C++의 boost::split 함수
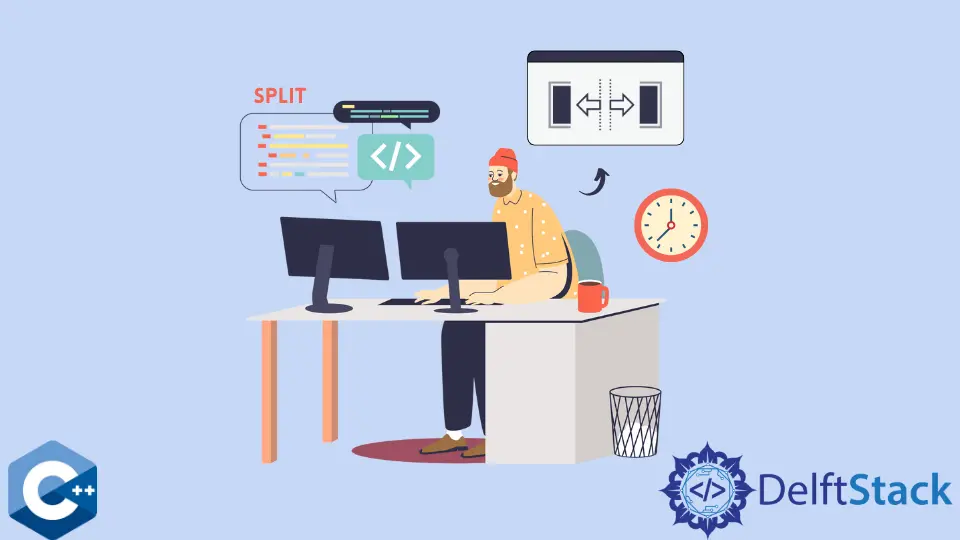
이 기사에서는 C++에서 boost::split
함수를 사용하는 방법을 보여줍니다.
boost::split
함수를 사용하여 주어진 문자열 토큰화
Boost는 성숙하고 잘 테스트된 라이브러리로 C++ 표준 라이브러리를 확장하는 강력한 도구를 제공합니다. 이 기사에서는 Boost 문자열 알고리즘 라이브러리의 일부인 boost::split
함수를 살펴봅니다. 후자는 트리밍, 교체 등과 같은 여러 문자열 조작 알고리즘을 포함합니다.
boost::split
함수는 주어진 문자열 시퀀스를 구분 기호로 구분된 토큰으로 분할합니다. 사용자는 구분자를 세 번째 매개변수로 식별하는 술어 함수를 제공해야 합니다. 주어진 요소가 구분 기호인 경우 제공된 함수는 true
를 반환해야 합니다.
다음 예에서는 isspace
함수 객체를 지정하여 주어진 텍스트에서 공백을 식별하고 이를 토큰으로 분할합니다. boost::split
에는 토큰화된 하위 문자열을 저장할 대상 시퀀스 컨테이너도 필요합니다. 대상 컨테이너가 첫 번째 매개변수로 전달되고 함수 호출 후 이전 내용을 덮어씁니다.
#include <boost/algorithm/string/split.hpp>
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
string text = "Lorem ipsum dolor sit amet, consectetur adipiscing elit.";
vector<string> words;
boost::split(words, text, isspace);
for (const auto &item : words) {
cout << item << "; ";
}
cout << endl;
return EXIT_SUCCESS;
}
출력:
Lorem; ipsum; ; dolor; sit; amet,; consectetur; adipiscing; elit.;
이전 코드 조각의 boost::split
호출은 두 개 이상의 구분 기호가 서로 옆에 있을 때 빈 문자열을 저장합니다. 그러나 네 번째 선택적 매개변수인 boost::token_compress_on
을 지정할 수 있으며 다음 예와 같이 인접한 구분 기호가 병합됩니다. 이 두 코드 조각을 성공적으로 실행하려면 Boost 라이브러리가 시스템에 설치되어 있어야 합니다.
#include <boost/algorithm/string/split.hpp>
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::stringstream;
using std::vector;
int main() {
string text = "Lorem ipsum dolor sit amet, consectetur adipiscing elit.";
vector<string> words;
boost::split(words, text, isspace, boost::token_compress_on);
for (const auto &item : words) {
cout << item << "; ";
}
cout << endl;
return EXIT_SUCCESS;
}
출력:
Lorem; ipsum; dolor; sit; amet,; consectetur; adipiscing; elit.;
stringstream
을 getline
함수와 함께 사용하여 구분 기호로 문자열 분할
또는 stringstream
클래스와 getline
함수를 사용하여 주어진 문자 구분 기호로 텍스트를 분할할 수 있습니다. 이 경우 STL 도구만 활용하며 Boost 헤더를 포함할 필요가 없습니다. 이 코드 버전은 부피가 더 크며 인접한 구분 기호 문자를 병합하려면 추가 단계가 필요합니다.
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::stringstream;
using std::vector;
int main() {
string text = "Lorem ipsum dolor sit amet, consectetur adipiscing elit.";
vector<string> words;
char space_char = ' ';
stringstream sstream(text);
string word;
while (std::getline(sstream, word, space_char)) {
words.push_back(word);
}
for (const auto &item : words) {
cout << item << "; ";
}
cout << endl;
return EXIT_SUCCESS;
}
출력:
Lorem; ipsum; ; dolor; sit; amet,; consectetur; adipiscing; elit.;
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook