C#의 AES 암호화
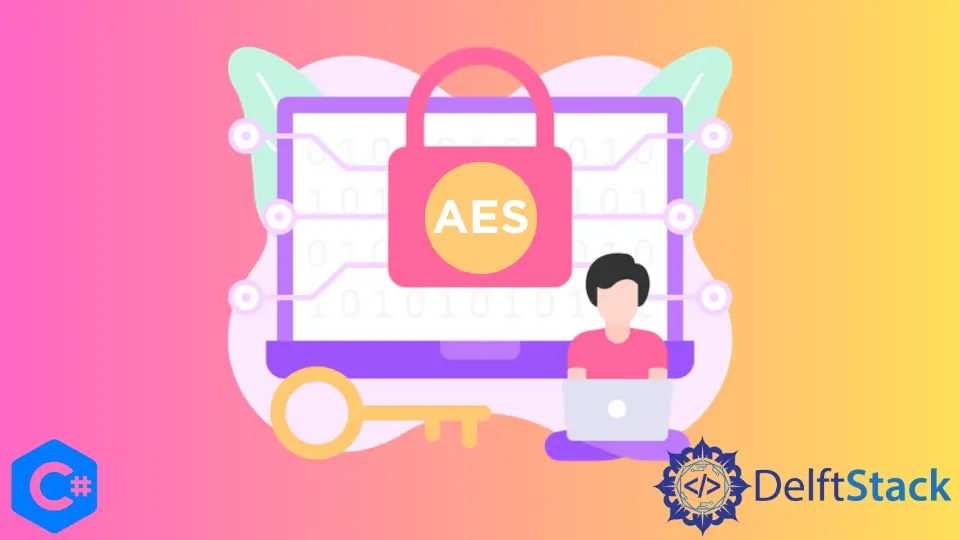
이 가이드에서는 C#의 AES 암호화에 대해 알아봅니다.
C#
의 AES 암호화
우리가 이야기하는 알고리즘은 AES 또는 Advanced Encryption Standard입니다. 이 대칭적으로 암호화된 알고리즘은 동일한 키를 사용하여 데이터를 암호화하거나 해독할 수 있는 발신자와 수신자 권한을 모두 부여합니다.
이 알고리즘은 3비트 암호화 모드를 지원합니다. 128, 192 및 256비트 암호화.
AES는 우리가 암호화된 키를 생성하는 데 도움을 주고 사용할 절실히 필요한 클래스와 기능을 제공합니다. 정부 및 민간 산업은 고급 암호화 표준(AES)을 테스트했습니다.
C#
에서 AES 암호화 작업
라이브러리 System.Security.Cryptography
는 암호화된 키를 만드는 데 필요한 모든 방법에 사용됩니다. 이제 이 라이브러리인 CryptoStream
및 MemoryStream
을 통해 사용되는 클래스에 대해 논의하겠습니다.
CryptoStream
은 위에서 언급한 모드의 모든 스트림을 저장합니다. 컴파일러가 모드를 지원하는 경우 모든 스트림을 암호화하고 해독할 수 있습니다.
MemoryStream
클래스는 클래스가 스트림을 구현하기 위해 저장할 때 사용됩니다. 이 경우 CryptoStream
이 사용 중이므로 MemoryStream
이 스트림을 구현합니다.
다음으로 사용되는 클래스는 AES 알고리즘을 관리하는 AesManaged
입니다. 마지막으로 사용된 클래스는 StreamWriter
이며 스트림에 문자를 씁니다. 따라서 AES 암호화 작업이 필요한 경우 이 클래스를 사용하는 것이 필수적입니다.
코드로 이동하기 전에 예외 처리를 사용하는 것이 중요합니다.
코드 - 메인 클래스:
using System;
using System.IO;
using System.Security.Cryptography;
namespace ConsoleApp1 {
class Program {
static void Main(string[] args) {
Console.WriteLine("Write any string to encrypt: ");
string text = Console.ReadLine();
EncryptedDATA(text); // write text to be encrypted
Console.Read();
}
}
}
코드 - 사용자로부터 문자열 입력을 받는 함수:
static void EncryptedDATA(string TextToEncrypt) {
try {
using (AesManaged dataEncrypt = new AesManaged()) {
byte[] TextEncrypted = Encrypt(TextToEncrypt, dataEncrypt.Key, dataEncrypt.IV);
// passing the string data inside the Encrypt() function
string myLine = $"the data encrypted is:{System.Text.Encoding.UTF8.GetString(TextEncrypted)}";
Console.WriteLine(myLine); // printing the encrypted data
}
} catch (Exception EX) // exception handling
{
Console.WriteLine(EX.Message);
}
}
코드 - 데이터를 암호화하는 기능:
static byte[] Encrypt(string TextToEncrypt, byte[] encrypt, byte[] IV) {
byte[] EncryptedText;
using (AesManaged AESencrypt = new AesManaged()) // using a AesManaged function
{
ICryptoTransform DataEncrypt =
AESencrypt.CreateEncryptor(encrypt, IV); // here we are creating an encryptor
using (MemoryStream memory = new MemoryStream()) // using a MemoryStream function
{
using (CryptoStream crypto = new CryptoStream(memory, DataEncrypt, CryptoStreamMode.Write))
// using a CryptoStream function to store and write stream
{
{
using (StreamWriter stream = new StreamWriter(crypto))
stream.Write(TextToEncrypt); // wrting stream
EncryptedText = memory.ToArray(); // storing stream
}
}
}
return EncryptedText;
}
AES 암호화로 작동하는 동안 오류가 나타날 수 있습니다. 이러한 오류를 처리하기 위해 예외 처리를 사용합니다.
전체 소스 코드:
using System;
using System.IO;
using System.Security.Cryptography;
class Program {
static void Main(string[] args) {
Console.WriteLine("Write any string to encrypt: ");
string text = Console.ReadLine();
EncryptedDATA(text); // write text to be encrypted
Console.Read();
}
static void EncryptedDATA(string TextToEncrypt) {
try {
using (AesManaged dataEncrypt = new AesManaged()) {
byte[] TextEncrypted = Encrypt(TextToEncrypt, dataEncrypt.Key, dataEncrypt.IV);
// passing the string data inside the Encrypt() function
string myLine =
$"the data encrypted is: {System.Text.Encoding.UTF8.GetString(TextEncrypted)}";
Console.WriteLine(myLine); // printing the encrypted data
}
} catch (Exception EX) // exception handling
{
Console.WriteLine(EX.Message);
}
}
static byte[] Encrypt(string TextToEncrypt, byte[] encrypt, byte[] IV) {
byte[] EncryptedText;
using (AesManaged AESencrypt = new AesManaged()) // using a AesManaged function
{
ICryptoTransform DataEncrypt =
AESencrypt.CreateEncryptor(encrypt, IV); // here we are creating an encryptor
using (MemoryStream memory = new MemoryStream()) // using a MemoryStream function
{
using (
CryptoStream crypto = new CryptoStream(
memory, DataEncrypt,
CryptoStreamMode.Write)) // using a CryptoStream function to store and write stream
{
{
using (StreamWriter stream = new StreamWriter(crypto))
stream.Write(TextToEncrypt); // wrting stream
EncryptedText = memory.ToArray(); // storing stream
}
}
}
return EncryptedText;
}
}
}
출력:
Write any string to encrypt:
Hello
the data encrypted is: ??*?2?@???W@G?
위의 코드는 모든 메시지를 암호화합니다. 데이터가 암호화되면 사용자만 액세스할 수 있습니다.
이 데이터를 읽는 방법은 해독하는 것입니다. 그런 다음에만 데이터가 표시됩니다.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn