Supprimer un caractère d'une chaîne de caractères en JavaScript
-
Utiliser la méthode
replace()
avec expression régulière en JavaScript - Supprimer un caractère spécifié à l’index donné en JavaScript
- Supprimer la première instance d’un caractère dans une chaîne de caractères en JavaScript
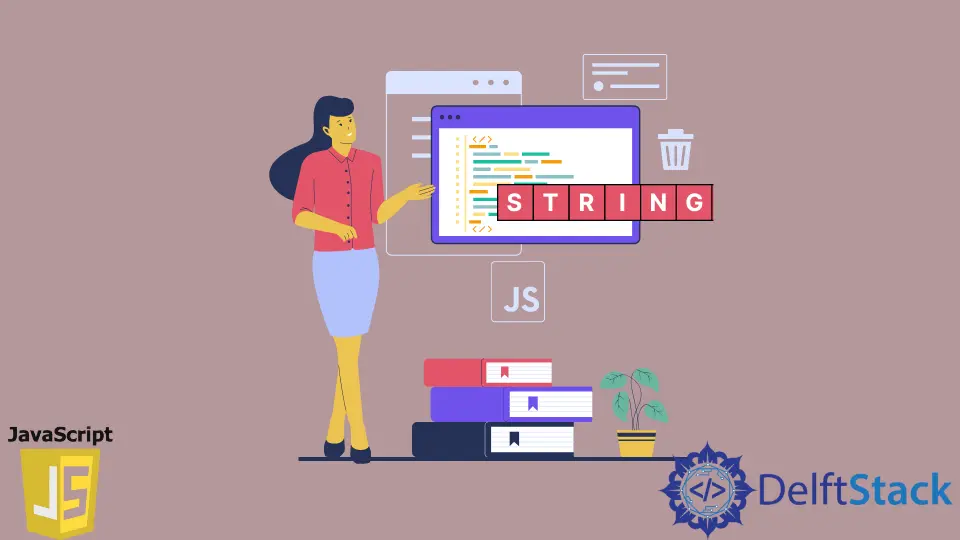
JavaScript dispose de différentes méthodes pour supprimer un caractère spécifique d’une chaîne de caractères. Nous allons présenter la manière de supprimer un caractère d’une chaîne de caractères en JavaScript.
Utiliser la méthode replace()
avec expression régulière en JavaScript
Nous utilisons la méthode replace()
avec l’expression régulière pour supprimer toutes les instances du caractère spécifié dans une chaîne en JavaScript.
JavaScript replace()
Syntaxe de l’expression régulière
replace(/regExp/g, '');
Exemple :
<!DOCTYPE html>
<html>
<head>
<title>
How to remove all instances of the specified character in a string?
</title>
</head>
<body>
<h1>
DelftStack
</h1>
<b>
How to remove all instances of the specified character in a string?
</b>
<p>The original string is DelftStack</p>
<p>
New Output is:
<span id="outputWord"></span>
</p>
<button onclick="removeCharacterFromString()">
Remove Character
</button>
<script type="text/javascript">
const removeCharacterFromString = () => {
originalWord = 'DelftStack';
newWord = originalWord.replace(/t/g, '');
document.querySelector('#outputWord').textContent
= newWord;
}
</script>
</body>
</html>
Production :
The original string is DelftStack
New Output is: DelfSack
Supprimer un caractère spécifié à l’index donné en JavaScript
Lorsque nous devons supprimer un caractère lorsque nous avons plus d’une instance de ce caractère dans une chaîne, par exemple, supprimer le caractère t
d’une chaîne DelftStack
, nous pouvons utiliser la méthode slice()
pour obtenir deux chaînes de caractères avant et après l’index donné et les concaténer.
Exemple :
<!DOCTYPE html>
<html>
<head>
<title>
How to remove Specified Character at a Given Index in a string?
</title>
</head>
<body>
<h1>
DelftStack
</h1>
<b>
How to remove Specified Character at a Given Index in a string?
</b>
<p>The original string is DelftStack</p>
<p>
New Output is:
<span id="outputWord"></span>
</p>
<button onclick="removeCharacterFromString(5)">
Remove Character
</button>
<script type="text/javascript">
const removeCharacterFromString = (position) => {
originalWord = 'DelftStack';
newWord = originalWord.slice(0, position - 1)
+ originalWord.slice(position, originalWord.length);
document.querySelector('#outputWord').textContent
= newWord;
}
</script>
</body>
</html>
Supprimer la première instance d’un caractère dans une chaîne de caractères en JavaScript
Nous pouvons utiliser la méthode replace()
sans expression régulière pour supprimer uniquement la première instance d’un caractère d’une chaîne de caractères en JavaScript. Nous passons le caractère à supprimer comme premier argument et la chaîne vide ''
comme deuxième argument.
Exemple :
<!DOCTYPE html>
<html>
<head>
<title>
How to remove First Instance of Character in a string?
</title>
</head>
<body>
<h1>
DelftStack
</h1>
<b>
How to remove First Instance of Character in a string?
</b>
<p>The original string is DelftStack</p>
<p>
New Output is:
<span id="outputWord"></span>
</p>
<button onclick="removeCharacterFromString()">
Remove Character
</button>
<script type="text/javascript">
const removeCharacterFromString = () => {
originalWord = 'DelftStack';
newWord = originalWord.replace('t', '');
document.querySelector('#outputWord').textContent
= newWord;
}
</script>
</body>
</html>
Article connexe - JavaScript String
- Obtenir le dernier caractère d'une chaîne en JavaScript
- Transformer une chaîne en une date en JavaScript
- Obtenir le premier caractère d'une chaîne en JavaScript
- Convertir un tableau en chaîne en JavaScript
- Vérifier l'égalité des chaînes en JavaScript
- Convertir un tableau en chaîne sans virgule en JavaScript