C++의 역행렬
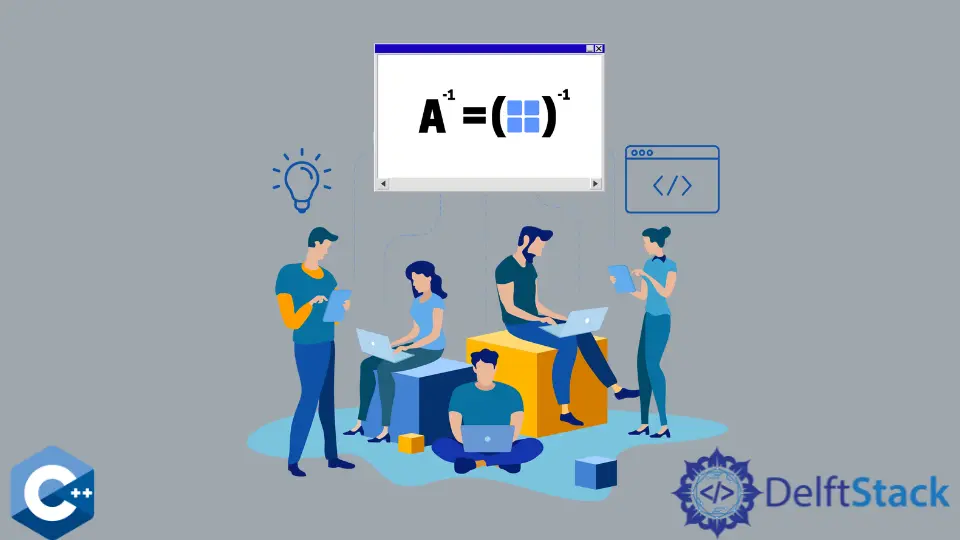
이 기사에서는 역행렬과 C++를 사용한 구현에 대해 설명합니다. C++ 구현을 쉽게 이해하려면 먼저 역행렬의 개념을 이해해야 합니다.
행렬의 역함수
행렬의 역행렬을 찾는 데는 세 단계가 있습니다. 단계에 대한 설명은 다음과 같습니다.
-
첫 번째 단계에서 주어진 행렬의 행렬식을 계산합니다.
-
두 번째 단계에서 결정자가 0이 아닌 경우 주어진 행렬의 adjoint를 계산합니다.
-
마지막으로 2단계에서 얻은 행렬에
1/determinant
를 곱합니다.
역행렬의 수학 공식
S를 3 x 3 행렬이라고 합니다. 그러면 그 역함수를 구하는 공식은 아래와 같습니다.
참고: 주어진 행렬의 결정자가 영(0)인 경우, 즉 행렬이 특이 행렬인 경우 역행렬을 찾을 수 없습니다.
다음은 C++에서 3x3 차수의 역행렬을 찾는 프로그램입니다. 단순성을 위해 행렬을 저장하기 위해 배열을 사용했습니다.
프로그램은 특이 행렬이 아닌 경우에만 행렬의 역행렬을 찾습니다. 다음 속성으로 답을 확인할 수 있습니다.
여기서 I
는 대각선에 항목이 1인 항등 행렬입니다. 위의 속성이 성립한다면 우리의 대답은 참이 될 것입니다. 그렇지 않으면 거짓입니다.
제안된 프로그램은 모듈로 나뉩니다.
3x3 행렬에 대한 역의 C++ 구현
역행렬은 벡터, 템플릿 및 클래스를 사용하여 구현할 수 있습니다. 그러나 단순화를 위해 3x3 행렬의 역을 결정하기 위해 2D 배열을 사용합니다.
그런 다음 솔루션을 일반화하여 NxN 행렬의 역수를 찾을 수 있습니다.
프로그램의 구성 요소를 하나씩 설명하겠습니다.
주요 기능
int main() // main function
{
float matrix3X3[3][3];
int r, c;
float determinant = 0;
for (r = 0; r < 3; r++) {
for (c = 0; c < 3; c++) {
cout << "Enter the value at index [" << r << "][" << c << "] : ";
cin >> matrix3X3[r][c];
}
}
display(matrix3X3);
determinant = findDeterminant(matrix3X3);
cout << "\n\nDeteterminant of the given matrix is : " << determinant;
if (determinant != 0) {
adjoint(matrix3X3);
inverse(matrix3X3, determinant);
} else {
cout << " As the determinant of the given matrix is 0, so we cannot take "
"find it's Inverse :";
}
}
main 함수에서 역행렬을 찾을 수 있는 행렬을 만들었습니다. 그런 다음 조건부 검사를 적용하여 행렬이 특이인지 여부를 확인했습니다.
특이하지 않은 경우 행렬의 역행렬이 함수에 의해 계산됩니다. 다음은 우리가 만든 각 기능에 대한 설명입니다.
디스플레이 기능
void display(float matrix3X3[][3]) {
printf("\nThe Elements of the matrix are :");
for (int r = 0; r < 3; r++) {
cout << "\n";
for (int c = 0; c < 3; c++) {
cout << matrix3X3[r][c] << "\t";
}
}
}
디스플레이 기능은 행렬을 표시하는 데 사용됩니다. 여기서 matrix3X3
은 값을 표시할 행렬입니다.
행렬식 계산 함수
float findDeterminant(float matrix3X3[][3]) {
float det = 0; // here det is the determinant of the matrix.
for (int r = 0; r < 3; r++) {
det = det + (matrix3X3[0][r] *
(matrix3X3[1][(r + 1) % 3] * matrix3X3[2][(r + 2) % 3] -
matrix3X3[1][(r + 2) % 3] * matrix3X3[2][(r + 1) % 3]));
}
return det;
}
findDeterminant
함수는 행렬의 행렬식을 찾는 데 사용됩니다. 여기서 det
는 행렬 matrix3X3
행렬식입니다.
행렬식을 본 후 행렬 역행렬이 가능한지 여부를 결론 지을 수 있습니다.
인접 기능
void adjoint(float matrix3X3[][3]) {
cout << "\n\nAdjoint of matrix is: \n";
for (int r = 0; r < 3; r++) {
for (int c = 0; c < 3; c++) {
cout << ((matrix3X3[(c + 1) % 3][(r + 1) % 3] *
matrix3X3[(c + 2) % 3][(r + 2) % 3]) -
(matrix3X3[(c + 1) % 3][(r + 2) % 3] *
matrix3X3[(c + 2) % 3][(r + 1) % 3]))
<< "\t";
}
cout << endl;
}
}
adjoint
함수는 행렬의 adjoint를 찾는 데 사용됩니다. adjoint 행렬을 찾은 후 행렬식의 역수를 곱하여 역행렬을 찾습니다.
역함수
void inverse(float matrix3X3[][3], float d) {
cout << "\n\nInverse of matrix is: \n";
for (int r = 0; r < 3; r++) {
for (int c = 0; c < 3; c++) {
cout << ((matrix3X3[(c + 1) % 3][(r + 1) % 3] *
matrix3X3[(c + 2) % 3][(r + 2) % 3]) -
(matrix3X3[(c + 1) % 3][(r + 2) % 3] *
matrix3X3[(c + 2) % 3][(r + 1) % 3])) /
d
<< "\t";
}
cout << endl;
}
}
마지막으로 inverse 함수는 행렬의 역함수를 계산하는 데 사용됩니다. 이 함수에서 adjoint 행렬의 각 항목은 역행렬을 찾기 위해 행렬식으로 나뉩니다.
이제 3x3 행렬의 역행렬에 대한 전체 코드를 살펴보겠습니다.
전체 C++ 프로그램
#include <iostream>
using namespace std;
void display(float matrix3X3[][3]) {
printf("\nThe Elements of the matrix are :");
for (int r = 0; r < 3; r++) {
cout << "\n";
for (int c = 0; c < 3; c++) {
cout << matrix3X3[r][c] << "\t";
}
}
}
// This function will calculate the determinant of the given matrix
float findDeterminant(float matrix3X3[][3]) {
float det = 0; // here det is the determinant of the matrix.
for (int r = 0; r < 3; r++) {
det = det + (matrix3X3[0][r] *
(matrix3X3[1][(r + 1) % 3] * matrix3X3[2][(r + 2) % 3] -
matrix3X3[1][(r + 2) % 3] * matrix3X3[2][(r + 1) % 3]));
}
return det;
}
// This function will calculate the adjoint of the given matrix
void adjoint(float matrix3X3[][3]) {
cout << "\n\nAdjoint of matrix is: \n";
for (int r = 0; r < 3; r++) {
for (int c = 0; c < 3; c++) {
cout << ((matrix3X3[(c + 1) % 3][(r + 1) % 3] *
matrix3X3[(c + 2) % 3][(r + 2) % 3]) -
(matrix3X3[(c + 1) % 3][(r + 2) % 3] *
matrix3X3[(c + 2) % 3][(r + 1) % 3]))
<< "\t";
}
cout << endl;
}
}
// This function will find the Inverse of the given matrix
void inverse(float matrix3X3[][3], float d) {
cout << "\n\nInverse of matrix is: \n";
for (int r = 0; r < 3; r++) {
for (int c = 0; c < 3; c++) {
cout << ((matrix3X3[(c + 1) % 3][(r + 1) % 3] *
matrix3X3[(c + 2) % 3][(r + 2) % 3]) -
(matrix3X3[(c + 1) % 3][(r + 2) % 3] *
matrix3X3[(c + 2) % 3][(r + 1) % 3])) /
d
<< "\t";
}
cout << endl;
}
}
int main() // main function
{
float matrix3X3[3][3];
int r, c;
float determinant = 0;
for (r = 0; r < 3; r++) {
for (c = 0; c < 3; c++) {
cout << "Enter the value at index [" << r << "][" << c << "] : ";
cin >> matrix3X3[r][c];
}
}
display(matrix3X3);
determinant = findDeterminant(matrix3X3);
cout << "\n\nDeteterminant of the given matrix is : " << determinant;
if (determinant != 0) {
adjoint(matrix3X3);
inverse(matrix3X3, determinant);
} else {
cout << " As determinant of the given matrix is 0, so we cannot take find "
"it's Inverse :";
}
}
입력 행렬이 특이 행렬일 때 출력:
출력 결과는 행렬이 특이 행렬일 때 알고리즘이 역행렬을 계산하지 않는다는 것을 보여줍니다.
입력 행렬이 특이 행렬이 아닌 경우 출력:
결과는 행렬이 비특이인 경우에만 알고리즘이 역함수를 계산함을 보여줍니다.