C++에서 개체 유형 찾기
- C++에서 클래스 개체 유형 찾기
-
typeid().name()
을 사용하여 C++에서 클래스 객체 유형 찾기 -
typeid.().name()
을__cxa_demangle
과 함께 사용하여 C++에서 클래스 객체 유형 찾기
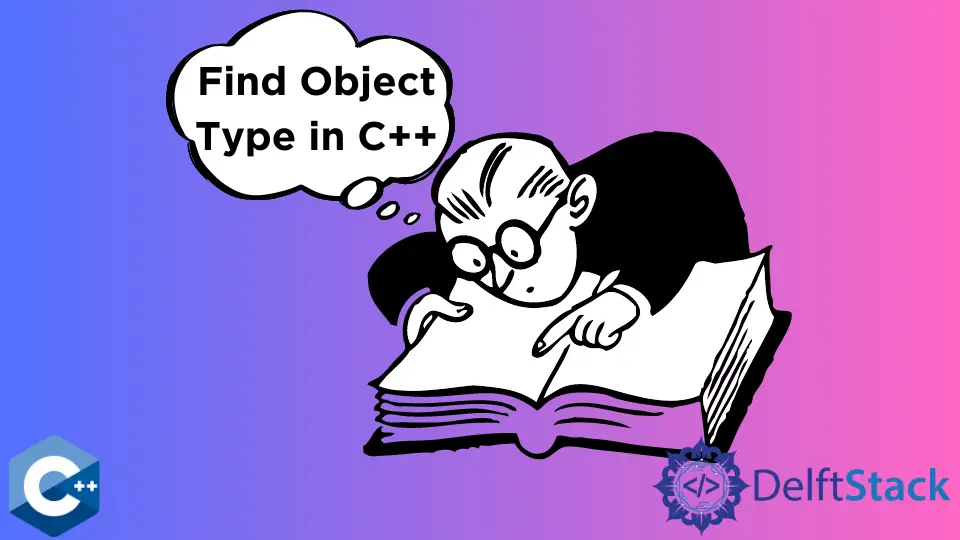
이 문서에서는 C++에서 개체 형식을 찾는 다양한 방법에 대해 설명합니다.
C++에서 클래스 개체 유형 찾기
간단한 변수에 int, bool 등과 같은 데이터 유형이 있는 것과 유사하게 클래스 객체에는 자신이 속한 클래스인 유형이 있습니다.
#include <iostream>
using namespace std;
class Vehicles {
public:
string make;
string model;
string year;
};
int main() { Vehicles car; }
위의 예에서 main()
에 정의된 car
객체 유형은 Vehicles
클래스이며 car
는 Vehicles
의 객체입니다.
위의 예는 매우 간단하며 개체 유형을 쉽게 식별할 수 있습니다. 그러나 더 복잡한 프로젝트, 특히 여러 클래스와 개체 및 여러 프로그래머가 함께 작업하는 경우 개체 유형을 추적하는 것은 매우 혼란스러울 수 있습니다.
이 문제를 방지하기 위해 객체의 유형을 결정하는 몇 가지 방법을 사용할 수 있으며 아래 예제를 통해 이에 대해 살펴보겠습니다.
typeid().name()
을 사용하여 C++에서 클래스 객체 유형 찾기
typeid()
는 typeinfo
라이브러리에 포함된 메서드입니다. typeid.().name()
함수는 변수 또는 클래스 객체를 가져와 해당 유형의 이름을 문자열로 반환합니다.
typeid.().name()
함수의 사용은 다음과 같습니다.
#include <iostream>
#include <typeinfo>
using namespace std;
class Vehicles {
public:
string make;
string model;
string year;
};
class Aircraft {
public:
string make;
string model;
string year;
};
int main() {
int x;
float y;
bool b;
Vehicles car;
Aircraft helicopter;
cout << "The type of the variable x "
<< " is " << typeid(x).name() << endl;
cout << "The type of the variable y "
<< " is " << typeid(y).name() << endl;
cout << "The type of the variable b "
<< " is " << typeid(b).name() << endl;
cout << "The type of the object car "
<< " is " << typeid(car).name() << endl;
cout << "The type of the object helicopter "
<< " is " << typeid(helicopter).name() << endl;
}
위의 코드 스니펫은 다음 출력을 생성합니다.
