할 몇 가지 사항
.svc
확장자는 필요하지 않습니다. WCF에 이전에 필요했음을 상기시키기 위한 것일 뿐입니다.PingService
의 경우 싱글톤뿐만 아니라 모든 라이프 사이클을 활용할 수 있습니다.- 클라이언트와 서버 바인딩은 동일해야 합니다.
- ASP.NET Core 필터는 무시되므로 사용할 수 없지만 대신 종속성 주입을 사용할 수 있습니다.
이전 버전과의 호환성을 위해 이 코드를 확실히 사용할 수 있지만 .NET Core에서 SOAP 서비스를 작성해야 하는 경우(예: Docker에 배포해야 하는 경우) 다음 제약 조건에 유의하십시오.
- WS - *표준은 지원되지 않습니다.
- MTOM은 지원하지 않습니다.
- HTTPS 및 ASP.NET Core의 기본 제공 보안 외에 보안에 대한 지원이 없습니다.
- 조절이나 부적절한 행동이 없을 것입니다.
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn