Générer une chaîne aléatoire de longueur fixe dans Golang
Jay Singh
26 aout 2022
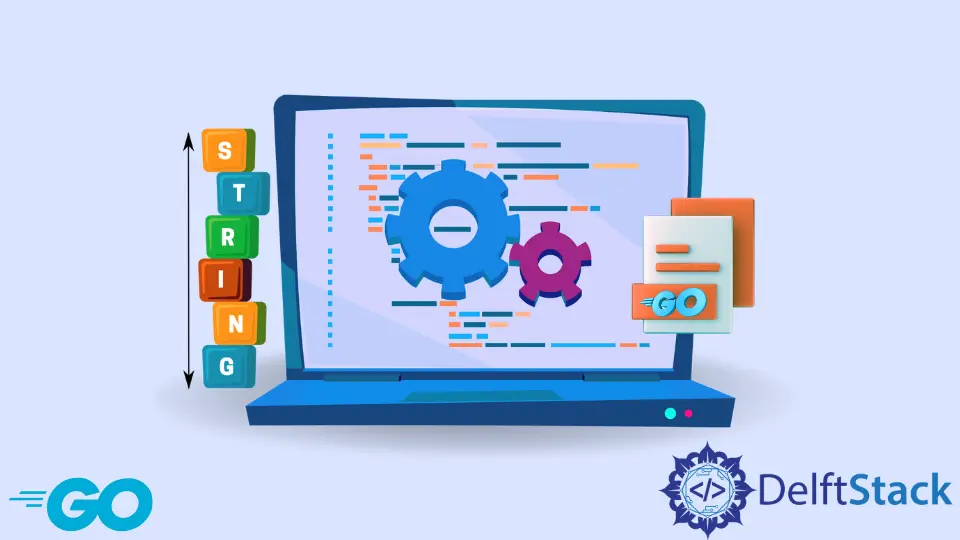
De nombreux algorithmes différents nécessitent la production de chaînes aléatoires d’une longueur spécifique. Cet article vous montrera comment produire une chaîne aléatoire d’une longueur fixe dans Golang selon plusieurs méthodes différentes.
Utilisez math/rand
pour générer une chaîne aléatoire de longueur fixe dans Golang
Le package math/rand
de Go permet la génération de nombres pseudo-aléatoires. Nous allons utiliser le package dans cet exemple et transmettre une chaîne aléatoire pour recevoir la sortie d’une chaîne de longueur fixe.
package main
import (
"fmt"
"math/rand"
)
func main() {
fmt.Println(RandomString(30))
}
func RandomString(n int) string {
var letterRunes = []rune("abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ")
b := make([]rune, n)
for i := range b {
b[i] = letterRunes[rand.Intn(len(letterRunes))]
}
return string(b)
}
Production:
XVlBzgbaiCMRAjWwhTHctcuAxhxKQF
Pour un autre exemple, la méthode accepte une chaîne contenant chaque lettre et produit une chaîne en en choisissant une lettre au hasard.
package main
import (
"fmt"
"math/rand"
)
func RandomString(n int) string {
var letters = []rune("abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789")
s := make([]rune, n)
for i := range s {
s[i] = letters[rand.Intn(len(letters))]
}
return string(s)
}
func main() {
fmt.Println(RandomString(13))
}
Production:
BpLnfgDsc2WD8
Prenons un dernier exemple pour bien comprendre comment générer une chaîne de longueur fixe dans Golang.
package main
import (
"fmt"
"math/rand"
)
func main() {
fmt.Println("Random String")
fmt.Println()
fmt.Println("11 chars: " + RandomString(11))
fmt.Println("20 chars: " + RandomString(20))
fmt.Println("32 chars: " + RandomString(32))
}
func RandomString(n int) string {
var letter = []rune("abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789")
b := make([]rune, n)
for i := range b {
b[i] = letter[rand.Intn(len(letter))]
}
return string(b)
}
Production:
Random String
11 chars: BpLnfgDsc2W
20 chars: D8F2qNfHK5a84jjJkwzD
32 chars: kh9h2fhfUVuS9jZ8uVbhV3vC5AWX39IV
Article connexe - Go String
- Comment analyser la chaîne de dates dans Go
- Comment concaténer efficacement des chaînes dans Go
- Comment convertir une chaîne de caractères en un nombre entier en Go
- Comment convertir une valeur int en chaîne dans Go
- Comment écrire des chaînes de caractères multilignes dans Go
- Convertir une chaîne en tableau d'octets dans Golang