The type of the variable x is i
The type of the variable y is f
The type of the variable b is b
The type of the object car is 8Vehicles
The type of the object helicopter is 8Aircraft
위에서 본 것처럼 typeid.().name()
함수는 단순 변수와 사용자 정의 클래스 객체 모두에 대해 작동합니다. 이 함수는 각각 int, float 및 bool에 대해 i
, f
및 b
를 반환합니다.
이 함수는 car
및 helicopter
개체에 대한 해당 클래스의 이름을 반환합니다. 클래스 및 변수 이름이 C++ 컨텍스트에 저장되는 방식 때문에 이 예제에서 출력 클래스 이름 앞에 숫자 8
이 있다는 점에 유의하는 것이 중요합니다.
typeid.().name()
함수는 클래스 목표와 함께 사용될 때 시스템 및 컴파일러에 따라 다르며 반환할 때 클래스 이름 앞에 임의의 문자를 추가할 수 있습니다. 사용 중인 시스템에서 컴파일러는 클래스 이름 앞에 클래스 이름의 문자 수를 추가합니다. 즉, Vehicle
과 Aircraft
모두 이름에 8개의 문자가 있습니다.
typeid.().name()
은 구현에 따라 다르므로 c++filt
명령 또는 __cxa_demangle
을 사용하여 차례로 디글글링할 수 있는 맹글링된 클래스 이름을 반환합니다.
엉망이 된 형태로 반환되는 클래스 이름에도 불구하고 여전히 읽을 수 있으며 개체의 클래스를 식별하는 데 사용할 수 있습니다. 그리고 리턴 타입이 문자열이기 때문에 같은 클래스인지 확인하기 위해 객체를 쉽게 비교할 수 있습니다.
typeid.().name()
을 __cxa_demangle
과 함께 사용하여 C++에서 클래스 객체 유형 찾기
이전 예제에서 볼 수 있듯이 typeid.().name()
을 사용하면 변환된 클래스 이름과 변수 유형이 반환됩니다. demangled 결과를 얻기 위해 cxxabi.h
라이브러리에서 __cxa_demangle
함수를 사용할 수 있습니다.
__cxa_demangle
의 구문은 다음과 같습니다.
char *abi::__cxa_demangle(const char *mangled_name, char *output_buffer, size_t *length, int *status)
typeid.().name()
과 __cxa_demangle
의 사용은 아래 예에 나와 있습니다.
#include <cxxabi.h>
#include <iostream>
using namespace std;
class Vehicles {
public:
string make;
string model;
string year;
};
class Aircraft {
public:
string make;
string model;
string year;
};
int main() {
int x;
float y;
bool b;
Vehicles car;
Aircraft helicopter;
cout << "The type of the variable x "
<< " is "
<< abi::__cxa_demangle(typeid(x).name(), nullptr, nullptr, nullptr)
<< endl;
cout << "The type of the variable y "
<< " is "
<< abi::__cxa_demangle(typeid(y).name(), nullptr, nullptr, nullptr)
<< endl;
cout << "The type of the variable b "
<< " is "
<< abi::__cxa_demangle(typeid(b).name(), nullptr, nullptr, nullptr)
<< endl;
cout << "The type of the object car "
<< " is "
<< abi::__cxa_demangle(typeid(car).name(), nullptr, nullptr, nullptr)
<< endl;
cout << "The type of the object helicopter "
<< " is "
<< abi::__cxa_demangle(typeid(helicopter).name(), nullptr, nullptr,
nullptr)
<< endl;
}
이 예제의 범위에서 우리는 __cxa_demangle
함수의 첫 번째 인수, 즉 맹글링된 이름에만 관심이 있었습니다. 이 경우 나머지 인수는 필요하지 않았습니다.
위의 코드는 다음과 같은 출력을 생성합니다.
The type of the variable x is int
The type of the variable y is float
The type of the variable b is bool
The type of the object car is Vehicles
The type of the object helicopter is Aircraft
출력에서 볼 수 있듯이 반환된 변수 및 형식 이름은 디글글링되어 코드 파일에서 정확히 어떻게 나타납니다.