Converti JSON in un DataFrame Pandas
-
Da JSON a Pandas DataFrame utilizzando
json_normalize()
-
Da JSON a Pandas DataFrame utilizzando
read_json()
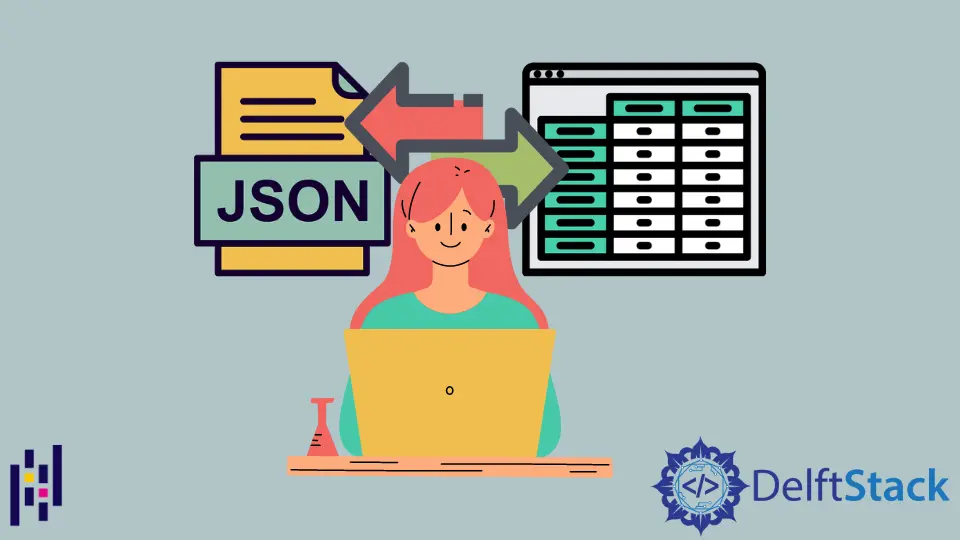
Questo articolo introdurrà come convertire JSON in un DataFrame Pandas.
JSON sta per JavaScript Object Notation. Si basa sul formato degli oggetti in JavaScript ed è una tecnica di codifica per rappresentare i dati strutturati. È ampiamente utilizzato in questi giorni, soprattutto per la condivisione di dati tra server e applicazioni web.
Grazie alla sua semplicità e influenza dalle strutture dati del linguaggio di programmazione, JSON sta diventando immensamente popolare. È relativamente facile da capire e il seguente è un semplice esempio di una risposta JSON da un’API.
{
"Results":
[
{ "id": "01", "Name": "Jay" },
{ "id": "02", "Name": "Mark" },
{ "id": "03", "Name": "Jack" }
],
"status": ["ok"]
}
Come puoi vedere nel nostro esempio, JSON sembra essere in qualche modo una combinazione di liste e dizionari annidati; pertanto, è relativamente facile estrarre dati da file JSON e persino archiviarli come Pandas DataFrame.
Le librerie Pandas e JSON in Python possono aiutare a raggiungere questo obiettivo. Abbiamo due funzioni read_json()
e json_normalize()
che possono aiutare a convertire la stringa JSON in un DataFrame.
Da JSON a Pandas DataFrame utilizzando json_normalize()
La funzione json_normalize()
è molto utilizzata per leggere la stringa JSON nidificata e restituire un DataFrame. Per utilizzare questa funzione, dobbiamo prima leggere la stringa JSON utilizzando la funzione json.loads()
nella libreria JSON in Python. Quindi passiamo questo oggetto JSON a json_normalize()
, che restituirà un Pandas DataFrame contenente i dati richiesti.
import pandas as pd
import json
from pandas import json_normalize
data = """
{
"Results":
[
{ "id": "1", "Name": "Jay" },
{ "id": "2", "Name": "Mark" },
{ "id": "3", "Name": "Jack" }
],
"status": ["ok"]
}
"""
info = json.loads(data)
df = json_normalize(info["Results"]) # Results contain the required data
print(df)
Produzione:
id Name
0 1 Jay
1 2 Mark
2 3 Jack
Da JSON a Pandas DataFrame utilizzando read_json()
Un’altra funzione di Pandas per convertire JSON in un DataFrame è read_json()
per stringhe JSON più semplici. Possiamo passare direttamente il percorso di un file JSON o la stringa JSON alla funzione per l’archiviazione dei dati in un Pandas DataFrame. read_json()
ha molti parametri, tra cui orient
specifica il formato della stringa JSON.
Lo svantaggio è che è difficile da usare con stringhe JSON nidificate. Quindi, per usare read_json()
, useremo un esempio molto più semplice come mostrato di seguito:
import pandas as pd
data = """
{
"0":{
"Name": "Jay",
"Age": "17"
},
"1":{
"Name": "Mark",
"Age": "15"
},
"2":{
"Name": "Jack",
"Age":"16"
}
}
"""
df = pd.read_json(data, orient="index")
print(df)
Produzione:
Name Age
0 Jay 17
1 Mark 15
2 Jack 16
Impostiamo orient
come 'index'
perché la stringa JSON fromat corrisponde al pattern come {index : {column: value}}
.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInArticolo correlato - Pandas DataFrame
- Come ottenere le intestazioni delle colonne DataFrame Pandas come lista
- Come cancellare la colonna DataFrame Pandas DataFrame
- Come convertire la colonna DataFrame in data e ora in pandas
- Converti un Float in un Integer in Pandas DataFrame
- Ordina Pandas DataFrame in base ai valori di una colonna
- Ottieni l'aggregato di Pandas Group-By e Sum