C++에서 반복자 역참조
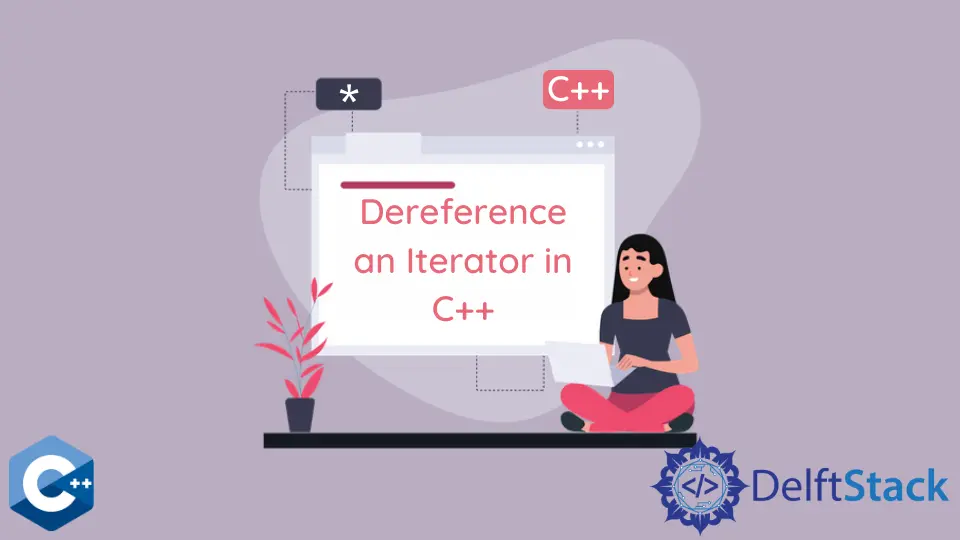
Iterator는 트리와 같은 복잡한 데이터 구조를 반복하는 데 도움이 되는 C++의 또 다른 강력한 메커니즘이며 배열과 같이 내부에 요소의 인덱스가 없는 곳을 설정합니다.
C++에서 반복자란?
C++에서 반복자는 배열, 목록 및 기타 데이터 구조 내의 요소를 가리키는 포인터와 같은 객체입니다. 반복자를 사용하여 컨테이너 요소를 반복합니다. 기존 루프를 사용할 수 있지만 반복자는 컨테이너를 반복하는 데 우위를 가집니다.
컨테이너의 요소를 순회하는 매우 일반적인 접근 방식을 제공합니다.
배열과 같은 일반적인 데이터 구조에서는 인덱스 변수를 사용하여 컨테이너를 반복할 수 있지만 트리 또는 정렬되지 않은 데이터 구조와 같이 반복할 인덱스 변수가 없는 고급 데이터 구조에서는 반복자를 사용하여 탐색합니다. .
컨테이너는 반복자에게 데이터 유형을 제공하고 컨테이너 클래스는 반복자가 컨테이너의 요소를 반복하는 데 도움이 되는 두 가지 기본 기능을 제공합니다.
Begin()
- 컨테이너의 첫 번째 요소를 반환합니다.End()
- 컨테이너의 마지막 요소 다음을 반환합니다.
적절한 코드 예제를 통해 이해해 봅시다.
#include <iostream>
#include <vector>
using namespace std;
int main() {
// Declaring a vector
vector<int> numbers = {1, 2, 3, 4, 5};
// Declaring an iterator
vector<int>::iterator i;
int j;
cout << "Traversing using loop = ";
// Accessing the elements using loops
for (j = 0; j < 5; ++j) {
cout << numbers[j] << ", ";
}
cout << endl << "Traversing using Iterators = ";
// Accessing the elements using iterators
for (i = numbers.begin(); i != numbers.end(); ++i) {
cout << *i << ", ";
}
// Adding another element to the vector
numbers.push_back(6);
cout << endl << "Traversing using loops after adding new value to numbers = ";
// After adding a new value to the numbers vector
for (j = 0; j < 5; ++j) {
cout << numbers[j] << ", ";
}
cout << endl
<< "Traversing using iterators after adding new value to numbers = ";
// Accessing the elements using iterators
for (i = numbers.begin(); i != numbers.end(); ++i) {
cout << *i << ", ";
}
return 0;
}
출력:
Traversing using loop = 1, 2, 3, 4, 5,
Traversing using Iterators = 1, 2, 3, 4, 5,
Traversing using loops after adding new value to numbers = 1, 2, 3, 4, 5,
Traversing using iterators after adding new value to numbers = 1, 2, 3, 4, 5, 6,
이 프로그램은 루프와 반복자를 사용하여 컨테이너를 통과하는 사용법의 차이점을 보여줍니다. 위의 코드에서 반복자는 코드를 추적하고 코드가 변경되면 동적으로 채택합니다.
루프에 있는 동안 루프에 대한 코드를 정적으로 업데이트해야 합니다. 반복자는 코드 재사용성 요소를 달성하고 있습니다.
반복자에서 begin()
함수는 컨테이너의 첫 번째 요소를 가져오고 end()
는 마지막 요소의 다음 요소를 가져옵니다.
C++의 역참조
C++의 역참조 연산자는 포인터가 가리키는 메모리 위치의 데이터에 액세스하거나 데이터를 조작하는 데 사용됩니다. *
별표 기호는 포인터 변수를 구별할 때 포인터 변수와 함께 사용되며 포인터의 역참조라고 하는 포인터 변수를 참조합니다.
코드 예:
#include <iostream>
using namespace std;
int main() {
int a = 9, b;
int *p; // Un-initialized Pointer
p = &a; // Store the address of a in pointer p
b = *p; // Put the Value of the pointer p in b
cout << "The value of a = " << a << endl;
cout << "The value of pointer p = " << *p << endl;
cout << "The value of b = " << b << endl;
}
출력:
The value of a = 9
The value of pointer p = 9
The value of b = 9
처음에는 a
에 9를 할당한 다음 포인터 p
를 정의합니다. 다음으로 포인터인 p
에 a
의 주소를 할당하고 마지막에 정수 데이터 유형의 변수 b
에 포인터를 할당합니다.
이제 표시할 때 p
에는 a
의 주소가 있기 때문에 a
, p
및 b
는 9와 동일한 출력을 얻습니다. 이는 a
의 값을 표시하는 데 아무 곳이나 사용할 수 있도록 도와줍니다.
C++에서 반복자를 역참조할 수 없는 이유
C++에서는 end()
함수가 데이터 구조의 유효한 멤버가 아닌 포인터로 반복자와 개체를 반환하기 때문에 반복자를 바로 역참조할 수 없습니다.
끝에서 역참조하면 끝 포인터의 목적이 도달했을 때만 확인하는 것이기 때문에 오류가 발생합니다.
Begin()
은 컨테이너가 비어 있지 않을 때, 즉 이 조건을 만족할 위치를 반환합니다.
v.begin() != v.end() // this condition checks, if the container is empty or not
그러나 마지막 요소에 액세스해야 하는 경우 아래 코드를 사용할 수 있습니다.
vector<object>::const_iterator i = vectorOfObjects.end();
i--;
cout << *i << endl; // this prints the last element of the container
참고로 이 코드는 벡터에 요소가 하나 이상 포함된 경우에만 작동합니다.
코드 예:
#include <iostream>
#include <string>
#include <vector>
using namespace std;
struct Student {
int roll;
string name;
int age;
};
int main() {
Student s1, s2, s3; // Objects of strucu
vector<Student> ListOfStudents;
s1.roll = 1; // populating the data members of Student
s2.roll = 2;
s3.roll = 3;
s1.name = "Mike";
s2.name = "John";
s3.name = "Tom";
s1.age = 15;
s2.age = 16;
s3.age = 14;
ListOfStudents.push_back(s1);
ListOfStudents.push_back(s2);
ListOfStudents.push_back(s3);
vector<Student>::const_iterator iter =
ListOfStudents.begin(); // begining position
while (iter != ListOfStudents.end()) { // if the container is empty or not
cout << "Roll number " << iter->roll << " is " << (*iter).name
<< " and he is " << iter->age << " years old." << endl;
++iter;
}
return 0;
}
출력:
Roll number 1 is Mike and he is 15 years old.
Roll number 2 is John and he is 16 years old.
Roll number 3 is Tom and he is 14 years old.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn