Ottieni l'ID thread in Java
-
Ottieni ID thread utilizzando
Thread.getId()
in Java -
Ottieni l’ID del pool di thread corrente utilizzando
Thread.currentThread().getId()
in Java
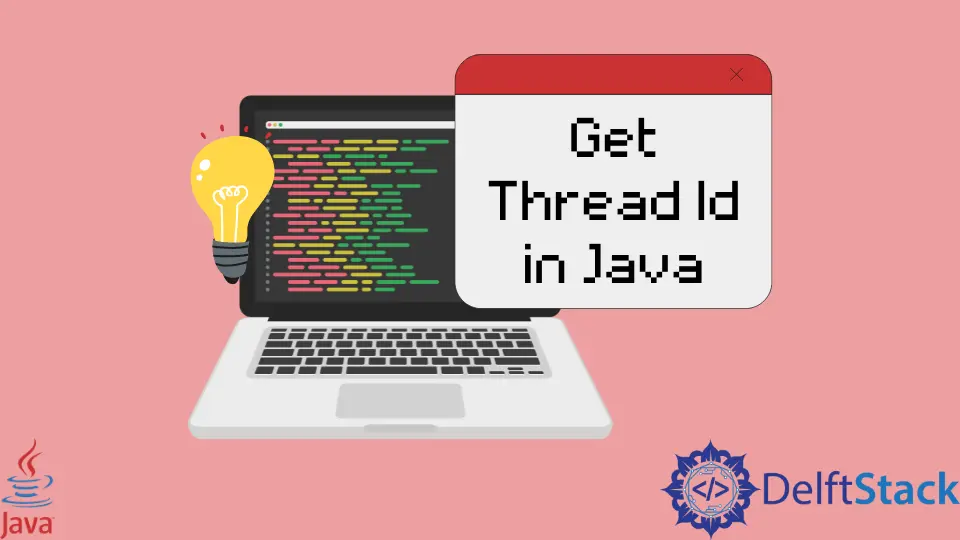
In questo tutorial, introdurremo metodi per ottenere l’ID del thread in Java. Vedremo anche come possiamo ottenere l’id del thread corrente da un pool di thread.
Ottieni ID thread utilizzando Thread.getId()
in Java
In questo esempio, abbiamo creato una classe Task
che implementa la classe Runnable
perché abbiamo bisogno del suo metodo run()
per eseguire il thread. La classe Task
prende il nome di un thread dal suo costruttore e il metodo run()
lo stampa sulla console quando viene eseguito.
Nel metodo main()
, creiamo due oggetti Task
nel costruttore e poi due oggetti thread in cui passiamo task1
e task2
per assegnare i compiti.
Chiameremo il metodo start()
usando thread1
e thread2
per eseguire i thread. Alla fine, una volta che i thread sono stati eseguiti, possiamo ottenere l’id di ogni thread usando thread.getId()
, che restituisce l’id come un long
.
public class GetThreadID {
public static void main(String[] args) {
Task task1 = new Task("Task 1");
Task task2 = new Task("Task 2");
Thread thread1 = new Thread(task1);
Thread thread2 = new Thread(task2);
thread1.start();
thread2.start();
System.out.println("Thread1's ID is: " + thread1.getId());
System.out.println("Thread2's ID is: " + thread2.getId());
}
}
class Task implements Runnable {
private String name;
Task(String name) {
this.name = name;
}
@Override
public void run() {
System.out.println("Executing " + name);
}
}
Produzione:
Thread1's ID is: 13
Thread2's ID is: 14
Executing Task 2
Executing Task 1
Ottieni l’ID del pool di thread corrente utilizzando Thread.currentThread().getId()
in Java
I pool di thread sono utili quando si tratta di eseguire attività pesanti. Nell’esempio seguente, creiamo un pool di thread utilizzando Executors.newFixedThreadPool(numberOfThreads)
. Possiamo specificare il numero di thread che vogliamo nel pool.
La classe Task
è responsabile dell’esecuzione del thread nel metodo run()
. È una semplice classe che imposta e ottiene il nome del thread passato nel costruttore. Per creare più attività, usiamo un cicli for
in cui vengono creati cinque oggetti Task
e cinque thread vengono eseguiti nel pool.
Il nostro obiettivo è ottenere l’id di ogni thread attualmente in esecuzione. Per farlo, useremo Thread.currentThread().getId()
che restituisce l’id del thread corrente. Nell’output, possiamo vedere gli ID di tutti i thread che eseguono le singole attività.
Una volta completate le attività, dovremmo interrompere l’esecuzione del pool di thread utilizzando threadExecutor.shutdown()
. !threadExecutor.isTerminated()
è usato per aspettare che il threadExecutor
sia terminato.
package com.company;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class GetThreadID {
public static void main(String[] args) {
int numberOfThreads = 5;
ExecutorService threadExecutor = Executors.newFixedThreadPool(numberOfThreads);
for (int i = 0; i < 5; i++) {
Task task = new Task("Task " + i);
System.out.println("Created Task: " + task.getName());
threadExecutor.execute(task);
}
threadExecutor.shutdown();
while (!threadExecutor.isTerminated()) {
}
System.out.println("All threads have completed their tasks");
}
}
class Task implements Runnable {
private String name;
Task(String name) {
this.name = name;
}
public String getName() {
return name;
}
@Override
public void run() {
System.out.println("Executing: " + name);
System.out.println(name + " is on thread id #" + Thread.currentThread().getId());
}
}
Produzione:
Created Task: Task 0
Created Task: Task 1
Created Task: Task 2
Created Task: Task 3
Created Task: Task 4
Executing: Task 0
Executing: Task 2
Executing: Task 1
Executing: Task 4
Executing: Task 3
Task 0 is on thread id #13
Task 1 is on thread id #14
Task 4 is on thread id #17
Task 2 is on thread id #15
Task 3 is on thread id #16
All threads have completed their tasks
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn