Calcul du pourcentage en C#
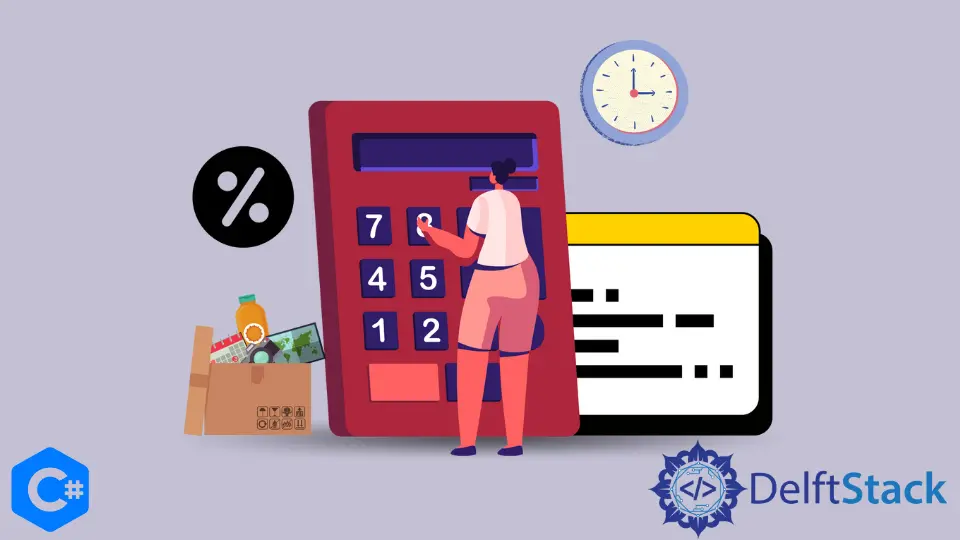
Cet article montre comment calculer des pourcentages dans le langage de programmation C#.
Créer un programme qui calculera le pourcentage à l’aide de C#
Pour commencer, nous devons importer les bibliothèques suivantes.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
Construisez ensuite quatre variables de type double
nommées sub1
, sub2
, sub3
et sub4
. Il y aura également une variable appelée total
qui stockera les notes totales pour toutes les matières.
Créez également une variable pourcentage de type double
nommée per
.
double sub1, sub2, sub3, sub4, total;
double per;
Nous pouvons maintenant calculer le pourcentage en utilisant la formule fournie.
per = total / 4; // Here "total" holds the total marks of all subjects, and "4" is the total number
// of subjects.
Code source complet :
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
public class PercentageCalculation {
static void Main(string[] args) {
double sub1, sub2, sub3, sub4, total;
double per;
Console.Write("Enter marks of subjects to find out the percentage. \n");
Console.Write("Input the marks of Subject1: ");
sub1 = Convert.ToInt32(Console.ReadLine());
Console.Write("Input the marks of Subject2: ");
sub2 = Convert.ToInt32(Console.ReadLine());
Console.Write("Input the marks of Subject3: ");
sub3 = Convert.ToInt32(Console.ReadLine());
Console.Write("Input the marks of Subject4: ");
sub4 = Convert.ToInt32(Console.ReadLine());
total = sub1 + sub2 + sub3 + sub4;
per = total / 4;
Console.Write("Percentage = {0}%\n", per);
}
}
Production:
Enter marks of subjects to find out the percentage.Input the marks of Subject1 : 34 Input the marks
of Subject2 : 45 Input the marks of Subject3 : 56 Input the marks of Subject4 : 45 Percentage =
45 %
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn