Programma istogramma in Java
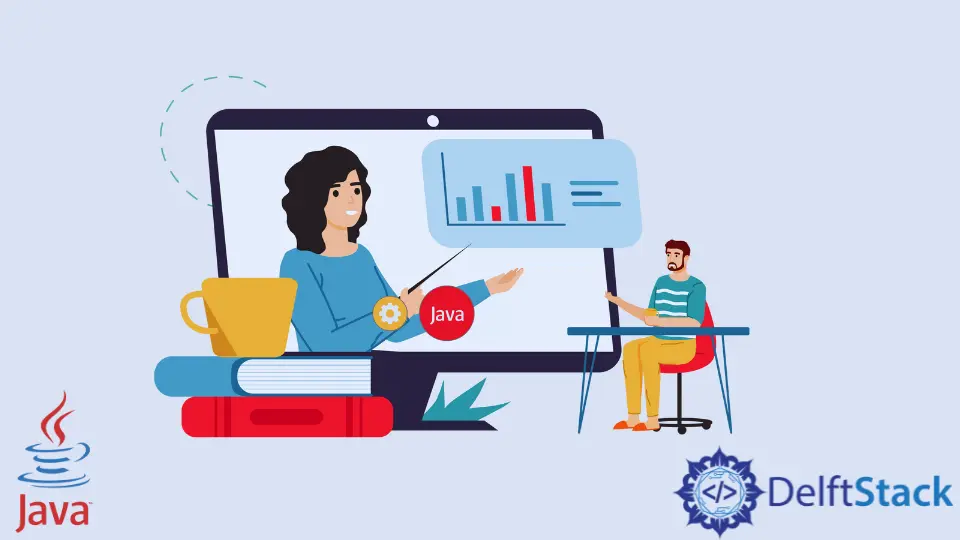
Gli istogrammi ci consentono di mantenere il conteggio di diverse categorie di valori. Possiamo anche rappresentarli graficamente.
In questo articolo utilizzeremo Java per creare un istogramma che memorizzerà la somma dei dadi lanciati.
Per verificare i valori, utilizzeremo la scala if-else
. La scala if-else
è un modo efficiente di confrontare un elemento con più valori. Manteniamo il conteggio di ogni somma in diverse variabili intere. Ogni variabile viene incrementata quando si verifica la corrispondenza.
Chiediamo all’utente l’input della dimensione dell’array dell’istogramma. Questa matrice rappresenterà il numero totale di volte in cui ogni dado verrà lanciato. Inizializzeremo quindi i numeri che risulteranno come somma di due dadi lanciati contemporaneamente, partendo da 2 a 12.
L’utente inserisce il valore per entrambi i dadi e noi calcoleremo la loro somma. La scala if-else
confronterà la somma con diverse possibilità e aumenterà ogni volta che si verifica una corrispondenza.
Attueremo tutto questo nel seguente programma.
import java.io.*;
class Main {
public static String toStars(int number) {
StringBuilder temp = new StringBuilder();
for (int i = 0; i < number; i++) {
temp.append("*");
}
return temp.toString();
}
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
System.out.print("Total rolls for each dice?");
int n = Integer.parseInt(br.readLine());
int[] rolls = new int[n];
int d1;
int d2;
int two = 0;
int three = 0;
int four = 0;
int five = 0;
int six = 0;
int seven = 0;
int eight = 0;
int nine = 0;
int ten = 0;
int eleven = 0;
int twelve = 0;
for (int roll = 0; roll < rolls.length; roll++) {
System.out.println("First dice roll");
d1 = Integer.parseInt(br.readLine());
System.out.println("Second dice roll");
d2 = Integer.parseInt(br.readLine());
System.out.println(" The first dice rolled a " + d1 + " the second dice rolled a " + d2);
int sum;
sum = d1 + d2;
if (sum == 2)
two++;
if (sum == 3)
three++;
if (sum == 4)
four++;
if (sum == 5)
five++;
if (sum == 6)
six++;
if (sum == 7)
seven++;
if (sum == 8)
eight++;
if (sum == 9)
nine++;
if (sum == 10)
ten++;
if (sum == 11)
eleven++;
if (sum == 12)
twelve++;
}
System.out.println("Histogram of rolls:");
System.out.println("2 occurred " + toStars(two) + " times");
System.out.println("3 occurred " + toStars(three) + " times");
System.out.println("4 occurred " + toStars(four) + " times");
System.out.println("5 occurred " + toStars(five) + " times");
System.out.println("6 occurred " + toStars(six) + " times");
System.out.println("7 occurred " + toStars(seven) + " times");
System.out.println("8 occurred " + toStars(eight) + " times");
System.out.println("9 occurred " + toStars(nine) + " times");
System.out.println("10 occurred " + toStars(ten) + " times");
System.out.println("11 occurred " + toStars(eleven) + " times");
System.out.println("12 occurred " + toStars(twelve) + " times");
}
}
Produzione:
Total rolls for each dice?5
First dice roll
1
Second dice roll
2
The first dice rolled a 1 the second dice rolled a 2
First dice roll
2
Second dice roll
1
The first dice rolled a 2 the second dice rolled a 1
First dice roll
5
Second dice roll
4
The first dice rolled a 5 the second dice rolled a 4
First dice roll
1
Second dice roll
1
The first dice rolled a 1 the second dice rolled a 1
First dice roll
3
Second dice roll
1
The first dice rolled a 3 the second dice rolled a 1
Histogram of rolls:
2 occurred * times
3 occurred ** times
4 occurred * times
5 occurred times
6 occurred times
7 occurred times
8 occurred times
9 occurred * times
10 occurred times
11 occurred times
12 occurred times
Nota che per visualizzare l’output finale, creiamo una funzione separata toStars()
che converte la frequenza per ogni possibilità nel numero di stelle. Questo metodo è visivamente accattivante e fornisce una buona rappresentazione di un istogramma.